Text
Hello everyone, I am Python artificial intelligence technology.
In this article, we will discuss the most commonly used python tricks. Most of these techniques are simple Tricks that I use in my daily work, and I think good things should be shared with everyone.
Without further ado, let’s get started! :)
Tips summary
1. Handling multiple inputs from the user
Sometimes we need to get multiple inputs from the user in order to use a loop or any iteration, generally The writing method is as follows:
# bad practice码 n1 = input("enter a number : ") n2 = input("enter a number : ") n2 = input("enter a number : ") print(n1, n2, n3)
But a better processing method is as follows:
# good practice n1, n2, n3 = input("enter a number : ").split() print(n1, n2, n3)
2. Processing multiple conditional statements
If we need to check multiple conditional statements in the code, At this point we can use the all() or any() function to achieve our goal. Generally, all() is used when we have multiple and conditions and any() is used when we have multiple or conditions. This usage will make our code clearer and easier to read, and will help us avoid trouble when debugging.
The general example for all() is as follows:
size = "lg" color = "blue" price = 50 # bad practice if size == "lg" and color == "blue" and price < 100: print("Yes, I want to but the product.")
A better processing method is as follows:
# good practice conditions = [ size == "lg", color == "blue", price < 100, ] if all(conditions): print("Yes, I want to but the product.")
The general example for any() is as follows:
# bad practice size = "lg" color = "blue" price = 50 if size == "lg" or color == "blue" or price < 100: print("Yes, I want to but the product.")
A better way to handle it is as follows:
# good practice conditions = [ size == "lg", color == "blue", price < 100, ] if any(conditions): print("Yes, I want to but the product.")
3. Determine number parity
This is easy to implement, we get the input from the user, convert it to an integer, check for the number 2 The remainder operation of , if the remainder is zero, it is an even number.
print('odd' if int(input('Enter a number: '))%2 else 'even')
4. Exchange variables
If we need to exchange the value of a variable in Python, we do not need to define a temporary variable to operate. We generally use the following code to implement variable exchange:
v1 = 100 v2 = 200 # bad practice temp = v1 v1 = v2 v2 = temp
But a better processing method is as follows:
v1 = 100 v2 = 200 # good practice v1, v2 = v2, v1
5. Determine whether the string is a palindrome string
Change the characters The simplest way to reverse a string is [::-1], the code is as follows:
print("John Deo"[::-1])
6. Reverse a string
Determine whether a string is a palindrome in Python String, just use the statement
string.find(string[::-1])== 0, the sample code is as follows:
v1 = "madam" # is a palindrome string v2 = "master" # is not a palindrome string print(v1.find(v1[::-1]) == 0) # True print(v1.find(v2[::-1]) == 0) # False
7. Try to use Inline if statement
Most of the time, we only have one statement after the condition, so using Inline if statement can help us write more concise code. For example, the general writing method is:
name = "ali" age = 22 # bad practices if name: print(name) if name and age > 18: print("user is verified")
But a better processing method is as follows:
# a better approach print(name if name else "") """ here you have to define the else condition too""" # good practice name and print(name) age > 18 and name and print("user is verified")
8. Delete duplicate elements in the list
We don’t need to traverse the entire list List to check for duplicate elements, we can simply use set() to delete duplicate elements, the code is as follows:
lst = [1, 2, 3, 4, 3, 4, 4, 5, 6, 3, 1, 6, 7, 9, 4, 0] print(lst) unique_lst = list(set(lst)) print(unique_lst)
9. Find the most repeated elements in the list
You can use max in Python ( ) function and pass list.count as key, you can find the element with the most repetitions in the list. The code is as follows:
lst = [1, 2, 3, 4, 3, 4, 4, 5, 6, 3, 1, 6, 7, 9, 4, 0] most_repeated_item = max(lst, key=lst.count) print(most_repeated_item)
10. List generation
My favorite in Python The function of List Comprehension is list comprehensions. This feature allows us to write very concise and powerful code, and these codes read almost as easy to understand as natural language. An example is as follows:
numbers = [1,2,3,4,5,6,7] evens = [x for x in numbers if x % 2 is 0] odds = [y for y in numbers if y not in evens] cities = ['London', 'Dublin', 'Oslo'] def visit(city): print("Welcome to "+city) for city in cities: visit(city)
11. Use *args to pass multiple parameters
In Python, we can use *args to pass multiple parameters to a function. An example is as follows:
def sum_of_squares(n1, n2) return n1**2 + n2**2 print(sum_of_squares(2,3)) # output: 13 """ what ever if you want to pass, multiple args to the function as n number of args. so let's make it dynamic. """ def sum_of_squares(*args): return sum([item**2 for item in args]) # now you can pass as many parameters as you want print(sum_of_squares(2, 3, 4)) print(sum_of_squares(2, 3, 4, 5, 6))
12. Processing subscripts during loops
Sometimes when we are working, we want to get the subscripts of elements in the loop. Generally speaking, the more elegant way of writing is as follows:
lst = ["blue", "lightblue", "pink", "orange", "red"] for idx, item in enumerate(lst): print(idx, item)
13. Splicing multiple elements in the list
In Python, the Join() function is generally used to splice all the elements in the list together. Of course, we can also add splicing symbols when splicing. The example is as follows:
names = ["john", "sara", "jim", "rock"] print(", ".join(names))
14. Merge the two dictionaries
In addition, search the top Python background of the public account and reply "Advanced" to get a surprise gift package.
