Soft Deletes in Laravel impact performance by complicating queries and increasing storage needs. To mitigate these issues: 1) Index the deleted_at column to speed up queries, 2) Use eager loading to reduce query count, and 3) Regularly clean up soft-deleted records to maintain database efficiency.
When dealing with Laravel's Soft Deletes, the primary performance concerns revolve around database queries and data management. Soft Deletes, while useful for maintaining data integrity without permanent deletion, can introduce overhead in terms of query complexity and storage.
Let's dive into the world of Soft Deletes in Laravel and explore how they can impact performance, along with some strategies to mitigate these issues.
Soft Deletes in Laravel offer a way to "delete" records without actually removing them from the database. Instead, a deleted_at
timestamp is added to the record, allowing for easy restoration if needed. This approach is fantastic for maintaining historical data and providing a safety net against accidental deletions. But, as with any feature, there are trade-offs, especially when it comes to performance.
When you start using Soft Deletes, your queries become a bit more complicated. Laravel automatically adds a WHERE deleted_at IS NULL
clause to your queries to ensure that "deleted" records aren't returned. This might seem trivial, but as your database grows, this additional condition can slow down your queries, especially if you're dealing with large datasets.
Consider this scenario: you're running a busy e-commerce platform with thousands of products. You've implemented Soft Deletes to manage product listings. Now, when you query the products table, Laravel adds that extra condition. On a small scale, it's not noticeable, but as your database scales, you might start to see performance degradation.
Here's a simple example of how Soft Deletes affect your queries:
// Without Soft Deletes $products = Product::all(); // With Soft Deletes $products = Product::whereNull('deleted_at')->get();
Now, let's talk about some strategies to keep your application humming along smoothly despite Soft Deletes.
One approach is to index the deleted_at
column. This can significantly speed up your queries, as the database can more efficiently filter out soft-deleted records. Here's how you might do it in a migration:
use Illuminate\Database\Migrations\Migration; use Illuminate\Database\Schema\Blueprint; class AddIndexToDeletedAtColumn extends Migration { public function up() { Schema::table('products', function (Blueprint $table) { $table->index('deleted_at'); }); } public function down() { Schema::table('products', function (Blueprint $table) { $table->dropIndex('deleted_at'); }); } }
Another strategy is to use eager loading when querying soft-deleted models. Eager loading can help reduce the number of queries executed, which is especially beneficial when dealing with related models. For example:
$products = Product::with('category')->whereNull('deleted_at')->get();
But what about those times when you actually want to include soft-deleted records in your results? Laravel provides the withTrashed()
method for this purpose:
$allProducts = Product::withTrashed()->get();
However, be cautious when using withTrashed()
as it can lead to unexpected results if not used carefully. Always consider the implications on your application's logic and performance.
One common pitfall I've encountered is the temptation to use Soft Deletes everywhere. While it's tempting to have that safety net, overusing Soft Deletes can lead to bloated databases and slower queries. It's crucial to evaluate whether Soft Deletes are truly necessary for each model. Sometimes, a simple hard delete might be more appropriate, especially for data that doesn't need to be restored.
Another aspect to consider is the impact on your application's memory usage. When dealing with large datasets, loading all records (including soft-deleted ones) into memory can be resource-intensive. Be mindful of this when designing your queries and consider using pagination or chunking to manage memory usage effectively.
Here's an example of how you might use chunking to process a large number of records:
Product::withTrashed()->chunk(100, function ($products) { foreach ($products as $product) { // Process each product } });
In terms of best practices, it's essential to regularly clean up soft-deleted records. Over time, these records can accumulate and impact performance. Implementing a scheduled task to permanently delete records that are no longer needed can help maintain a lean and efficient database. Here's how you might set up a simple cron job to do this:
// In your app/Console/Kernel.php file protected function schedule(Schedule $schedule) { $schedule->command('model:prune')->daily(); } // Create a custom command to handle pruning php artisan make:command PruneSoftDeletedRecords // In app/Console/Commands/PruneSoftDeletedRecords.php class PruneSoftDeletedRecords extends Command { protected $signature = 'model:prune'; public function handle() { Product::onlyTrashed()->where('deleted_at', '<', now()->subDays(30))->forceDelete(); } }
In conclusion, while Soft Deletes in Laravel offer a powerful way to manage data, they come with performance considerations that need to be addressed. By understanding how they impact your queries, indexing appropriately, using eager loading, and implementing regular cleanup, you can mitigate these performance issues and keep your application running smoothly. Remember, the key is to use Soft Deletes judiciously and always keep an eye on your database's health and performance.
The above is the detailed content of Laravel: Soft Deletes performance issues. For more information, please follow other related articles on the PHP Chinese website!

Laravel10introducesseveralkeyfeaturesthatenhancewebdevelopment.1)Lazycollectionsallowefficientprocessingoflargedatasetswithoutloadingallrecordsintomemory.2)The'make:model-and-migration'artisancommandsimplifiescreatingmodelsandmigrations.3)Integration

LaravelMigrationsshouldbeusedbecausetheystreamlinedevelopment,ensureconsistencyacrossenvironments,andsimplifycollaborationanddeployment.1)Theyallowprogrammaticmanagementofdatabaseschemachanges,reducingerrors.2)Migrationscanbeversioncontrolled,ensurin

Yes,LaravelMigrationisworthusing.Itsimplifiesdatabaseschemamanagement,enhancescollaboration,andprovidesversioncontrol.Useitforstructured,efficientdevelopment.

SoftDeletesinLaravelimpactperformancebycomplicatingqueriesandincreasingstorageneeds.Tomitigatetheseissues:1)Indexthedeleted_atcolumntospeedupqueries,2)Useeagerloadingtoreducequerycount,and3)Regularlycleanupsoft-deletedrecordstomaintaindatabaseefficie

Laravelmigrationsarebeneficialforversioncontrol,collaboration,andpromotinggooddevelopmentpractices.1)Theyallowtrackingandrollingbackdatabasechanges.2)Migrationsensureteammembers'schemasstaysynchronized.3)Theyencouragethoughtfuldatabasedesignandeasyre

Laravel's soft deletion feature protects data by marking records rather than actual deletion. 1) Add SoftDeletestrait and deleted_at fields to the model. 2) Use the delete() method to mark the delete and restore it using the restore() method. 3) Use withTrashed() or onlyTrashed() to include soft delete records when querying. 4) Regularly clean soft delete records that have exceeded a certain period of time to optimize performance.

LaravelMigrationsareversioncontrolfordatabaseschemas,allowingreproducibleandreversiblechanges.Tousethem:1)Createamigrationwith'phpartisanmake:migration',2)Defineschemachangesinthe'up()'methodandreversalin'down()',3)Applychangeswith'phpartisanmigrate'

Laravelmigrationsmayfailtorollbackduetodataintegrityissues,foreignkeyconstraints,orirreversibleactions.1)Dataintegrityissuescanoccurifamigrationaddsdatathatcan'tbeundone,likeacolumnwithadefaultvalue.2)Foreignkeyconstraintscanpreventrollbacksifrelatio


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

Zend Studio 13.0.1
Powerful PHP integrated development environment

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
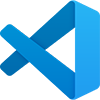
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
