In web development, tags generally refer to keywords in a certain piece of content. Using tags can help users better classify and retrieve information. When a tag is widely used, there is a need to query the content related to the tag. So how to implement the tag query function when using the thinkphp framework?
First, you need to create a tag table (tag) in the database, which contains tag id, tag name (tag_name), tag creation time (create_time) and other fields. In scenarios where tags need to be queried, we need to pass a tag name (tag_name) parameter. Here is a method of using thinkphp to query tags:
① Define a Tag model class
Create a new Tag.php file in the application\common\model directory. This file is used to define the Tag model class and inherits the think\Model class. The basic information and operation methods of the model are defined in the model class. The specific code is as follows:
<?php namespace app\common\model; use think\Model; class Tag extends Model { protected $name = 'tag';//指定表名 }
② Write the index method of the Tag controller
Create a new Tag.php in the application\index\controller directory file, which is used to define the Tag controller class and write the index method in this class to query tags. In the index method, the tag_name parameter passed by the front desk is accepted, and the tag data is queried using the where conditional statement and the select method. The specific code is as follows:
<?php namespace app\index\controller; use app\common\model\Tag; class Tag { public function index($tag_name) { //实例化模型类 $tagModel = new Tag(); //查询标签数据 $tagList = $tagModel->where('tag_name', '=', $tag_name)->select(); //返回查询结果 return json($tagList); } }
③ Define the route
Define the route in the route directory of the project, and map tag/:tag_name to the index method of the Tag controller. The specific code is as follows:
use think\Route; Route::get('tag/:tag_name', 'index/Tag/index');
Through the above steps, you can realize the function of querying tag data by passing the tag name. By using the ORM (Object Relational Mapping) function and powerful routing function provided by the thinkphp framework, you can quickly and easily implement data addition, deletion, modification and query operations, which greatly improves web development efficiency and development experience.
The above is the detailed content of How to implement the query tag function in thinkphp. For more information, please follow other related articles on the PHP Chinese website!
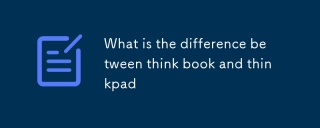
This article compares Lenovo's ThinkBook and ThinkPad laptop lines. ThinkPads prioritize durability and performance for professionals, while ThinkBooks offer a stylish, affordable option for everyday use. The key differences lie in build quality, p
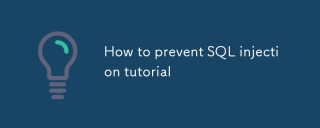
This article explains how to prevent SQL injection in ThinkPHP applications. It emphasizes using parameterized queries via ThinkPHP's query builder, avoiding direct SQL concatenation, and implementing robust input validation & sanitization. Ad
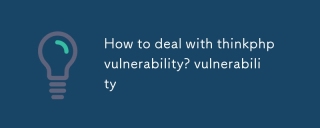
This article addresses ThinkPHP vulnerabilities, emphasizing patching, prevention, and monitoring. It details handling specific vulnerabilities via updates, security patches, and code remediation. Proactive measures like secure configuration, input
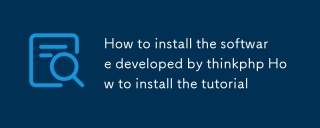
This article details ThinkPHP software installation, covering steps like downloading, extraction, database configuration, and permission verification. It addresses system requirements (PHP version, web server, database, extensions), common installat

This tutorial addresses common ThinkPHP vulnerabilities. It emphasizes regular updates, security scanners (RIPS, SonarQube, Snyk), manual code review, and penetration testing for identification and remediation. Preventative measures include secure

This guide details database connection in ThinkPHP, focusing on configuration via database.php. It uses PDO and allows for ORM or direct SQL interaction. The guide covers troubleshooting common connection errors, managing multiple connections, en
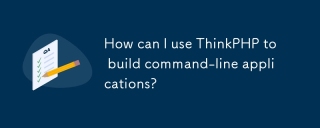
This article demonstrates building command-line applications (CLIs) using ThinkPHP's CLI capabilities. It emphasizes best practices like modular design, dependency injection, and robust error handling, while highlighting common pitfalls such as insu
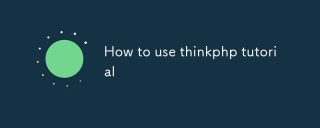
This article introduces ThinkPHP, a free, open-source PHP framework. It details ThinkPHP's MVC architecture, features (routing, database interaction), advantages (rapid development, ease of use), and disadvantages (potential over-engineering, commun


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
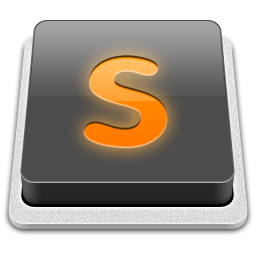
SublimeText3 Mac version
God-level code editing software (SublimeText3)

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

Zend Studio 13.0.1
Powerful PHP integrated development environment
