In recent years, blockchain technology has been paid attention to and used by more and more people. Among them, the application of digital currency is also becoming more and more widespread. One of the core technologies of blockchain, smart contracts, has become the key to realizing transfers between digital currencies. This article will introduce how to implement digital currency transfer using Golang language.
1. Golang language
Golang, referred to as Go, is an open source programming language launched by Google. It provides simple and easy-to-use programming syntax, supports multi-threading, has strong security, and is easy to expand. Therefore, Golang language is widely used in cloud computing, data storage, network communications, API development and other fields.
2. Smart Contract for Digital Currency Transfer
Smart contract is a special program code that can be stored on the blockchain and used to implement trusted transaction logic. Digital currency transfer is one of the typical cases. The core idea is to store currency transaction information in smart contracts during the transfer process, and use blockchain technology to record the process of each transaction. After each transfer is completed, the correct transaction of currency can be achieved through the recorded information of the smart contract.
3. The process of realizing digital currency transfer in Golang
- Creating an account
In Golang, we can use struct to create an account and record it The account's private and public keys. The private key is the key information used for account signature, while the public key is used for account verification and transaction record access.
type account struct { privateKey string publicKey string }
- Create Transaction
Transaction refers to the transfer process of digital currency. In Golang, we can use struct to create a transaction and record the sender, receiver, quantity, timestamp and other information of the transaction.
type transaction struct { sender string receiver string amount float32 time int64 }
- Generate transaction signature
Transaction signature is the signature information generated using the private key during the transaction process to ensure the security of the transaction. In Golang, we can use the RSA algorithm in the crypto library to generate transaction signatures.
func generateSignature(privateKey string, data []byte) ([]byte, error) { key, err := parseRSAPrivateKeyFromPEM(privateKey) if err != nil { return nil, err } h := crypto.SHA256.New() h.Write(data) hashed := h.Sum(nil) signature, err := rsa.SignPKCS1v15(rand.Reader, key, crypto.SHA256, hashed) if err != nil { return nil, err } return signature, nil }
- Send transaction
In Golang, we can use the http library to construct a request to send a transaction and send it using RPC. Specifically, Golang will call the blockchain node through RPC to verify and record transactions.
func sendTransaction(tx *transaction, privateKey string, endpoint string) error { data, _ := json.Marshal(tx) signature, err := generateSignature(privateKey, data) if err != nil { return err } signedTx := base64.StdEncoding.EncodeToString(signature) + "." + base64.StdEncoding.EncodeToString(data) body := strings.NewReader(signedTx) req, _ := http.NewRequest("POST", endpoint, body) client := &http.Client{} resp, err := client.Do(req) if err != nil { return err } defer resp.Body.Close() return nil }
4. Summary
Golang is a programming language that is very suitable for blockchain application development. It is easy to use, efficient, secure, and scalable, making digital Smart contract implementation for currency transfers just got easier. This will contribute to the wider application and development of blockchain technology.
The above is the detailed content of How to implement digital currency transfer in golang. For more information, please follow other related articles on the PHP Chinese website!
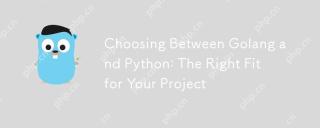
Golangisidealforperformance-criticalapplicationsandconcurrentprogramming,whilePythonexcelsindatascience,rapidprototyping,andversatility.1)Forhigh-performanceneeds,chooseGolangduetoitsefficiencyandconcurrencyfeatures.2)Fordata-drivenprojects,Pythonisp
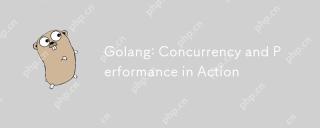
Golang achieves efficient concurrency through goroutine and channel: 1.goroutine is a lightweight thread, started with the go keyword; 2.channel is used for secure communication between goroutines to avoid race conditions; 3. The usage example shows basic and advanced usage; 4. Common errors include deadlocks and data competition, which can be detected by gorun-race; 5. Performance optimization suggests reducing the use of channel, reasonably setting the number of goroutines, and using sync.Pool to manage memory.
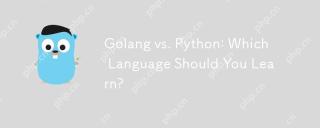
Golang is more suitable for system programming and high concurrency applications, while Python is more suitable for data science and rapid development. 1) Golang is developed by Google, statically typing, emphasizing simplicity and efficiency, and is suitable for high concurrency scenarios. 2) Python is created by Guidovan Rossum, dynamically typed, concise syntax, wide application, suitable for beginners and data processing.
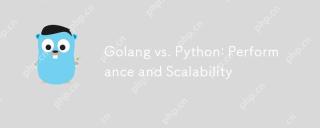
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
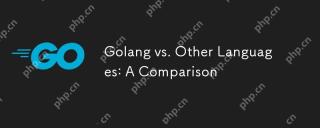
Go language has unique advantages in concurrent programming, performance, learning curve, etc.: 1. Concurrent programming is realized through goroutine and channel, which is lightweight and efficient. 2. The compilation speed is fast and the operation performance is close to that of C language. 3. The grammar is concise, the learning curve is smooth, and the ecosystem is rich.
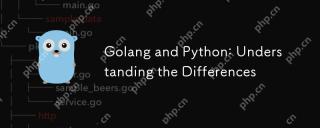
The main differences between Golang and Python are concurrency models, type systems, performance and execution speed. 1. Golang uses the CSP model, which is suitable for high concurrent tasks; Python relies on multi-threading and GIL, which is suitable for I/O-intensive tasks. 2. Golang is a static type, and Python is a dynamic type. 3. Golang compiled language execution speed is fast, and Python interpreted language development is fast.
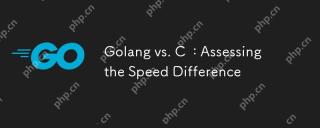
Golang is usually slower than C, but Golang has more advantages in concurrent programming and development efficiency: 1) Golang's garbage collection and concurrency model makes it perform well in high concurrency scenarios; 2) C obtains higher performance through manual memory management and hardware optimization, but has higher development complexity.
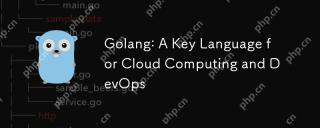
Golang is widely used in cloud computing and DevOps, and its advantages lie in simplicity, efficiency and concurrent programming capabilities. 1) In cloud computing, Golang efficiently handles concurrent requests through goroutine and channel mechanisms. 2) In DevOps, Golang's fast compilation and cross-platform features make it the first choice for automation tools.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use
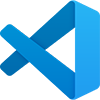
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
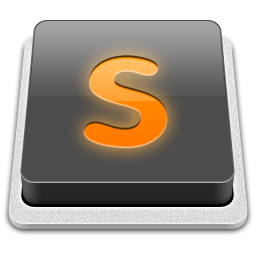
SublimeText3 Mac version
God-level code editing software (SublimeText3)