Laravel is worth choosing because it can make the code structure clear and the development process more artistic. 1) Laravel is based on PHP, follows the MVC architecture, and simplifies web development. 2) Its core features such as Eloquent ORM, Artisan tools and Blade templates enhance the elegance and robustness of development. 3) Through routing, controllers, models and views, developers can efficiently build applications. 4) Advanced functions such as queue and event monitoring further improve application performance.
introduction
Laravel, a framework known as a well-known framework in the field of web development, aims to help developers build robust and elegant web applications. Why choose Laravel? Because it not only makes your code structure clear, but also makes the development process more artistic. Today, let’s take a deeper look at how Laravel achieves this goal, as well as the challenges and solutions that may be encountered during use.
Review of basic knowledge
Laravel is an open source web application framework based on PHP, following the MVC (model-view-controller) architecture design pattern. It aims to simplify the web development process and provides a range of tools and libraries that allow developers to focus more on application logic than infrastructure. The core concepts of Laravel include routing, controllers, models, views, and database migrations, which are the cornerstones for building modern web applications.
Core concept or function analysis
The core of Laravel: Elegance and Strongness
Laravel's design philosophy is to make web development a treat, not a chore. Its elegance is reflected in concise syntax and rich APIs, while its robustness comes from its powerful features, such as Eloquent ORM, Artisan command line tools, Blade template engine, etc.
Eloquent ORM
Eloquent ORM is Laravel's object-relational mapper, which makes database operations as simple as operating objects. You can interact with database tables by defining models without writing complex SQL queries.
// Define a User model class User extends Model { protected $table = 'users'; } <p>// Use Eloquent ORM to query data $user = User::find(1); echo $user->name;</p>
Eloquent ORM not only simplifies data operations, but also provides powerful relationship management functions, such as definition and query of one-to-one, one-to-many, and many-to-many relationships.
Artisan command line tool
Artisan is the command line interface of Laravel, which provides a large number of commands to assist in the development process. For example, creating controllers, models, migration files, etc. can all be done in one click through the Artisan command.
// Create a new controller php artisan make:controller UserController <p>// Create a new migration file php artisan make:migration create_users_table</p>
Artisan not only improves development efficiency, but also makes the development process more standardized and reduces human errors.
How it works
How Laravel works can be understood from its request processing flow and dependency injection mechanism. After the request enters the Laravel application, it first matches through the routing system and then passes it to the corresponding controller for processing. The controller obtains the required services and models through dependency injection, and after completing the business logic, it renders the results into the view and returns them to the user.
Dependency injection is the key to Laravel's loose coupling and testability. It allows developers to declare required dependencies in code, which are automatically injected by containers at runtime, avoiding hard-coded dependencies and improving code flexibility and maintainability.
Example of usage
Basic usage
Let's look at a simple Laravel application example showing how to create a basic user list page.
// Define route Route::get('/users', 'UserController@index'); <p>// Define index method in UserController class UserController extends Controller { public function index() { $users = User::all(); return view('users.index', compact('users')); } }</p><p> // Define the view in resources/views/users/index.blade.php </p>
-
@foreach($users as $user)
- {{ $user->name }} @endforeach
This example shows how to build a simple page using routes, controllers, models, and views.
Advanced Usage
Laravel also supports more complex functions, such as queues, event listening, task scheduling, etc. Let's look at an example of using queues to handle asynchronous tasks.
// Define a Job class in the App\Jobs directory class ProcessPodcast implements ShouldQueue { use Dispatchable, InteractsWithQueue, Queueable, SerializesModels; <pre class='brush:php;toolbar:false;'>protected $podcast; public function __construct(Podcast $podcast) { $this->podcast = $podcast; } public function handle() { // Handle the logic of podcast}
}
// Trigger queue task in the controller public function processPodcast(Podcast $podcast) { ProcessPodcast::dispatch($podcast); }
Using queues can improve application responsiveness and scalability, especially when dealing with time-consuming tasks.
Common Errors and Debugging Tips
When using Laravel, you may encounter some common problems, such as migration failure, dependency injection errors, permission problems, etc. Here are some debugging tips:
- Migration failed: Check the database connection configuration to ensure that the database user has sufficient permissions. Use
php artisan migrate:status
to view migration status. - Dependency injection error: Check the constructor of the controller or service provider to ensure that all dependencies are declared correctly. Use
php artisan tinker
for interactive debugging. - Permissions issue: Check the permissions of the storage directory to ensure that the web server user has read and write permissions. Create symbolic links using
php artisan storage:link
.
Performance optimization and best practices
In Laravel applications, performance optimization and best practices are key to ensuring that the application runs efficiently. Here are some suggestions:
- Using caching: Laravel provides a powerful caching system that can cache database query results, API responses, etc., reducing database load.
- Optimize database queries: Use Eloquent's eager loading to avoid N 1 query problems and use indexes to improve query speed.
- Code specification: Follow the PSR-2 encoding standard to maintain code consistency and readability. Use Laravel's code style guide and automation tools such as PHP-CS-Fixer.
// Example using cache $users = Cache::remember('users', 3600, function () { return User::all(); }); <p>// Optimize database query example $users = User::with('posts')->get(); // Use eager loading</p>
In the process of using Laravel, developers may encounter some challenges, such as learning curves, performance bottlenecks, etc., but through continuous practice and optimization, they can give full play to the advantages of Laravel and build a robust and elegant web application.
The above is the detailed content of Laravel's Purpose: Building Robust and Elegant Web Applications. For more information, please follow other related articles on the PHP Chinese website!

