PHP is one of the popular programming languages. It is inevitable that some exceptions will occur during the development process. These exceptions may cause the program to crash or run into problems, affecting the stability and reliability of the system. This article will introduce solutions to PHP exception codes to help developers better solve PHP exception problems.
- Exception overview
In PHP, exception means that when an error or unexpected situation occurs during the running of the program, the outside world can be notified by throwing an exception. So that the program can run more safely and stably. When an exception is thrown, the program will handle the exception first to avoid program crashes or running problems.
- Exception handling
Exception handling in PHP is divided into two methods: custom exception handling and system default exception handling. Custom exception handling means that developers define the circumstances and handling methods of throwing exceptions by themselves, while system default exception handling refers to the PHP system's built-in handling method. During development, we can choose to customize or use the system default exception handling as needed.
2.1 Custom exception handling
Custom exception handling is where the developer defines the circumstances and handling methods for throwing exceptions. By customizing exception handling, exceptions can be flexibly handled according to the actual situation and improve the reliability and stability of the program.
The following is an example of using custom exception handling:
try { //检查数据库连接是否为空 if(!$db_conn) { throw new Exception("数据库连接失败"); } } catch(Exception $e) { //处理异常 echo $e->getMessage(); }
In the above code, if the database connection fails, an exception will be thrown and an error message will be output. By catching exceptions, error situations can be better handled and the normal operation of the program can be ensured.
2.2 System default exception handling
System default exception handling refers to the built-in exception handling method of the PHP system. The PHP system has many built-in exception classes for developers to use, such as RuntimeException, InvalidArgumentException, LogicException, etc. These exception classes can help developers better handle errors and exceptions.
The following is an example of using the system default exception handling:
try { //解析字符串 parse_str("name=Tom&age=18", $output); if(empty($output)) { throw new InvalidArgumentException("参数解析错误"); } } catch(InvalidArgumentException $e) { //处理异常 echo $e->getMessage(); } catch(Exception $e) { //处理其他异常 echo $e->getMessage(); }
In the above code, if an error occurs in parameter parsing, an invalid parameter exception will be thrown, through different types of exception classes to handle different exception situations.
- PHP exception debugging
In development, PHP exception problems cannot be avoided. How to quickly locate and solve abnormal problems is particularly important. PHP provides some debugging tools to help developers better troubleshoot and handle exception problems.
3.1 Error report settings
In PHP, we can set the error level and error reporting method by modifying the php.ini file. These settings can help developers better view and locate anomalies.
display_errors = On //打开错误输出 error_reporting = E_ALL //输出所有错误级别信息
3.2 Log
PHP’s Log log can record all exception information in the PHP program, such as error codes, error files, error line numbers, etc. Developers can quickly locate the cause of errors and resolve abnormal situations through Log logs.
ini_set("log_errors", 1); ini_set("error_log", "/tmp/php-error.log");
In the above code, all PHP error messages are output by setting the error log path.
3.3 xdebug debugging tool
xdebug is a powerful debugging tool that can help developers better debug PHP programs. It provides functions similar to breakpoint debugging, which can quickly locate and solve abnormal problems.
- Summary
During the PHP development process, abnormal situations cannot be avoided. Understanding the use of PHP exception handling and debugging tools can help us better solve exception problems and improve the reliability and stability of the program.
The above is the detailed content of How to solve php exception code? (A brief analysis of the method). For more information, please follow other related articles on the PHP Chinese website!
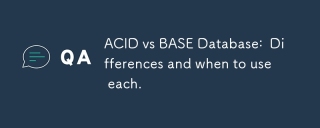
The article compares ACID and BASE database models, detailing their characteristics and appropriate use cases. ACID prioritizes data integrity and consistency, suitable for financial and e-commerce applications, while BASE focuses on availability and
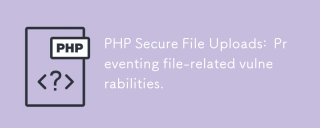
The article discusses securing PHP file uploads to prevent vulnerabilities like code injection. It focuses on file type validation, secure storage, and error handling to enhance application security.
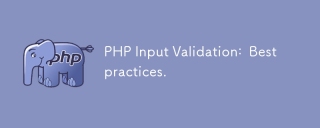
Article discusses best practices for PHP input validation to enhance security, focusing on techniques like using built-in functions, whitelist approach, and server-side validation.
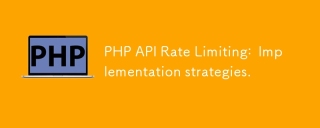
The article discusses strategies for implementing API rate limiting in PHP, including algorithms like Token Bucket and Leaky Bucket, and using libraries like symfony/rate-limiter. It also covers monitoring, dynamically adjusting rate limits, and hand
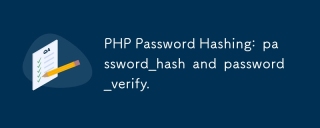
The article discusses the benefits of using password_hash and password_verify in PHP for securing passwords. The main argument is that these functions enhance password protection through automatic salt generation, strong hashing algorithms, and secur
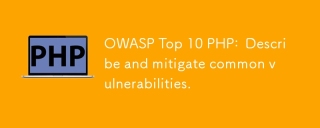
The article discusses OWASP Top 10 vulnerabilities in PHP and mitigation strategies. Key issues include injection, broken authentication, and XSS, with recommended tools for monitoring and securing PHP applications.
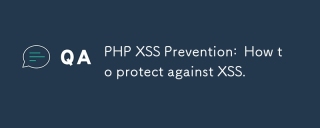
The article discusses strategies to prevent XSS attacks in PHP, focusing on input sanitization, output encoding, and using security-enhancing libraries and frameworks.

The article discusses the use of interfaces and abstract classes in PHP, focusing on when to use each. Interfaces define a contract without implementation, suitable for unrelated classes and multiple inheritance. Abstract classes provide common funct


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
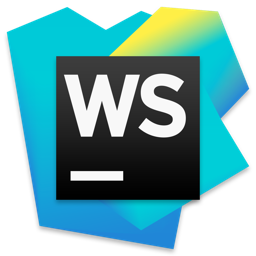
WebStorm Mac version
Useful JavaScript development tools
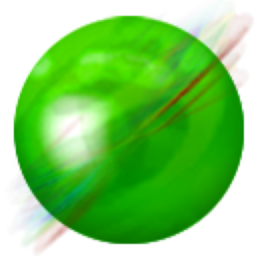
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.