Laravel is a very popular PHP framework that provides powerful ORM (Object Relational Mapping) tools that allow developers to manage databases more easily. Using Laravel ORM, you can define the database table structure through code instead of manually writing SQL statements. Laravel also provides a very convenient tool - the database structure generator, which can automatically generate the corresponding database table structure based on the model file. This article explains how to use the Laravel database schema generator.
1. Create model files
In Laravel, model files are usually stored in the Models
subdirectory under the app
directory. The first thing to do is to create a model file corresponding to the database table. It can be created through the Artisan command:
php artisan make:model Models/User
This will create a model file named User.php
in the app/Models
directory. In this file, you can use Eloquent ORM to define the database table structure as follows:
namespace App\Models; use Illuminate\Database\Eloquent\Model; class User extends Model { protected $table = 'users'; protected $fillable = [ 'name', 'email', 'password', ]; }
In this example, we define a User
model, which corresponds to users
surface. We also define the $fillable
attribute to limit the fields that can be batch assigned.
2. Create a data table
Once the model file is created, we can use the Artisan command to generate the corresponding data table:
php artisan migrate
This will be created in the databaseusers
table, the structure of this table will correspond to the User
model we defined.
3. Generate database structure
With the model file and data table, we can use the Laravel database structure generator to generate the corresponding database structure. Enter the following command in the console:
php artisan make:migration create_users_table --table=users
This will create a migration file named create_users_table
in the database/migrations
directory. We can define the table structure in this file using Laravel's database structure generator. The following is a typical generator code snippet:
public function up() { Schema::create('users', function (Blueprint $table) { $table->id(); $table->string('name'); $table->string('email')->unique(); $table->timestamp('email_verified_at')->nullable(); $table->string('password'); $table->rememberToken(); $table->timestamps(); }); }
In this example, we create the users
table through the Schema::create
method. The Blueprint
class provides many methods to define table structures, such as string
, integer
, boolean
, timestamp
, etc. wait.
Once the table structure is defined, we can run the following command to perform the migration:
php artisan migrate
This will create the table corresponding to the migration file we defined.
4. Conclusion
When developing using Laravel ORM, using the database structure generator can greatly improve development efficiency. It eliminates the tediousness of manually writing SQL statements and also ensures consistency between data tables and model files. The data table structure can be easily updated by simply modifying the model file. In Laravel, this is all very easy to do, just follow simple steps.
The above is the detailed content of How to use Laravel database structure generator. For more information, please follow other related articles on the PHP Chinese website!

In Laravel full-stack development, effective methods for managing APIs and front-end logic include: 1) using RESTful controllers and resource routing management APIs; 2) processing front-end logic through Blade templates and Vue.js or React; 3) optimizing performance through API versioning and paging; 4) maintaining the separation of back-end and front-end logic to ensure maintainability and scalability.

Totackleculturalintricaciesindistributedteams,fosteranenvironmentcelebratingdifferences,bemindfulofcommunication,andusetoolsforclarity.1)Implementculturalexchangesessionstosharestoriesandtraditions.2)Adjustcommunicationmethodstosuitculturalpreference

Toassesstheeffectivenessofremotecommunication,focuson:1)Engagementmetricslikemessagefrequencyandresponsetime,2)Sentimentanalysistogaugeemotionaltone,3)Meetingeffectivenessthroughattendanceandactionitems,and4)Networkanalysistounderstandcommunicationpa

Toprotectsensitivedataindistributedteams,implementamulti-facetedapproach:1)Useend-to-endencryptionforsecurecommunication,2)Applyrole-basedaccesscontrol(RBAC)tomanagepermissions,3)Encryptdataatrestwithkeymanagementtools,and4)Fosterasecurity-consciousc

No, emailisnotthebostforremotecollaborationToday.Modern platformlack, Microsoft teams, Zoom, ASANA, AndTrelloFhertreal-Time Communication, Project management, Andintegrationfeaturesthancteamworkandefficiency.

Collaborative document editing is an effective tool for distributed teams to optimize their workflows. It improves communication and project progress through real-time collaboration and feedback loops, and common tools include Google Docs, Microsoft Teams, and Notion. Pay attention to challenges such as version control and learning curve when using it.

ThepreviousversionofLaravelissupportedwithbugfixesforsixmonthsandsecurityfixesforoneyearafteranewmajorversion'srelease.Understandingthissupporttimelineiscrucialforplanningupgrades,ensuringprojectstability,andleveragingnewfeaturesandsecurityenhancemen

Laravelcanbeeffectivelyusedforbothfrontendandbackenddevelopment.1)Backend:UtilizeLaravel'sEloquentORMforsimplifieddatabaseinteractions.2)Frontend:LeverageBladetemplatesforcleanHTMLandintegrateVue.jsfordynamicSPAs,ensuringseamlessfrontend-backendinteg


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!
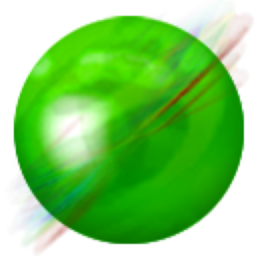
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

SublimeText3 Chinese version
Chinese version, very easy to use
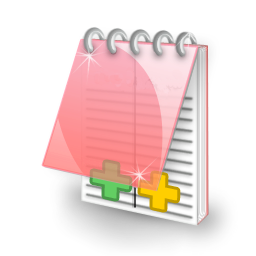
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
