ThinkPHP is a mature PHP development framework that can help developers quickly build stable and efficient applications. During development, obtaining object parameters is a common operation. This article will introduce how to obtain object parameters in ThinkPHP.
1. Obtaining parameters through the request object
In ThinkPHP, the most common way to obtain request parameters is through the request object. The request object is a system-level object that can obtain parameters of request methods such as GET, POST, PUT, and DELETE, as well as data such as uploaded files.
The method of using the request object to obtain parameters is as follows:
//获取GET方式的参数 $request->get('paramName'); //获取POST方式的参数 $request->post('paramName'); //获取PUT方式的参数 $input = file_get_contents('php://input'); parse_str($input, $data); $request->put($data); //获取DELETE方式的参数 $input = file_get_contents('php://input'); parse_str($input, $data); $request->delete($data);
Among them, $paramName is the name of the parameter, which can be modified according to the specific situation. When using PUT and DELETE to obtain parameters, the original data needs to be parsed into an array and then obtained through the request object.
2. Obtain parameters through the controller object
In the controller, you can directly use the $this object to obtain the request object and obtain the request parameters through the request object. For example:
class UserController extends Controller { public function index() { $param = $this->request->param('id'); echo $param; } }
In this example, what is obtained in $param is the value of the parameter named id passed in GET mode.
3. Obtain parameters through the model object
In the model, you can obtain the controller object through the $this object, and then obtain the request parameters through the controller object. For example:
class User extends Model { public function getInfo() { $param = $this->controller->request->param('id'); echo $param; } }
In this example, what is obtained in $param is the value of the parameter named id passed in GET mode.
Summary:
Getting object parameters is a common operation. ThinkPHP provides us with a variety of methods to get request parameters. We can choose the appropriate method to obtain parameters according to the specific situation, making the code more concise and efficient.
The above is the detailed content of Let's talk about how to obtain object parameters in ThinkPHP. For more information, please follow other related articles on the PHP Chinese website!
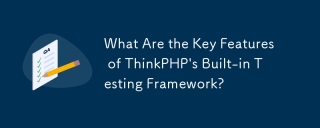
The article discusses ThinkPHP's built-in testing framework, highlighting its key features like unit and integration testing, and how it enhances application reliability through early bug detection and improved code quality.
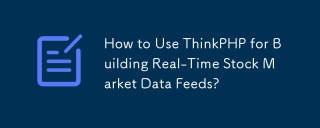
Article discusses using ThinkPHP for real-time stock market data feeds, focusing on setup, data accuracy, optimization, and security measures.

The article discusses key considerations for using ThinkPHP in serverless architectures, focusing on performance optimization, stateless design, and security. It highlights benefits like cost efficiency and scalability, but also addresses challenges
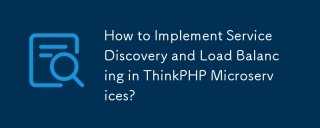
The article discusses implementing service discovery and load balancing in ThinkPHP microservices, focusing on setup, best practices, integration methods, and recommended tools.[159 characters]
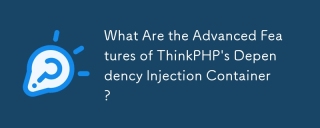
ThinkPHP's IoC container offers advanced features like lazy loading, contextual binding, and method injection for efficient dependency management in PHP apps.Character count: 159
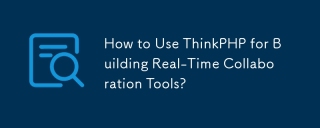
The article discusses using ThinkPHP to build real-time collaboration tools, focusing on setup, WebSocket integration, and security best practices.
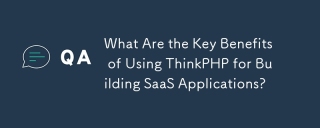
ThinkPHP benefits SaaS apps with its lightweight design, MVC architecture, and extensibility. It enhances scalability, speeds development, and improves security through various features.
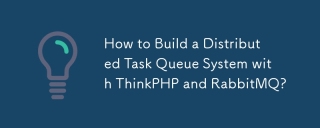
The article outlines building a distributed task queue system using ThinkPHP and RabbitMQ, focusing on installation, configuration, task management, and scalability. Key issues include ensuring high availability, avoiding common pitfalls like imprope


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use

Atom editor mac version download
The most popular open source editor
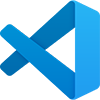
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

Zend Studio 13.0.1
Powerful PHP integrated development environment

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software