In modern web development, APIs have become an indispensable part. In order to complete data transmission and interaction from client to server, we need to send and receive HTTP requests and responses. In this article, we will use Go language to implement the process of requesting API.
Go language is an open source programming language that is very suitable for building highly concurrent, scalable and efficient web applications. In this article, we will use the standard library of the Go language or a third-party library to request the API and process the response data.
References:
- https://golang.org/pkg/net/http/
- https://github.com/go-resty/ resty
- https://golang.org/pkg/encoding/json/
- https://github.com/bitly/go-simplejson
Understand HTTP request
Before understanding how to use Go language to request API, we need to first understand how HTTP request works. HTTP requests usually consist of three parts: request line, request headers and request body.
The request line includes the requested method, URL, and HTTP version. Here, we generally use the GET method because it is the most commonly used method.
Request headers include different types of metadata. For example, we can use request headers to pass information such as authorization tokens, user agents, and cookies.
The request body usually contains the data sent to the server. However, when using a GET request, the request body is usually empty.
Use Go standard library request API
The standard library of Go language includes an HTTP package, which provides basic functions for sending HTTP requests and processing responses.
The following is an example of using the Go language standard library to request the API:
package main import ( "fmt" "net/http" ) func main() { resp, err := http.Get("https://jsonplaceholder.typicode.com/posts") if err != nil { fmt.Println("请求错误:", err) return } defer resp.Body.Close() fmt.Println("响应状态码:", resp.StatusCode) fmt.Println("响应头部:", resp.Header) }
In this example, we use http.Get() to send an HTTP GET request, passing in the request API address.
If the request is successful, we can read the content of the response body through resp.Body. Finally, we close the response body using resp.Body.Close() to prevent resource leaks.
In this example, we only output the status code and header information of the response. If we want to handle the body of the request, we need to read the contents of the response.
Processing response data
The standard library in Go language supports processing of multiple response bodies. For example, we can use the json package to process responses in JSON format, use the xml package to process responses in XML format, etc. If the response sent by the API is not in one of these data formats, we can use the io package to read the response body.
The following is an example of using the Go standard library to process JSON format responses:
package main import ( "encoding/json" "fmt" "net/http" ) type Post struct { UserId int `json:"userId"` Id int `json:"id"` Title string `json:"title"` Body string `json:"body"` } func main() { resp, err := http.Get("https://jsonplaceholder.typicode.com/posts") if err != nil { fmt.Println("请求错误:", err) return } defer resp.Body.Close() var posts []Post err = json.NewDecoder(resp.Body).Decode(&posts) if err != nil { fmt.Println("解析错误:", err) return } for _, p := range posts { fmt.Println(p) } }
In this example, we define a structure Post, which corresponds to the JSON format returned by the API. We use the json package to parse the response body and parse the JSON into a Post structure.
Note that we passed the &posts parameter because the json.NewDecoder() method requires a pointer to the parsed variable. Finally, we print all requested posts.
Use third-party libraries to request API
In addition to the standard library of Go language, there are also some third-party libraries that can simplify the process of requesting APIs. For example, the Resty library can make the request API simpler and easier to use.
The following is an example of using the Resty library to request the API:
package main import ( "fmt" "github.com/go-resty/resty" ) type Post struct { UserId int `json:"userId"` Id int `json:"id"` Title string `json:"title"` Body string `json:"body"` } func main() { client := resty.New() resp, err := client.R().Get("https://jsonplaceholder.typicode.com/posts") if err != nil { fmt.Println("请求错误:", err) return } var posts []Post err = json.Unmarshal(resp.Body(), &posts) if err != nil { fmt.Println("解析错误:", err) return } for _, p := range posts { fmt.Println(p) } }
In this example, we use the Resty library to send the request, where client.R().Get() is to send GET Requested shortcut. We use the Unmarshal() method to parse the response body and parse the JSON into a Post structure.
Unlike the Go standard library, the Resty library also supports functions such as adding request headers, passing parameters, setting proxies, etc., making API requests more flexible and convenient.
