


PHP is a scripting language widely used in web development. In PHP, array is a very important data type that can be used to store multiple values. In PHP, sometimes you need to determine whether an array contains a certain element. This article will introduce some methods for determining whether a PHP array contains elements.
- in_array() function
The in_array() function is used to determine whether a value appears in the array. The syntax is as follows:
in_array($value, $array)
Among them, $value is the value to be found, and $array is the array to be found. Returns TRUE if $value appears in $array, otherwise returns FALSE.
The following is an example:
$array = array('apple', 'pear', 'banana'); if (in_array('apple', $array)) { echo 'apple is in the array'; } else { echo 'apple is not in the array'; }
In this example, $array contains 3 elements: 'apple', 'pear' and 'banana'. The code determines whether 'apple' is in $array, and the result is TRUE, so 'apple is in the array' will be output.
- array_search() function
array_search() function is used to find a value in an array and return the key name where the value is located. The syntax is as follows:
array_search($value, $array)
Among them, $value is the value to be found, and $array is the array to be found. If $value appears in $array, return the key name where $value is located, otherwise return FALSE.
The following is an example:
$array = array('apple', 'pear', 'banana'); $key = array_search('apple', $array); if ($key !== false) { echo 'apple is in the array, and its key is ' . $key; } else { echo 'apple is not in the array'; }
In this example, $array contains 3 elements: 'apple', 'pear' and 'banana'. The code uses the array_search() function to find the key name of 'apple' in $array. The result is 0, so 'apple is in the array, and its key is 0' will be output.
It should be noted that the array_search() function will consider the data type of the element when searching. This means that if the value you are looking for is equal to an element in the array but has a different data type, FALSE will also be returned. For example:
$array = array('1', 1, true); $key1 = array_search('1', $array); // 返回0 $key2 = array_search(1, $array); // 返回1 $key3 = array_search(true, $array); // 返回2 $key4 = array_search('true', $array); // 返回FALSE
In the above example, $array contains 3 elements: '1', 1 and true. The code uses the array_search() function to find the key names of '1', 1, true and 'true' in $array respectively, and the results are 0, 1, 2 and FALSE respectively.
- isset() function
isset() function is used to determine whether a variable exists and is not NULL. The syntax is as follows:
isset($variable)
Among them, $variable is the variable to be judged. Returns TRUE if $variable exists and is not NULL, otherwise returns FALSE.
In PHP, you can use the isset() function to determine whether a key in an array exists. For example:
$array = array('apple' => 1, 'pear' => 2, 'banana' => 3); if (isset($array['apple'])) { echo 'apple is in the array'; } else { echo 'apple is not in the array'; }
In this example, $array contains 3 elements, each element has both a key and a value. The code uses the isset() function to determine whether the key 'apple' in $array exists. The result is TRUE, so 'apple is in the array' will be output.
- array_key_exists() function
array_key_exists() function is used to determine whether a specified key exists in an array. The syntax is as follows:
array_key_exists($key, $array)
Among them, $key is the key name to be found, and $array is the array to be found. Returns TRUE if $key exists in $array, otherwise returns FALSE.
For example:
$array = array('apple' => 1, 'pear' => 2, 'banana' => 3); if (array_key_exists('apple', $array)) { echo 'apple is in the array'; } else { echo 'apple is not in the array'; }
In this example, $array contains 3 elements, each element has both a key and a value. The code uses the array_key_exists() function to determine whether 'apple' has a key name in $array. The result is TRUE, so 'apple is in the array' will be output.
It should be noted that the searches of the in_array() function, array_search() function, isset() function and array_key_exists() function are all case-sensitive.
Summary
The above are several ways to determine whether an array contains elements in PHP. In actual development, appropriate methods can be selected for judgment based on specific circumstances.
The above is the detailed content of How to determine whether an array contains specified elements in PHP (A brief analysis of the method). For more information, please follow other related articles on the PHP Chinese website!
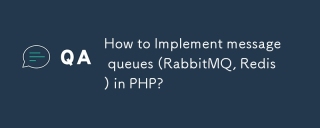
This article details implementing message queues in PHP using RabbitMQ and Redis. It compares their architectures (AMQP vs. in-memory), features, and reliability mechanisms (confirmations, transactions, persistence). Best practices for design, error
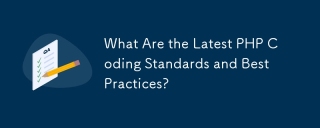
This article examines current PHP coding standards and best practices, focusing on PSR recommendations (PSR-1, PSR-2, PSR-4, PSR-12). It emphasizes improving code readability and maintainability through consistent styling, meaningful naming, and eff
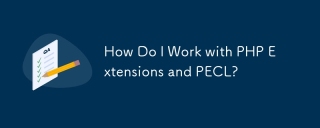
This article details installing and troubleshooting PHP extensions, focusing on PECL. It covers installation steps (finding, downloading/compiling, enabling, restarting the server), troubleshooting techniques (checking logs, verifying installation,
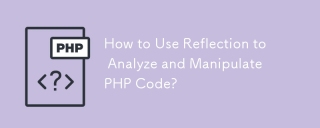
This article explains PHP's Reflection API, enabling runtime inspection and manipulation of classes, methods, and properties. It details common use cases (documentation generation, ORMs, dependency injection) and cautions against performance overhea
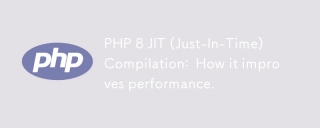
PHP 8's JIT compilation enhances performance by compiling frequently executed code into machine code, benefiting applications with heavy computations and reducing execution times.
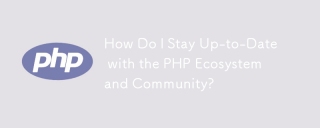
This article explores strategies for staying current in the PHP ecosystem. It emphasizes utilizing official channels, community forums, conferences, and open-source contributions. The author highlights best resources for learning new features and a
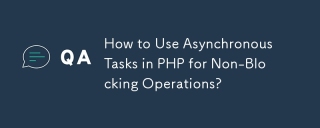
This article explores asynchronous task execution in PHP to enhance web application responsiveness. It details methods like message queues, asynchronous frameworks (ReactPHP, Swoole), and background processes, emphasizing best practices for efficien
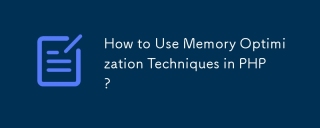
This article addresses PHP memory optimization. It details techniques like using appropriate data structures, avoiding unnecessary object creation, and employing efficient algorithms. Common memory leak sources (e.g., unclosed connections, global v


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
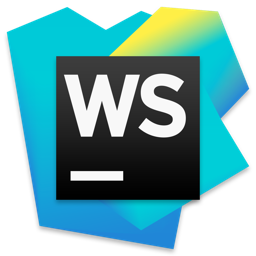
WebStorm Mac version
Useful JavaScript development tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
