In Golang, method coverage is an important way to achieve polymorphism. In this article, we will discuss method overriding in Golang and how to use it correctly.
In Golang, every method belongs to a type. If a type defines a method that is the same as another type, then this method can be called an overriding method because it overrides the method defined by the other type. A method of a type can override a method of its supertype with the same name and signature. In Golang, the signature of a method consists of the name of the method and the parameter types.
For example, we define a structure of type Shape, which has a CalcArea method, used to calculate the area of the shape:
type Shape struct { color string } func (s Shape) CalcArea() float64 { return 0 }
Now we define a Circle structure, which has a CalcArea method with the same name and parameters:
type Circle struct { Shape radius float64 } func (c Circle) CalcArea() float64 { return math.Pi * c.radius * c.radius }
In this example, the Circle type defines a CalcArea method with the same name and signature as the parent type Shape, so that it overrides the method defined by the parent type. Now, when we call the CalcArea method of a Circle instance, it will call the overridden method instead of the CalcArea method from the parent type.
In Golang, method coverage is an important way to achieve polymorphism through interfaces. If a type implements an interface and the interface defines a method, the type can redefine that method, thereby overriding the interface's method. This allows us to maintain consistent behavior between different instances of a type.
The following is an example of implementing an interface:
type Shape interface { CalcArea() float64 } type Circle struct { radius float64 } func (c Circle) CalcArea() float64 { return math.Pi * c.radius * c.radius }
In this example, the Circle type implements the Shape interface, and the interface defines a CalcArea method. The CalcArea method here is the same as the previous example, but now it allows us to call the CalcArea method on variables of type Shape, not just variables of type Circle.
In Golang, you can reuse methods of a type by embedding it. If a type contains another type as its field, then the type will automatically have methods of the embedded type. If the embedded type and the current type have methods with the same name, the current type's methods override the embedded type's methods.
Here is an example:
type Shape struct { color string } func (s Shape) ShowColor() { fmt.Println(s.color) } type Circle struct { Shape radius float64 } func (c Circle) ShowColor() { fmt.Println("Circle color:", c.color) }
In this example, we define a ShowColor method, explaining how to override methods in embedded types. The Circle type contains a field of type Shape, and the ShowColor method of the embedded type is displayed. Now, when we call the ShowColor method of a variable of type Circle, it will print out the correct color of type Circle, not the color of type Shape.
In Golang, method coverage is a very powerful programming technique that allows us to achieve polymorphism and code reuse. However, we need to use it carefully to ensure correct behavior. In practice, we should overload methods that meet expectations to avoid unnecessary errors.
The above is the detailed content of Discuss the use of method coverage in Golang. For more information, please follow other related articles on the PHP Chinese website!
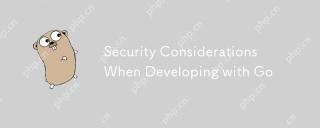
Gooffersrobustfeaturesforsecurecoding,butdevelopersmustimplementsecuritybestpracticeseffectively.1)UseGo'scryptopackageforsecuredatahandling.2)Manageconcurrencywithsynchronizationprimitivestopreventraceconditions.3)SanitizeexternalinputstoavoidSQLinj
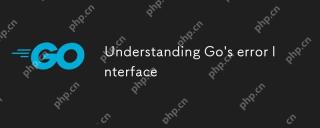
Go's error interface is defined as typeerrorinterface{Error()string}, allowing any type that implements the Error() method to be considered an error. The steps for use are as follows: 1. Basically check and log errors, such as iferr!=nil{log.Printf("Anerroroccurred:%v",err)return}. 2. Create a custom error type to provide more information, such as typeMyErrorstruct{MsgstringDetailstring}. 3. Use error wrappers (since Go1.13) to add context without losing the original error message,
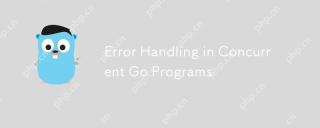
ToeffectivelyhandleerrorsinconcurrentGoprograms,usechannelstocommunicateerrors,implementerrorwatchers,considertimeouts,usebufferedchannels,andprovideclearerrormessages.1)Usechannelstopasserrorsfromgoroutinestothemainfunction.2)Implementanerrorwatcher
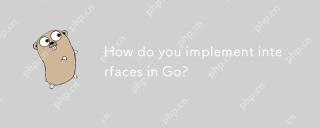
In Go language, the implementation of the interface is performed implicitly. 1) Implicit implementation: As long as the type contains all methods defined by the interface, the interface will be automatically satisfied. 2) Empty interface: All types of interface{} types are implemented, and moderate use can avoid type safety problems. 3) Interface isolation: Design a small but focused interface to improve the maintainability and reusability of the code. 4) Test: The interface helps to unit test by mocking dependencies. 5) Error handling: The error can be handled uniformly through the interface.
