Laravel is a popular PHP framework for building web applications. Setting HTTP response headers is a common need in web development because response headers can contain important information about the response. In this post, we will discuss how to set response headers in Laravel.
Laravel provides an easy way to set response headers. Simply use the second parameter of the response constructor to pass an array of headers.
return response($content) ->header('Content-Type', $type);
In the above example, we create a response and set its Content-Type header to the value of the $type variable.
In addition, we can also use the withHeader method to set the header.
return response($content) ->withHeader('Content-Type', $type);
The withHeader method is essentially the same as the header method, but it can be called continuously to set multiple headers. For example:
return response($content) ->withHeader('Content-Type', $type) ->withHeader('X-Content-Version', 'v1');
In the above example, we set two headers, Content-Type and X-Content-Version.
In addition to these methods, Laravel also provides a convenient way to set Cross-Origin Resource Sharing (CORS) headers.
return response($content) ->header('Access-Control-Allow-Origin', '*');
In the above example, we set the Access-Control-Allow-Origin header to *, indicating that requests from any origin are allowed.
If you need to set other CORS headers, such as Access-Control-Allow-Methods or Access-Control-Allow-Headers, you can use Laravel's cors middleware. In your application's routes file, attach this middleware to the appropriate route:
Route::middleware('cors')->get('/example', function () { return response('Hello World', 200); });
In the above example, we are using the cors middleware with the GET request in the route. Now we can see the allowed CORS headers in the response headers.
There are many situations where you need to set global headers in your Laravel application. You can use the following code in your application's boot method:
public function boot() { header('X-Frame-Options: SAMEORIGIN'); }
In the above example, we set the X-Frame-Options header to SAMEORIGIN to allow embedding of our application in the same origin.
Summary
Laravel provides various flexible ways to set HTTP response headers. You can set single or multiple headers using the response constructor, the withHeader method, or Laravel's cors middleware. Additionally, you can use PHP's built-in header function to set global headers. Now that you've learned how to set HTTP response headers, let's start using them in your application!
The above is the detailed content of How to set header in laravel. For more information, please follow other related articles on the PHP Chinese website!

Laravel stands out by simplifying the web development process and delivering powerful features. Its advantages include: 1) concise syntax and powerful ORM system, 2) efficient routing and authentication system, 3) rich third-party library support, allowing developers to focus on writing elegant code and improve development efficiency.

Laravelispredominantlyabackendframework,designedforserver-sidelogic,databasemanagement,andAPIdevelopment,thoughitalsosupportsfrontenddevelopmentwithBladetemplates.

Laravel and Python have their own advantages and disadvantages in terms of performance and scalability. Laravel improves performance through asynchronous processing and queueing systems, but due to PHP limitations, there may be bottlenecks when high concurrency is present; Python performs well with the asynchronous framework and a powerful library ecosystem, but is affected by GIL in a multi-threaded environment.

Laravel is suitable for projects that teams are familiar with PHP and require rich features, while Python frameworks depend on project requirements. 1.Laravel provides elegant syntax and rich features, suitable for projects that require rapid development and flexibility. 2. Django is suitable for complex applications because of its "battery inclusion" concept. 3.Flask is suitable for fast prototypes and small projects, providing great flexibility.

Laravel can be used for front-end development. 1) Use the Blade template engine to generate HTML. 2) Integrate Vite to manage front-end resources. 3) Build SPA, PWA or static website. 4) Combine routing, middleware and EloquentORM to create a complete web application.

PHP and Laravel can be used to build efficient server-side applications. 1.PHP is an open source scripting language suitable for web development. 2.Laravel provides routing, controller, EloquentORM, Blade template engine and other functions to simplify development. 3. Improve application performance and security through caching, code optimization and security measures. 4. Test and deployment strategies to ensure stable operation of applications.

Laravel and Python have their own advantages and disadvantages in terms of learning curve and ease of use. Laravel is suitable for rapid development of web applications. The learning curve is relatively flat, but it takes time to master advanced functions. Python's grammar is concise and the learning curve is flat, but dynamic type systems need to be cautious.

Laravel's advantages in back-end development include: 1) elegant syntax and EloquentORM simplify the development process; 2) rich ecosystem and active community support; 3) improved development efficiency and code quality. Laravel's design allows developers to develop more efficiently and improve code quality through its powerful features and tools.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
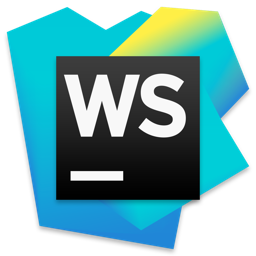
WebStorm Mac version
Useful JavaScript development tools

Atom editor mac version download
The most popular open source editor
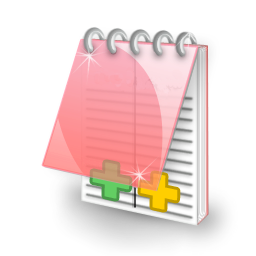
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software