Golang is a relatively young programming language, but thanks to its extremely high operating efficiency and strong concurrent processing capabilities, it is increasingly favored by developers. In actual development, we often need to convert time into strings in different formats, or convert strings into time. In this article, we will share how to efficiently implement time conversion in Golang.
- Time type
In Golang, there are two main time types: time.Time and time.Duration. Among them, time.Time represents a time point, which can be used to represent a specific time, such as 12 o'clock on July 1, 2021, and time.Duration represents a time period, which can be used to represent the time span between a certain time point. Such as 10 seconds, 5 minutes, etc.
- Formatted output of time
If you need to convert a time of type time.Time into a string, you can use Format() in type time.Time method. The Format() method receives a time format layout as an input parameter, and then formats the time into a corresponding string.
The following is a sample code:
package main import ( "fmt" "time" ) func main() { t := time.Now() fmt.Println(t.Format("2006-01-02 15:04:05")) }
Running result:
2021-07-01 12:00:00
In the format string, different characters represent different meanings, as shown in the following table:
Format characters | Meaning |
---|---|
年( Year used in the example) | |
month | |
日 | |
hours (24-hour format) | |
hours (12-hour format) | |
Minutes | |
Seconds | |
Milliseconds | |
AM or PM (12-hour clock) | |
Day of the week (full name, such as Monday) | |
Day of the week (abbreviated name, such as Mon) | |
Month (full name, such as January) | |
Month (abbreviated name, such as Jan) | |
Time zone name (such as MST, PST, etc.) |
t := time.Now() fmt.Println(t.Format("2006年01月02日 15:04:05.000 PM MST"))Convert string to time type
- If you need to convert a string into a time of type time.Time, you can use time.Parse( )method. The Parse() method receives two parameters, the first parameter is the time string, and the second parameter is the time format layout. For example:
package main import ( "fmt" "time" ) func main() { str := "2021-07-01 12:00:00" layout := "2006-01-02 15:04:05" t, err := time.Parse(layout, str) if err != nil { fmt.Println(err) } fmt.Println(t) }
In the above code, we converted the string into a time.Time type variable t, and determined errors in the conversion process in the code to ensure the robustness of the program.
Calculate the difference in time- In Golang, we can directly use subtraction to calculate the difference in time, for example:
package main import ( "fmt" "time" ) func main() { t1 := time.Date(2021, 7, 1, 12, 0, 0, 0, time.Local) t2 := time.Date(2021, 7, 1, 12, 1, 0, 0, time.Local) duration := t2.Sub(t1) fmt.Println(duration) }
In the above code, we use the time.Date() function to create two times t1 and t2, calculate their time difference, and save the result to a variable duration of type time.Duration.
Convert timestamp to time.Time type- In programs, timestamps are sometimes used to represent time. In Golang, time.Unix() can be used Method converts the timestamp into a time of type time.Time. For example:
package main import ( "fmt" "time" ) func main() { timestamp := int64(1625133600) t := time.Unix(timestamp, 0) fmt.Println(t) }
Run result:
2021-07-01 13:00:00 +0800 CST
In the above code, we use time. The Unix() method converts a time t of type time.Time.
Notes on time operations- In the process of using Golang for time operations, you need to pay attention to the following points:
1) When using time When the .Parse() method converts a string into a time of type time.Time, it must ensure that the time string matches the format of the time format layout, otherwise an error will occur.
2) When we need to perform time zone conversion when processing time, we must use the two variables time.UTC() and time.Local. time.UTC() represents Coordinated Universal Time, and time.Local represents The current time zone of the system.
3) When processing time, when you need to obtain the separate parts of the time such as the year, month, day, hour, minute, and second, you can use Year(), Month(), Day(), Hour( in the time.Time type ), Minute(), Second() and other methods.
4) When using a time.Duration type variable to represent a time span, you can use the time.ParseDuration() method to convert the string type time span into a time.Duration type variable and control the accuracy. .
In short, when using Golang to process time, you need to pay attention to details to ensure the accuracy of the time and the robustness of the program.
Summary- In this article, we discussed how to perform time conversion operations in Golang, including time formatted output, string conversion to time.Time type, Knowledge points on calculating time differences, converting timestamps into time.Time types, etc. By mastering this knowledge, we can help us deal with time-related issues more efficiently and improve the practicality and reliability of the program.
The above is the detailed content of How to efficiently implement time conversion in Golang. For more information, please follow other related articles on the PHP Chinese website!
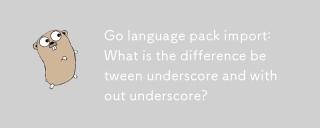
This article explains Go's package import mechanisms: named imports (e.g., import "fmt") and blank imports (e.g., import _ "fmt"). Named imports make package contents accessible, while blank imports only execute t

This article explains Beego's NewFlash() function for inter-page data transfer in web applications. It focuses on using NewFlash() to display temporary messages (success, error, warning) between controllers, leveraging the session mechanism. Limita
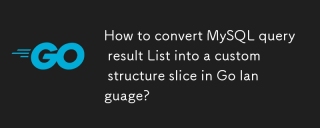
This article details efficient conversion of MySQL query results into Go struct slices. It emphasizes using database/sql's Scan method for optimal performance, avoiding manual parsing. Best practices for struct field mapping using db tags and robus
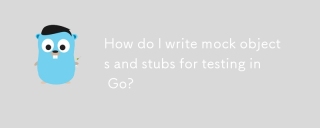
This article demonstrates creating mocks and stubs in Go for unit testing. It emphasizes using interfaces, provides examples of mock implementations, and discusses best practices like keeping mocks focused and using assertion libraries. The articl
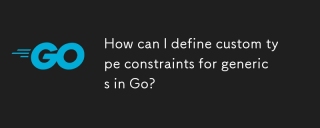
This article explores Go's custom type constraints for generics. It details how interfaces define minimum type requirements for generic functions, improving type safety and code reusability. The article also discusses limitations and best practices

This article details efficient file writing in Go, comparing os.WriteFile (suitable for small files) with os.OpenFile and buffered writes (optimal for large files). It emphasizes robust error handling, using defer, and checking for specific errors.
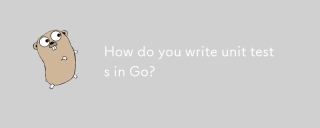
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
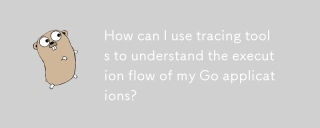
This article explores using tracing tools to analyze Go application execution flow. It discusses manual and automatic instrumentation techniques, comparing tools like Jaeger, Zipkin, and OpenTelemetry, and highlighting effective data visualization


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
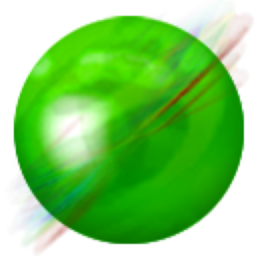
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

SublimeText3 English version
Recommended: Win version, supports code prompts!

Zend Studio 13.0.1
Powerful PHP integrated development environment
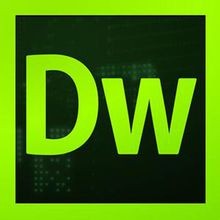
Dreamweaver CS6
Visual web development tools
