Laravel is a popular PHP framework. After years of development, it has become one of the preferred frameworks for PHP developers. It has very powerful routing functions. This article will introduce how to write routes in Laravel.
In Laravel, all routes are defined in the routes/web.php
file. The routes defined in this file are the main routes for the web application.
Laravel's routing is divided into two methods: GET and POST routing. The GET route is used to obtain data, and the POST route is used to send data to the server. The following describes how to define GET and POST routes in Laravel.
How to define GET route
In the routes/web.php
file, use the following code to define the GET route:
Route::get('/', function () { return view('welcome'); });
The above code defines A route that returns the welcome
view when the user accesses the project root path. In this example, /
represents the project root path, and function () {}
is an anonymous function that will be executed when the route is accessed.
You can also specify methods in the Controller:
Route::get('/users', 'UserController@index');
The above code defines a users
route. When the user accesses the route, Laravel will call UserController
The index
method in the controller. Methods in the Controller can perform more complex operations, such as retrieving data from the database and displaying it in the view.
How to define POST routing
In the routes/web.php
file, use the following code to define the POST route:
Route::post('/users', function (Request $request) { $name = $request->input('name'); $email = $request->input('email'); // 保存到数据库中 return view('users'); });
The above code defines A users
route. When a user sends a POST request to this route, Laravel will execute the defined anonymous function. This function uses an instance of the Request
class to receive the data from the POST request and then saves the data to the database.
In addition to GET and POST, there are other HTTP request methods, such as PUT, DELETE, etc. Laravel supports all HTTP request methods. The following is an example of using a PUT request:
Route::put('/users/{id}', function ($id) { // 根据ID更新用户 });
The above code defines a users
route. When the user accesses this route using the PUT request method, Laravel will execute the defined anonymous function. This function receives a $id
parameter, which is used to indicate that the user's ID is to be updated.
In Laravel, routing also supports parameters and route grouping. Here is an example of using parameters and route grouping:
Route::group(['middleware' => 'auth'], function () { Route::get('/dashboard', 'DashboardController@show'); Route::get('/users/{id}', 'UserController@show'); });
The above code defines a dashboard
route and a users
route, both routes are in Under the protection of auth
middleware. The DashboardController@show
method will display the dashboard view, and the UserController@show
method will display the user information based on the passed in $id
parameter.
Conclusion
Laravel's routing function is very powerful. It can easily define all types of routes and provides many convenient functions, such as routing parameters, route grouping, middleware, etc. When you start using Laravel, be sure to understand these routing knowledge to better use this powerful PHP framework.
The above is the detailed content of Detailed code explanation of routing in Laravel. For more information, please follow other related articles on the PHP Chinese website!

Laravel is suitable for building web applications quickly, while Python is suitable for a wider range of application scenarios. 1.Laravel provides EloquentORM, Blade template engine and Artisan tools to simplify web development. 2. Python is known for its dynamic types, rich standard library and third-party ecosystem, and is suitable for Web development, data science and other fields.

Laravel and Python each have their own advantages: Laravel is suitable for quickly building feature-rich web applications, and Python performs well in the fields of data science and general programming. 1.Laravel provides EloquentORM and Blade template engines, suitable for building modern web applications. 2. Python has a rich standard library and third-party library, and Django and Flask frameworks meet different development needs.

Laravel is worth choosing because it can make the code structure clear and the development process more artistic. 1) Laravel is based on PHP, follows the MVC architecture, and simplifies web development. 2) Its core functions such as EloquentORM, Artisan tools and Blade templates enhance the elegance and robustness of development. 3) Through routing, controllers, models and views, developers can efficiently build applications. 4) Advanced functions such as queue and event monitoring further improve application performance.

Laravel is not only a back-end framework, but also a complete web development solution. It provides powerful back-end functions, such as routing, database operations, user authentication, etc., and supports front-end development, improving the development efficiency of the entire web application.

Laravel is suitable for web development, Python is suitable for data science and rapid prototyping. 1.Laravel is based on PHP and provides elegant syntax and rich functions, such as EloquentORM. 2. Python is known for its simplicity, widely used in Web development and data science, and has a rich library ecosystem.

Laravelcanbeeffectivelyusedinreal-worldapplicationsforbuildingscalablewebsolutions.1)ItsimplifiesCRUDoperationsinRESTfulAPIsusingEloquentORM.2)Laravel'secosystem,includingtoolslikeNova,enhancesdevelopment.3)Itaddressesperformancewithcachingsystems,en

Laravel's core functions in back-end development include routing system, EloquentORM, migration function, cache system and queue system. 1. The routing system simplifies URL mapping and improves code organization and maintenance. 2.EloquentORM provides object-oriented data operations to improve development efficiency. 3. The migration function manages the database structure through version control to ensure consistency. 4. The cache system reduces database queries and improves response speed. 5. The queue system effectively processes large-scale data, avoid blocking user requests, and improve overall performance.

Laravel performs strongly in back-end development, simplifying database operations through EloquentORM, controllers and service classes handle business logic, and providing queues, events and other functions. 1) EloquentORM maps database tables through the model to simplify query. 2) Business logic is processed in controllers and service classes to improve modularity and maintainability. 3) Other functions such as queue systems help to handle complex needs.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
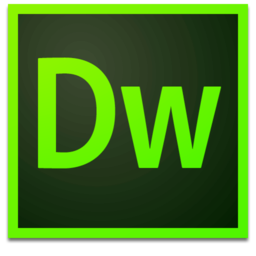
Dreamweaver Mac version
Visual web development tools
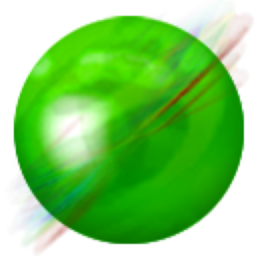
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment