As PHP becomes the mainstream language for modern web development, more and more developers are beginning to get involved in object programming. In PHP, objects are a very powerful and common data structure. However, in actual development, we often need to convert objects into string or array objects in order to perform other operations. This article will introduce how to perform object conversion in PHP, including string conversion, array conversion, and object array conversion.
1. Convert PHP objects to strings
In PHP, converting objects to strings is very simple. You only need to define a __toString() magic method in the object, which will automatically convert the object to a string when called. For example, the following sample code:
class Person{ public $name; public $age; public function __construct($name,$age){ $this->name = $name; $this->age = $age; } public function __toString(){ return $this->name . " is " . $this->age . " years old."; } } $person = new Person("Tom",20); echo $person;
The running result is:
Tom is 20 years old.
In the above sample code, we define a Person class, which has two attributes $name and $age, Indicate the person's name and age respectively. We also define a __toString() method, which automatically converts the object to a string to achieve object conversion.
2. Convert PHP objects to arrays
In addition to converting objects to strings, we can also convert objects to arrays. PHP provides two methods to convert objects into arrays. One is to use the get_object_vars() function, which can return a list of object attributes. The other method is to use the object attribute accessor "->" to obtain the object attribute value. As shown in the following sample code:
class Person{ public $name; public $age; public function __construct($name,$age){ $this->name = $name; $this->age = $age; } public function toArray(){ return get_object_vars($this); } } $person = new Person("Tom",20); print_r($person->toArray());
The running result is:
Array ( [name] => Tom [age] => 20 )
In the above sample code, we define a Person class, which has two attributes $name and $ Age represents the person's name and age respectively. We also define a toArray() method, which uses the get_object_vars() function to return a list of object properties to convert the object into an array.
In addition to using the get_object_vars() function, we can also use the object attribute accessor "->" to obtain the object attribute value, thereby converting the object into an array. As shown below:
class Person{ public $name; public $age; public function __construct($name,$age){ $this->name = $name; $this->age = $age; } public function toArray(){ return array( "name" => $this->name, "age" => $this->age ); } } $person = new Person("Tom",20); print_r($person->toArray());
The running result is:
Array ( [name] => Tom [age] => 20 )
In the above example code, we define a Person class, which has two attributes $name and $age, representing respectively Name and age of the person. We also define a toArray() method, which uses the object property accessor "->" to obtain the object property value and returns the property array, thereby converting the object into an array.
3. Convert PHP object array to array
In actual development, we often need to convert multiple objects into arrays and form these arrays into an array object. PHP provides two methods to achieve this function, one is to use array traversal, and the other is to use array mapping. As shown in the following sample code:
class Person{ public $name; public $age; public function __construct($name,$age){ $this->name = $name; $this->age = $age; } public function toArray(){ return array( "name" => $this->name, "age" => $this->age ); } } $persons = array( new Person("Tom",20), new Person("Jerry",25), new Person("Kate",30) ); //数组遍历 $result = array(); foreach($persons as $person){ $result[] = $person->toArray(); } print_r($result); //数组映射 $result = array_map(function($person){ return $person->toArray(); },$persons); print_r($result);
The running result is:
Array ( [0] => Array ( [name] => Tom [age] => 20 ) [1] => Array ( [name] => Jerry [age] => 25 ) [2] => Array ( [name] => Kate [age] => 30 ) ) Array ( [0] => Array ( [name] => Tom [age] => 20 ) [1] => Array ( [name] => Jerry [age] => 25 ) [2] => Array ( [name] => Kate [age] => 30 ) )
In the above sample code, we define a Person class, which has two attributes $name and $ Age represents the person's name and age respectively. We also define a toArray() method, which uses the object property accessor "->" to obtain the object property value and returns the property array, thereby converting the object into an array.
We define an array $persons containing multiple Person objects. Using array traversal or array mapping, we can convert each Person object into an array and then form an array object. The end result is an array containing multiple array objects.
Summary:
PHP provides a variety of methods to achieve object conversion, including string conversion, array conversion, and object array conversion. We can choose the appropriate method according to actual needs to realize the object conversion function.
The above is the detailed content of How to convert objects in PHP. For more information, please follow other related articles on the PHP Chinese website!
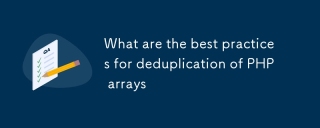
This article explores efficient PHP array deduplication. It compares built-in functions like array_unique() with custom hashmap approaches, highlighting performance trade-offs based on array size and data type. The optimal method depends on profili
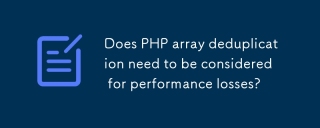
This article analyzes PHP array deduplication, highlighting performance bottlenecks of naive approaches (O(n²)). It explores efficient alternatives using array_unique() with custom functions, SplObjectStorage, and HashSet implementations, achieving
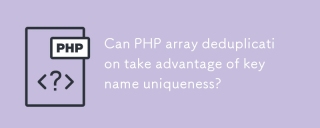
This article explores PHP array deduplication using key uniqueness. While not a direct duplicate removal method, leveraging key uniqueness allows for creating a new array with unique values by mapping values to keys, overwriting duplicates. This ap
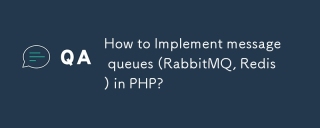
This article details implementing message queues in PHP using RabbitMQ and Redis. It compares their architectures (AMQP vs. in-memory), features, and reliability mechanisms (confirmations, transactions, persistence). Best practices for design, error
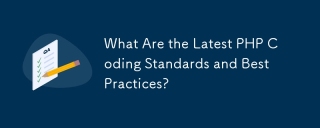
This article examines current PHP coding standards and best practices, focusing on PSR recommendations (PSR-1, PSR-2, PSR-4, PSR-12). It emphasizes improving code readability and maintainability through consistent styling, meaningful naming, and eff
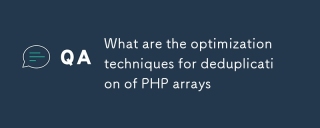
This article explores optimizing PHP array deduplication for large datasets. It examines techniques like array_unique(), array_flip(), SplObjectStorage, and pre-sorting, comparing their efficiency. For massive datasets, it suggests chunking, datab
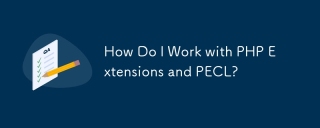
This article details installing and troubleshooting PHP extensions, focusing on PECL. It covers installation steps (finding, downloading/compiling, enabling, restarting the server), troubleshooting techniques (checking logs, verifying installation,
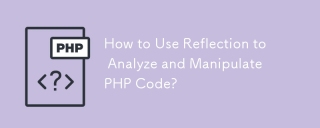
This article explains PHP's Reflection API, enabling runtime inspection and manipulation of classes, methods, and properties. It details common use cases (documentation generation, ORMs, dependency injection) and cautions against performance overhea


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

SublimeText3 English version
Recommended: Win version, supports code prompts!
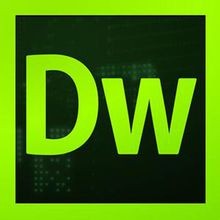
Dreamweaver CS6
Visual web development tools
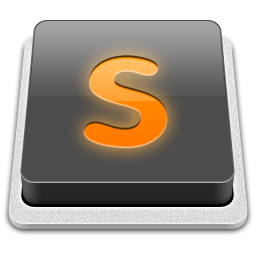
SublimeText3 Mac version
God-level code editing software (SublimeText3)
