Laravel is a PHP web application development framework that is widely popular among developers. It is known for its simplicity, elegance and excellent functionality, while also being flexible, scalable and efficient. The Laravel framework provides various developer tools and features, one of which is setting default values. Next we will focus on how to set default values in the Laravel framework.
Setting Default Values in Laravel
The Laravel framework provides many ways to set default values, depending on where you need to set default values. In the following sections, we'll detail how to set default values.
1. Set default values in the configuration file
Laravel provides various configuration files in the config folder, such as app.php and database.php. These files typically store application-related constants and parameters. You can easily access these parameters in your application using the config function. In order to set the default value, you can define the corresponding key and value in the corresponding configuration file, as in the following example:
'welcome_message' => 'Welcome to my Laravel application!'
In this example, the key is 'welcome_message' and the value is 'Welcome to my Laravel application!'. The default value of this key is the string "Welcome to my Laravel application!"
You can use the config function to obtain this default value, and use the default value as a rollback option when accessing the welcome_message configuration value. Here is a code example to get this default value:
$message = config('app.welcome_message', 'Default welcome message');
In this example, we use the config function to get the value of the 'welcome_message' key. If the value is found, it is assigned to the $message variable. If the value is not found, the "Default welcome message" string will be used as the rollback option.
2. Set default values in the model
In the Laravel framework, the model is the core of accessing the database. Models typically correspond to tables in a relational database, and they provide methods for reading, writing, and updating data in the tables. If you need to set default values in the model, you can use the boot method provided by the Eloquent model. The following is an example of implementing this method:
class User extends Model { protected $fillable = [ 'name', 'email', 'password', 'phone', ]; protected static function boot() { parent::boot(); static::creating(function ($user) { $user->phone = $user->phone ?? '1234567890'; }); } }
The boot method of this User model will be called when a new instance is created. In this example, we check the $phone property and set it to the string '1234567890' using the null coalescing operator. Every time a new User model is created, the default value of the 'phone' attribute will be '1234567890'.
3. Set default values in requests
When processing HTTP requests, you may need to define default values to handle parameters transmitted from the request. The Laravel framework provides multiple methods to obtain HTTP request parameters, including values or corresponding returned messages.
Here, we use the Illuminate\Http\Request instance to get the HTTP request parameters. If the parameter is not found, the default value will be used, here is the example:
public function update(Request $request, $id) { $name = $request->input('name', 'Lucy'); ... }
In this example, when the client sends the 'name' parameter to this method through the HTTP request, we use the $input method Retrieve the 'name' parameter from the $request instance. If there is no parameter named 'name', we use the 'Lucky' string as the default value.
4. Set default values in the form
Finally, you may need to set default values in the form to display on the HTML form component. To set default values, you can use HTML form helpers without having to hardcode HTML form elements.
{!! Form::text('name', $user->name ?? 'Lucky') !!}
In this example, we use the form helper provided by Laravel to create HTML input elements. In the parameters, we specified the name of the text box as 'name' and set 'user->name' as the default value. If 'user->name' does not exist, the 'Lucy' string is used as the default value.
Summary
In the Laravel framework, setting default values is a common task. Whether in a configuration file, in a model, in a request, or in a form, the Laravel framework provides various methods to set default values easily. By using the built-in functions and features of the Laravel framework, you can improve the readability, maintainability, and scalability of your application.
The above is the detailed content of How to set default value in Laravel. For more information, please follow other related articles on the PHP Chinese website!

Selecting Laravel or Python depends on the project requirements: 1) If you need to quickly develop web applications and use ORM and authentication systems, choose Laravel; 2) If it involves data analysis, machine learning or scientific computing, choose Python.

Laravel is suitable for building web applications quickly, and Python is suitable for projects that require flexibility and versatility. 1) Laravel provides rich features such as ORM and routing, suitable for the PHP ecosystem. 2) Python is known for its concise syntax and a powerful library ecosystem, and is suitable for fields such as web development and data science.

Use Laravel and PHP to create dynamic websites efficiently and fun. 1) Laravel follows the MVC architecture, and the Blade template engine simplifies HTML writing. 2) The routing system and request processing mechanism make URL definition and user input processing simple. 3) EloquentORM simplifies database operations. 4) The use of database migration, CRUD operations and Blade templates are demonstrated through the blog system example. 5) Laravel provides powerful user authentication and authorization functions. 6) Debugging skills include using logging systems and Artisan tools. 7) Performance optimization suggestions include lazy loading and caching.

Laravel realizes full-stack development through the Blade template engine, EloquentORM, Artisan tools and LaravelMix: 1. Blade simplifies front-end development; 2. Eloquent simplifies database operations; 3. Artisan improves development efficiency; 4. LaravelMix manages front-end resources.

Laravel is a modern PHP-based framework that follows the MVC architecture model, provides rich tools and functions, and simplifies the web development process. 1) It contains EloquentORM for database interaction, 2) Artisan command line interface for fast code generation, 3) Blade template engine for efficient view development, 4) Powerful routing system for defining URL structure, 5) Authentication system for user management, 6) Event listening and broadcast for real-time functions, 7) Cache and queue systems for performance optimization, making it easier and more efficient to build and maintain modern web applications.

Laravel is suitable for building web applications quickly, while Python is suitable for a wider range of application scenarios. 1.Laravel provides EloquentORM, Blade template engine and Artisan tools to simplify web development. 2. Python is known for its dynamic types, rich standard library and third-party ecosystem, and is suitable for Web development, data science and other fields.

Laravel and Python each have their own advantages: Laravel is suitable for quickly building feature-rich web applications, and Python performs well in the fields of data science and general programming. 1.Laravel provides EloquentORM and Blade template engines, suitable for building modern web applications. 2. Python has a rich standard library and third-party library, and Django and Flask frameworks meet different development needs.

Laravel is worth choosing because it can make the code structure clear and the development process more artistic. 1) Laravel is based on PHP, follows the MVC architecture, and simplifies web development. 2) Its core functions such as EloquentORM, Artisan tools and Blade templates enhance the elegance and robustness of development. 3) Through routing, controllers, models and views, developers can efficiently build applications. 4) Advanced functions such as queue and event monitoring further improve application performance.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor
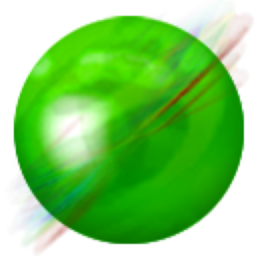
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
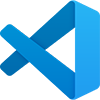
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.