PHP is a popular server-side scripting language used for developing web applications. In the process of developing web applications, it is often necessary to return data to the front end in JSON format. In PHP, converting raw data types to JSON is very convenient. This article will introduce how to convert an array into a JSON array in PHP.
- Use the json_encode() function
In PHP, use the json_encode() function to convert an array into JSON format. This function receives one parameter, which is the array to be converted, and the return value is a string in JSON format.
The following is a sample code that uses the json_encode() function to convert an array into a JSON array:
<code><?php $arr = array('name' => '张三', 'age' => 20, 'gender' => '男'); echo json_encode($arr); ?></code>
Running the above code will output the following results:
<code>{"name":"\u5f20\u4e09","age":20,"gender":"\u7537"}</code>
You can see Yes, the above code has successfully converted the array into a JSON array. The json_encode() function escapes all Unicode characters to ensure that the generated JSON data is legal.
- Processing Chinese characters
In practical applications, it is often necessary to process Chinese characters. When PHP's json_encode() function processes Chinese characters, it will convert the Chinese characters into Unicode characters. This will This causes some unfriendly-looking characters to appear in the JSON data.
In order to solve this problem, you can add the parameter JSON_UNESCAPED_UNICODE to the json_encode() function. This parameter tells the json_encode() function not to escape Chinese characters, thereby retaining the original Chinese characters.
The following is a sample code that uses the JSON_UNESCAPED_UNICODE parameter to convert an array into a JSON array:
<code><?php $arr = array('name' => '张三', 'age' => 20, 'gender' => '男'); echo json_encode($arr, JSON_UNESCAPED_UNICODE); ?></code>
Running the above code will output the following results:
<code>{"name":"张三","age":20,"gender":"男"}</code>
As you can see, using the JSON_UNESCAPED_UNICODE parameter After that, the Chinese characters in the JSON array have been encoded into original Chinese characters.
- Processing multi-dimensional arrays
In practical applications, sometimes it is necessary to convert multi-dimensional arrays into JSON arrays.
So we need to use a recursive function to first determine whether the current value is an array. If it is an array, then call the function recursively until the current value is not an array.
The following is a sample code that converts a multi-dimensional array into a JSON array:
<code><?php $arr = array( array('name' => '张三', 'age' => 20, 'gender' => '男'), array('name' => '李四', 'age' => 22, 'gender' => '女'), array('name' => '王五', 'age' => 24, 'gender' => '男') ); echo json_encode($arr, JSON_UNESCAPED_UNICODE); function array_to_json($array) { if (!is_array($array)) { return null; } $json = '['; foreach ($array as $key => $value) { if (is_array($value)) { $value = array_to_json($value); } else { $value = json_encode($value, JSON_UNESCAPED_UNICODE); } if ($json != '[') { $json .= ','; } $json .= $value; } $json .= ']'; return $json; } $new_arr = array( 'name' => '张三', 'info' => array( 'age' => 20, 'address' => array( 'province' => '广东省', 'city' => '深圳市' ) ) ); echo array_to_json($new_arr); ?></code>
Running the above code will output the following results:
<code>[{"name":"张三","age":20,"gender":"男"},{"name":"李四","age":22,"gender":"女"},{"name":"王五","age":24,"gender":"男"}] {"name":"张三","info":{"age":20,"address":{"province":"\u5e7f\u4e1c\u7701","city":"\u6df1\u5733\u5e02"}}}</code>
As you can see, through array_to_json( ) function, successfully converts a multi-dimensional array into a JSON array.
The above is the detailed content of php convert array into json array. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

SublimeText3 Chinese version
Chinese version, very easy to use
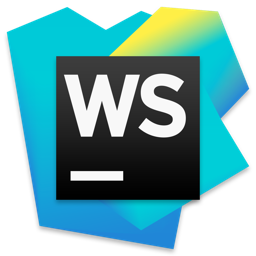
WebStorm Mac version
Useful JavaScript development tools

Zend Studio 13.0.1
Powerful PHP integrated development environment
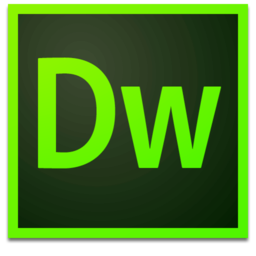
Dreamweaver Mac version
Visual web development tools