Laravel10introducesseveralkeyfeaturesthatenhancewebdevelopment.1)Lazycollectionsallowefficientprocessingoflargedatasetswithoutloadingallrecordsintomemory.2)The'make:model-and-migration'artisancommandsimplifiescreatingmodelsandmigrations.3)Integration

LaravelMigrationsshouldbeusedbecausetheystreamlinedevelopment,ensureconsistencyacrossenvironments,andsimplifycollaborationanddeployment.1)Theyallowprogrammaticmanagementofdatabaseschemachanges,reducingerrors.2)Migrationscanbeversioncontrolled,ensurin

Yes,LaravelMigrationisworthusing.Itsimplifiesdatabaseschemamanagement,enhancescollaboration,andprovidesversioncontrol.Useitforstructured,efficientdevelopment.

SoftDeletesinLaravelimpactperformancebycomplicatingqueriesandincreasingstorageneeds.Tomitigatetheseissues:1)Indexthedeleted_atcolumntospeedupqueries,2)Useeagerloadingtoreducequerycount,and3)Regularlycleanupsoft-deletedrecordstomaintaindatabaseefficie

Laravelmigrationsarebeneficialforversioncontrol,collaboration,andpromotinggooddevelopmentpractices.1)Theyallowtrackingandrollingbackdatabasechanges.2)Migrationsensureteammembers'schemasstaysynchronized.3)Theyencouragethoughtfuldatabasedesignandeasyre

Laravel's soft deletion feature protects data by marking records rather than actual deletion. 1) Add SoftDeletestrait and deleted_at fields to the model. 2) Use the delete() method to mark the delete and restore it using the restore() method. 3) Use withTrashed() or onlyTrashed() to include soft delete records when querying. 4) Regularly clean soft delete records that have exceeded a certain period of time to optimize performance.

LaravelMigrationsareversioncontrolfordatabaseschemas,allowingreproducibleandreversiblechanges.Tousethem:1)Createamigrationwith'phpartisanmake:migration',2)Defineschemachangesinthe'up()'methodandreversalin'down()',3)Applychangeswith'phpartisanmigrate'

Laravelmigrationsmayfailtorollbackduetodataintegrityissues,foreignkeyconstraints,orirreversibleactions.1)Dataintegrityissuescanoccurifamigrationaddsdatathatcan'tbeundone,likeacolumnwithadefaultvalue.2)Foreignkeyconstraintscanpreventrollbacksifrelatio


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
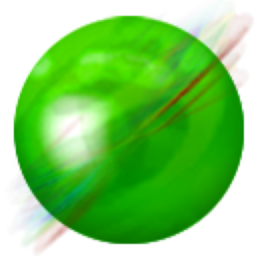
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

SublimeText3 Linux new version
SublimeText3 Linux latest version