In Python we can use {**dict_name, **dict_name2, …} to merge multiple dictionaries. The example is as follows:
d1 = {"v1": 22, "v2": 33} d2 = {"v2": 44, "v3": 55} d3 = {**d1, **d2} print(d3)
The result is as follows:
{'v1': 22, 'v2': 44, 'v3': 55}
15 Use two lists to generate a dictionary
In Python, if we need to combine the corresponding elements in the two lists into a dictionary, then we can use the zip function to easily do this. The code is as follows:
keys = ['a', 'b', 'c'] vals = [1, 2, 3] zipped = dict(zip(keys, vals))
16. Sort the dictionary according to value
In Python we use the sorted() function to sort the dictionary according to its value. The code is as follows:
d = { "v1": 80, "v2": 20, "v3": 40, "v4": 20, "v5": 10, } sorted_d = dict(sorted(d.items(), key=lambda item: item[1])) print(sorted_d) 当然我们也可以使用itemgetter( )来替代上述 lambda函数,代码如下: from operator import itemgetter sorted_d = dict(sorted(d.items(), key=itemgetter(1)))
Further, we can also sort it in descending order by passing reverse=True:
sorted_d = dict(sorted(d.items(), key=itemgetter(1), reverse=True))
17, Pretty print
Using the Print() function in Python, sometimes the output It's ugly. At this time, we can use pprint to make the output more beautiful. The sample is as follows:
from pprint import pprint data = { "name": "john deo", "age": "22", "address": {"contry": "canada", "state": "an state of canada :)", "address": "street st.34 north 12"}, "attr": {"verified": True, "emialaddress": True}, } print(data) pprint(data)
The output is as follows:
{'name': 'john deo', 'age': '22', 'address': {'contry': 'canada', 'state': 'an state of canada :)', 'address': 'street st.34 north 12'}, 'attr': {'verified': True, 'emialaddress': True}} {'address': {'address': 'street st.34 north 12', 'contry': 'canada', 'state': 'an state of canada :)'}, 'age': '22', 'attr': {'emialaddress': True, 'verified': True}, 'name': 'john deo'}
It can be seen that using the pprint function can make the output of the dictionary easier to read.
The above is the detailed content of 17 useful Python tips to share!. For more information, please follow other related articles on the PHP Chinese website!
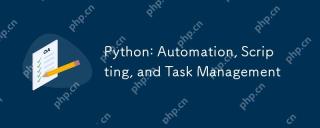
Python excels in automation, scripting, and task management. 1) Automation: File backup is realized through standard libraries such as os and shutil. 2) Script writing: Use the psutil library to monitor system resources. 3) Task management: Use the schedule library to schedule tasks. Python's ease of use and rich library support makes it the preferred tool in these areas.
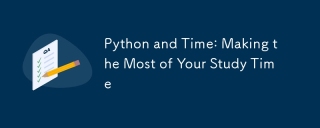
To maximize the efficiency of learning Python in a limited time, you can use Python's datetime, time, and schedule modules. 1. The datetime module is used to record and plan learning time. 2. The time module helps to set study and rest time. 3. The schedule module automatically arranges weekly learning tasks.
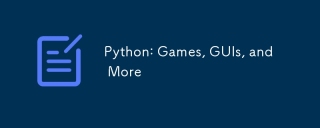
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.
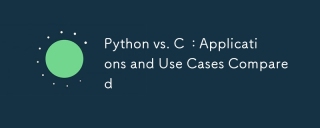
Python is suitable for data science, web development and automation tasks, while C is suitable for system programming, game development and embedded systems. Python is known for its simplicity and powerful ecosystem, while C is known for its high performance and underlying control capabilities.
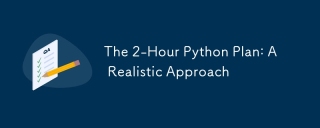
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
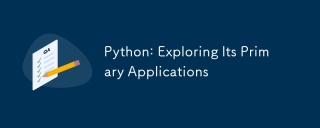
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
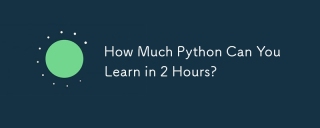
You can learn the basics of Python within two hours. 1. Learn variables and data types, 2. Master control structures such as if statements and loops, 3. Understand the definition and use of functions. These will help you start writing simple Python programs.
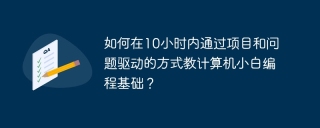
How to teach computer novice programming basics within 10 hours? If you only have 10 hours to teach computer novice some programming knowledge, what would you choose to teach...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
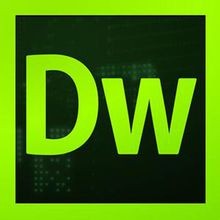
Dreamweaver CS6
Visual web development tools

Zend Studio 13.0.1
Powerful PHP integrated development environment
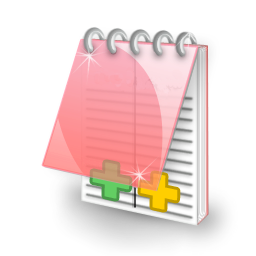
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.