Summary
In this article, we learned how to use Go language to send API requests and process response data. We learned about the three components of HTTP requests, and how to use the Go language standard library and Resty library.
Of course, in addition to these libraries, there are many other third-party libraries that can be used. Through continuous learning and experimentation, we can find the libraries and tools that are most suitable for the current project and use them to improve development efficiency and code quality.
The above is the detailed content of Let's talk about the process of using Go language to request API. For more information, please follow other related articles on the PHP Chinese website!
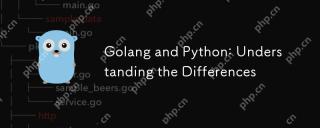
The main differences between Golang and Python are concurrency models, type systems, performance and execution speed. 1. Golang uses the CSP model, which is suitable for high concurrent tasks; Python relies on multi-threading and GIL, which is suitable for I/O-intensive tasks. 2. Golang is a static type, and Python is a dynamic type. 3. Golang compiled language execution speed is fast, and Python interpreted language development is fast.
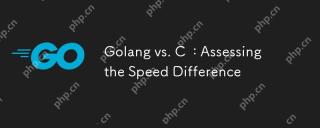
Golang is usually slower than C, but Golang has more advantages in concurrent programming and development efficiency: 1) Golang's garbage collection and concurrency model makes it perform well in high concurrency scenarios; 2) C obtains higher performance through manual memory management and hardware optimization, but has higher development complexity.
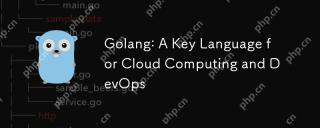
Golang is widely used in cloud computing and DevOps, and its advantages lie in simplicity, efficiency and concurrent programming capabilities. 1) In cloud computing, Golang efficiently handles concurrent requests through goroutine and channel mechanisms. 2) In DevOps, Golang's fast compilation and cross-platform features make it the first choice for automation tools.
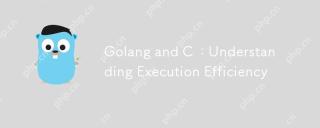
Golang and C each have their own advantages in performance efficiency. 1) Golang improves efficiency through goroutine and garbage collection, but may introduce pause time. 2) C realizes high performance through manual memory management and optimization, but developers need to deal with memory leaks and other issues. When choosing, you need to consider project requirements and team technology stack.
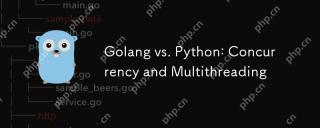
Golang is more suitable for high concurrency tasks, while Python has more advantages in flexibility. 1.Golang efficiently handles concurrency through goroutine and channel. 2. Python relies on threading and asyncio, which is affected by GIL, but provides multiple concurrency methods. The choice should be based on specific needs.
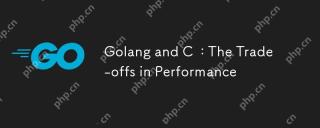
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
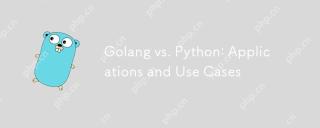
ChooseGolangforhighperformanceandconcurrency,idealforbackendservicesandnetworkprogramming;selectPythonforrapiddevelopment,datascience,andmachinelearningduetoitsversatilityandextensivelibraries.

Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
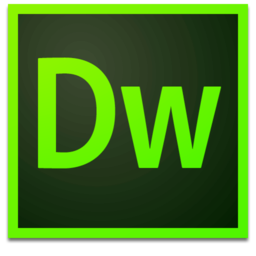
Dreamweaver Mac version
Visual web development tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
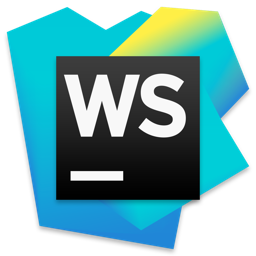
WebStorm Mac version
Useful JavaScript development tools