Laravel is a very popular web development framework currently in the PHP field. In Laravel, middleware plays a very important role. So how is Laravel's middleware implemented? This article will introduce this in detail.
1. What is middleware
Before we start introducing the implementation details of Laravel middleware, we need to clarify what middleware is. In web development, middleware refers to the components that sit between the client and server. Middleware controls client input and server output, and can preprocess and respond to requests.
In Laravel, the functions that middleware can achieve are very rich. For example, verify whether the user is logged in, set response headers, filter requests, etc. Using middleware in Laravel helps decouple request and response processing logic, making it easier to maintain the code.
2. How to implement Laravel middleware
In the Laravel framework, the implementation of middleware is very simple. You only need to inherit the Illuminate\Http\Middleware\Middleware class. The following is an example to verify whether the user is logged in:
<?php namespace App\Http\Middleware; use Closure; use Illuminate\Support\Facades\Auth; class AuthMiddleware { public function handle($request, Closure $next) { if (!Auth::check()) { return redirect('/login'); } return $next($request); } }
The above is the basic implementation of a middleware, which implements the handle method to preprocess and process requests and responses.
In Laravel middleware, the handle method is required to handle requests and responses. The handle method has two parameters. The first parameter is $request, which represents the request instance; the second parameter is $next, which represents the closure function that continues execution of the next request.
If we need to perform response processing in middleware, we can do so by returning a Response instance. For example:
<?php namespace App\Http\Middleware; use Closure; use Illuminate\Http\Response; class SetHeadersMiddleware { public function handle($request, Closure $next) { $response = $next($request); $response->headers->set('X-Powered-By', 'Laravel'); return $response; } }
The above is an example of changing the response header.
3. Using Laravel middleware
In Laravel, we can easily use middleware. Add the middleware class we wrote to the $routeMiddleware attribute, and then use it in the routing configuration. For example:
- Add the AuthMiddleware class in the $routeMiddleware attribute in the app/Http/Kernel.php file, the code is as follows:
protected $routeMiddleware = [ ... 'auth' => \App\Http\Middleware\AuthMiddleware::class, ... ];
- In routing Specify middleware, for example:
Route::get('/dashboard', function () { return view('dashboard'); })->middleware('auth');
The above code specifies that when the user accesses the /dashboard path, it needs to verify whether the user is logged in, and if not logged in, redirect to the login page.
4. Conclusion
This article briefly introduces the implementation and use of Laravel middleware. Middleware can help us decouple request and response processing logic and improve code maintainability. In the actual development process, using Laravel middleware can help us improve code reusability and scalability, and is a very recommended way.
The above is the detailed content of Detailed explanation of the implementation of Laravel middleware. For more information, please follow other related articles on the PHP Chinese website!
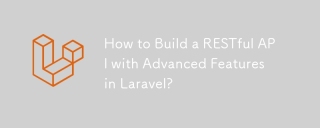
This article guides building robust Laravel RESTful APIs. It covers project setup, resource management, database interactions, serialization, authentication, authorization, testing, and crucial security best practices. Addressing scalability chall
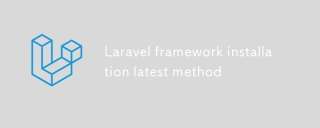
This article provides a comprehensive guide to installing the latest Laravel framework using Composer. It details prerequisites, step-by-step instructions, troubleshooting common installation issues (PHP version, extensions, permissions), and minimu
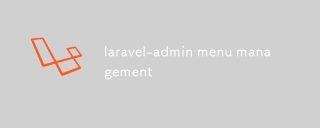
This article guides Laravel-Admin users on menu management. It covers menu customization, best practices for large menus (categorization, modularization, search), and dynamic menu generation based on user roles and permissions using Laravel's author
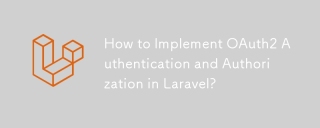
This article details implementing OAuth 2.0 authentication and authorization in Laravel. It covers using packages like league/oauth2-server or provider-specific solutions, emphasizing database setup, client registration, authorization server configu
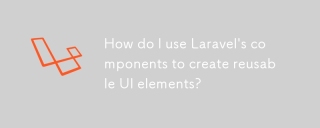
The article discusses creating and customizing reusable UI elements in Laravel using components, offering best practices for organization and suggesting enhancing packages.
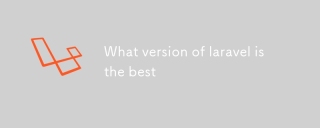
This article guides Laravel developers in choosing the right version. It emphasizes the importance of selecting the latest Long Term Support (LTS) release for stability and security, while acknowledging that newer versions offer advanced features.
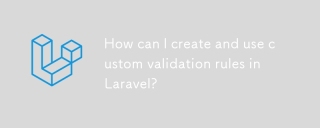
The article discusses creating and using custom validation rules in Laravel, offering steps to define and implement them. It highlights benefits like reusability and specificity, and provides methods to extend Laravel's validation system.
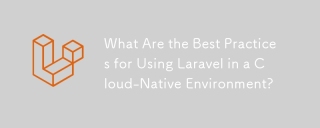
The article discusses best practices for deploying Laravel in cloud-native environments, focusing on scalability, reliability, and security. Key issues include containerization, microservices, stateless design, and optimization strategies.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

SublimeText3 Linux new version
SublimeText3 Linux latest version
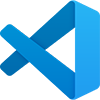
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
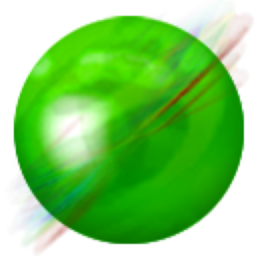
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