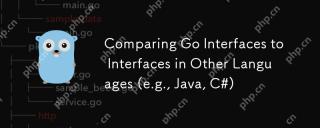
Go'sinterfacesareimplicitlyimplemented,unlikeJavaandC#whichrequireexplicitimplementation.1)InGo,anytypewiththerequiredmethodsautomaticallyimplementsaninterface,promotingsimplicityandflexibility.2)JavaandC#demandexplicitinterfacedeclarations,offeringc
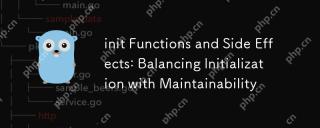
Toensureinitfunctionsareeffectiveandmaintainable:1)Minimizesideeffectsbyreturningvaluesinsteadofmodifyingglobalstate,2)Ensureidempotencytohandlemultiplecallssafely,and3)Breakdowncomplexinitializationintosmaller,focusedfunctionstoenhancemodularityandm
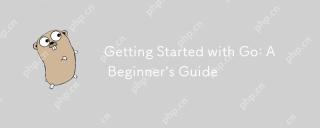
Goisidealforbeginnersandsuitableforcloudandnetworkservicesduetoitssimplicity,efficiency,andconcurrencyfeatures.1)InstallGofromtheofficialwebsiteandverifywith'goversion'.2)Createandrunyourfirstprogramwith'gorunhello.go'.3)Exploreconcurrencyusinggorout
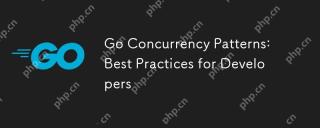
Developers should follow the following best practices: 1. Carefully manage goroutines to prevent resource leakage; 2. Use channels for synchronization, but avoid overuse; 3. Explicitly handle errors in concurrent programs; 4. Understand GOMAXPROCS to optimize performance. These practices are crucial for efficient and robust software development because they ensure effective management of resources, proper synchronization implementation, proper error handling, and performance optimization, thereby improving software efficiency and maintainability.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
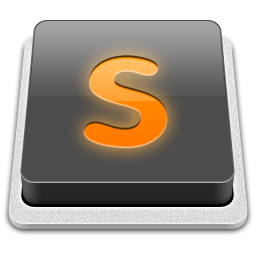
SublimeText3 Mac version
God-level code editing software (SublimeText3)

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
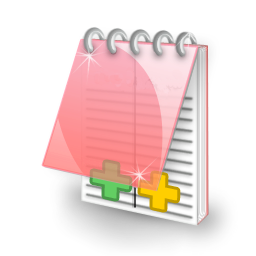
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
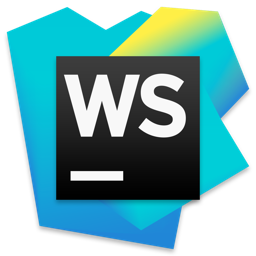
WebStorm Mac version
Useful JavaScript development tools
