With the rapid development of e-commerce, the demand for express delivery business is also increasing. Many companies take express delivery as their main business, and the realization of express mailing functions has become a necessary function for many companies. This article will introduce how to implement the express delivery function in the Vue project to help developers better complete related business needs.
1. Select Express API
To implement the express mailing function in the Vue project, you need to use a third-party express API. Currently, there are many optional express APIs on the market, such as aggregate data, Express 100, etc. Developers can choose the express API that suits them based on the actual situation of their projects.
2. Express Query Interface
The first step to realize the express mailing function is to query the express delivery information. It is necessary to provide users with an interface for querying the express delivery information. Generally, the selected express API will provide such an interface, and the returned data format is JSON format. To make interface calls in the Vue project, you can use network request libraries such as axios to obtain data. Simple interface example for querying express delivery information:
getData() { const url = "https://apis.juhe.cn/express/query"; // 接口地址 const params = { com: "shentong", // 快递公司的编码 no: "1234567890", // 快递单号 key: "*************" // 接口的key }; axios.get(url, {params}) .then(response => { console.log(response.data); // 打印接口返回的数据 }) .catch(error => { console.log(error); }); }
3. Express ordering interface
After querying express delivery information, the next function we need to provide users is to place express delivery orders. Compared with querying express delivery information, it is more difficult to place an express delivery order. The information that needs to be entered when placing an order generally includes sender information, recipient information, courier company, courier number, mobile phone number, etc. According to the information filled in by the user, the data is passed to the ordering interface of the Express API to complete the ordering operation.
The data format for placing orders is usually also in JSON format, and the data needs to be encapsulated into a JSON object. According to the order interface document provided by the express API, define an example of the order interface:
submitOrder() { const url = "https://apis.juhe.cn/express/order"; // 接口地址 const params = { sendMan: "张三", sendMobile: "13312345678", sendAddress: "广东省深圳市罗湖区", receiveMan: "李四", receiveMobile: "13512345678", receiveAddress: "湖北省武汉市洪山区", courierCode: "shentong", courierNumber: "1234567890", key: "*************" }; axios.post(url, params) .then(response => { console.log(response.data); // 打印接口返回的数据 }) .catch(error => { console.log(error); }); }
4. Express list display
In order to facilitate users’ subsequent express tracking and query, we need to use the Vue framework to Express delivery information is displayed. Before data display, the queried courier information and order information need to be stored. You can use VueX to share state between components. For smaller projects, you can directly use Vue's data binding to achieve it.
Express delivery list display is generally divided into two types: unsigned and signed. Classification display can be performed by judging the status of the express delivery, as follows:
Not signed for:
-
快递公司:{{item.com}}
快递单号:{{item.no}}
状态:{{item.state}}
Signed for:
-
快递公司:{{item.com}}
快递单号:{{item.no}}
状态:{{item.state}}
5. Express delivery status query
In order to facilitate users to track the status of express delivery at any time, we need to set up an entrance to query the status of express delivery. Similarly, when querying the express status, you need to call the express query interface provided by the express API, and the returned data format is also in JSON format. Example of the interface for querying express delivery status:
getExpressStatus() { const url = "https://apis.juhe.cn/express/query"; // 接口地址 const params = { com: "shentong", // 快递公司的编码 no: "1234567890", // 快递单号 key: "*************" // 接口的key }; axios.get(url, {params}) .then(response => { console.log(response.data); // 打印接口返回的数据 }) .catch(error => { console.log(error); }); }
6. Summary
This article introduces the method of implementing the express mailing function in the Vue project. Through third-party express delivery API calls and Vue framework data display, we can quickly and easily implement express delivery functions, making it convenient for users to use and track express delivery status.
The above is the detailed content of How to do express delivery in vue project. For more information, please follow other related articles on the PHP Chinese website!

React is a JavaScript library for building modern front-end applications. 1. It uses componentized and virtual DOM to optimize performance. 2. Components use JSX to define, state and attributes to manage data. 3. Hooks simplify life cycle management. 4. Use ContextAPI to manage global status. 5. Common errors require debugging status updates and life cycles. 6. Optimization techniques include Memoization, code splitting and virtual scrolling.

React's future will focus on the ultimate in component development, performance optimization and deep integration with other technology stacks. 1) React will further simplify the creation and management of components and promote the ultimate in component development. 2) Performance optimization will become the focus, especially in large applications. 3) React will be deeply integrated with technologies such as GraphQL and TypeScript to improve the development experience.

React is a JavaScript library for building user interfaces. Its core idea is to build UI through componentization. 1. Components are the basic unit of React, encapsulating UI logic and styles. 2. Virtual DOM and state management are the key to component work, and state is updated through setState. 3. The life cycle includes three stages: mount, update and uninstall. The performance can be optimized using reasonably. 4. Use useState and ContextAPI to manage state, improve component reusability and global state management. 5. Common errors include improper status updates and performance issues, which can be debugged through ReactDevTools. 6. Performance optimization suggestions include using memo, avoiding unnecessary re-rendering, and using us

Using HTML to render components and data in React can be achieved through the following steps: Using JSX syntax: React uses JSX syntax to embed HTML structures into JavaScript code, and operates the DOM after compilation. Components are combined with HTML: React components pass data through props and dynamically generate HTML content, such as. Data flow management: React's data flow is one-way, passed from the parent component to the child component, ensuring that the data flow is controllable, such as App components passing name to Greeting. Basic usage example: Use map function to render a list, you need to add a key attribute, such as rendering a fruit list. Advanced usage example: Use the useState hook to manage state and implement dynamics

React is the preferred tool for building single-page applications (SPAs) because it provides efficient and flexible ways to build user interfaces. 1) Component development: Split complex UI into independent and reusable parts to improve maintainability and reusability. 2) Virtual DOM: Optimize rendering performance by comparing the differences between virtual DOM and actual DOM. 3) State management: manage data flow through state and attributes to ensure data consistency and predictability.

React is a JavaScript library developed by Meta for building user interfaces, with its core being component development and virtual DOM technology. 1. Component and state management: React manages state through components (functions or classes) and Hooks (such as useState), improving code reusability and maintenance. 2. Virtual DOM and performance optimization: Through virtual DOM, React efficiently updates the real DOM to improve performance. 3. Life cycle and Hooks: Hooks (such as useEffect) allow function components to manage life cycles and perform side-effect operations. 4. Usage example: From basic HelloWorld components to advanced global state management (useContext and

The React ecosystem includes state management libraries (such as Redux), routing libraries (such as ReactRouter), UI component libraries (such as Material-UI), testing tools (such as Jest), and building tools (such as Webpack). These tools work together to help developers develop and maintain applications efficiently, improve code quality and development efficiency.

React is a JavaScript library developed by Facebook for building user interfaces. 1. It adopts componentized and virtual DOM technology to improve the efficiency and performance of UI development. 2. The core concepts of React include componentization, state management (such as useState and useEffect) and the working principle of virtual DOM. 3. In practical applications, React supports from basic component rendering to advanced asynchronous data processing. 4. Common errors such as forgetting to add key attributes or incorrect status updates can be debugged through ReactDevTools and logs. 5. Performance optimization and best practices include using React.memo, code segmentation and keeping code readable and maintaining dependability


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
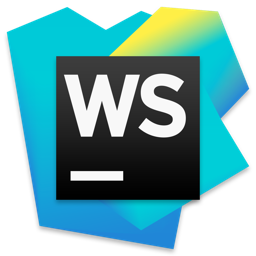
WebStorm Mac version
Useful JavaScript development tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.