As Laravel becomes one of the most popular PHP frameworks in web development, developers frequently need to determine whether different data exists. In Laravel, determining whether something exists is a basic task, and it can be used in a variety of different scenarios. This article will introduce how to determine the existence of various data in Laravel.
- Determine whether the data in the database exists
In Laravel, determining whether the data in the database exists is a common task. The following introduces two methods of querying whether data in the database exists through the Eloquent model:
Method 1: Use method chain to query and judge at the same time
Laravel provides us with many query constructors, which can Use them to build queries. In this example, we will use Eloquent's where method to query whether there is data that meets the conditions in the database:
use App\User; if(User::where('name', '=', 'john')->exists()){ // 如果数据存在 }else{ // 如果数据不存在 }
In the above code snippet, we use the exists() method to determine whether the query result set is exist. Returns true if the result set exists, false otherwise.
Method 2: Use the findOrFail method to find data
In Laravel, the findOrFail() method allows us to easily obtain the specified data from the database, and if the data does not exist, it will Throws ModelNotFoundException. The following is how the findOrFail method is used:
use App\User; try { $user = User::findOrFail(1); } catch (ModelNotFoundException $e) { dd("用户不存在"); }
In the above code snippet, if the user with id 1 does not exist, the ModelNotFoundException exception will be caught.
- Determine whether a file exists
In Laravel, determining whether a file exists is a common task. The following are two methods to determine whether a file exists:
Method 1: Use the file system to determine whether the file exists
Laravel has an abstraction layer of the file system, which allows us to easily Manipulate files, whether based on local or cloud storage. The following is how to use Laravel's file system to determine whether a file exists:
use Illuminate\Support\Facades\Storage; if(Storage::exists('file.txt')){ // 如果文件存在 }else{ // 如果文件不存在 }
In the above code, we use the exists method in Laravel's storage module to determine whether the file exists. Returns true if the file exists, false otherwise.
Method 2: Use PHP's file_exists function to determine whether the file exists
In addition to using Laravel's file system to operate files, we can also directly use PHP's file_exists() function to determine whether the file exists exist. The following is how to use the file_exists() function to determine whether a file exists:
if(file_exists('file.txt')){ // 如果文件存在 }else{ // 如果文件不存在 }
The effects of method one and method two are the same, and which method to use depends on the developer's personal preference.
- Determine whether a variable exists
In Laravel development, we often encounter situations where we need to determine whether a variable exists. In order to avoid errors caused by the variable not existing, we can use the isset() function to determine whether the variable is set. The following is how to use the isset function to determine whether a variable exists:
if(isset($variable)){ // 如果变量存在 }else{ // 如果变量不存在 }
In the above code, we use the isset() function to determine whether the variable $variable exists. Returns true if present, false otherwise.
- Determine whether an element in an array exists
In Laravel, determining whether an element in an array exists is also a relatively common task. We can use the in_array() function to determine whether the specified element exists in the array. The following is how to use the in_array() function to determine whether the specified element exists in the array:
$array = array("apple", "banana", "orange"); if(in_array("apple", $array)){ // 如果元素存在 }else{ // 如果元素不存在 }
In the above code, we use the in_array() function to determine whether the element "apple" exists in the array $array . Returns true if present, false otherwise.
Summary
In this article, we learned how to determine the existence of various data in Laravel development. Whether you need to determine whether data in the database exists in Laravel development, or whether you need to determine whether elements in files, variables, or arrays exist, this article provides you with detailed solutions. Hope this article can be helpful to you!
The above is the detailed content of How to determine the existence of various data in Laravel. For more information, please follow other related articles on the PHP Chinese website!

Laravel is suitable for building web applications quickly, while Python is suitable for a wider range of application scenarios. 1.Laravel provides EloquentORM, Blade template engine and Artisan tools to simplify web development. 2. Python is known for its dynamic types, rich standard library and third-party ecosystem, and is suitable for Web development, data science and other fields.

Laravel and Python each have their own advantages: Laravel is suitable for quickly building feature-rich web applications, and Python performs well in the fields of data science and general programming. 1.Laravel provides EloquentORM and Blade template engines, suitable for building modern web applications. 2. Python has a rich standard library and third-party library, and Django and Flask frameworks meet different development needs.

Laravel is worth choosing because it can make the code structure clear and the development process more artistic. 1) Laravel is based on PHP, follows the MVC architecture, and simplifies web development. 2) Its core functions such as EloquentORM, Artisan tools and Blade templates enhance the elegance and robustness of development. 3) Through routing, controllers, models and views, developers can efficiently build applications. 4) Advanced functions such as queue and event monitoring further improve application performance.

Laravel is not only a back-end framework, but also a complete web development solution. It provides powerful back-end functions, such as routing, database operations, user authentication, etc., and supports front-end development, improving the development efficiency of the entire web application.

Laravel is suitable for web development, Python is suitable for data science and rapid prototyping. 1.Laravel is based on PHP and provides elegant syntax and rich functions, such as EloquentORM. 2. Python is known for its simplicity, widely used in Web development and data science, and has a rich library ecosystem.

Laravelcanbeeffectivelyusedinreal-worldapplicationsforbuildingscalablewebsolutions.1)ItsimplifiesCRUDoperationsinRESTfulAPIsusingEloquentORM.2)Laravel'secosystem,includingtoolslikeNova,enhancesdevelopment.3)Itaddressesperformancewithcachingsystems,en

Laravel's core functions in back-end development include routing system, EloquentORM, migration function, cache system and queue system. 1. The routing system simplifies URL mapping and improves code organization and maintenance. 2.EloquentORM provides object-oriented data operations to improve development efficiency. 3. The migration function manages the database structure through version control to ensure consistency. 4. The cache system reduces database queries and improves response speed. 5. The queue system effectively processes large-scale data, avoid blocking user requests, and improve overall performance.

Laravel performs strongly in back-end development, simplifying database operations through EloquentORM, controllers and service classes handle business logic, and providing queues, events and other functions. 1) EloquentORM maps database tables through the model to simplify query. 2) Business logic is processed in controllers and service classes to improve modularity and maintainability. 3) Other functions such as queue systems help to handle complex needs.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
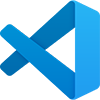
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
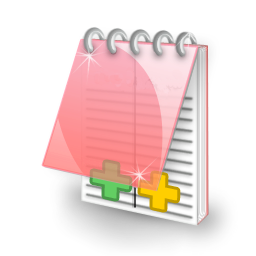
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function