Javascript is a powerful programming language with numerous data processing and calculation functions. Among them, group summation is an important function that can help us conduct in-depth analysis and processing of complex data.
We can use the array method in Javascript to perform group sum operations. For example, we have an array containing multiple sets of data, each set of data contains a name and corresponding value. Now we want to group this data by name and calculate the total value of each group.
First, we can use the reduce method in Javascript to group the data. The reduce method can traverse the array and use an accumulator to record the grouping of the data. Next, we can define an empty object to save the grouped data:
const data = [ { name: "Group A", value: 10 }, { name: "Group B", value: 15 }, { name: "Group A", value: 20 }, { name: "Group B", value: 25 }, { name: "Group C", value: 30 }, ]; const groupedData = data.reduce((acc, cur) => { if (!acc[cur.name]) { acc[cur.name] = []; } acc[cur.name].push(cur.value); return acc; }, {});
After executing the above code, we will get an object containing the grouped data:
{ "Group A": [10, 20], "Group B": [15, 25], "Group C": [30] }
Next , we can use the map method in Javascript to sum the grouped data. The map method can traverse the array and perform the specified operation on each element. Here, we sum each grouped data array and store the result in a new array:
const summedGroups = Object.keys(groupedData).map((groupName) => { const groupValues = groupedData[groupName]; const sum = groupValues.reduce((acc, cur) => acc + cur); return { name: groupName, value: sum }; });
After executing the above code, we will get an array containing the grouped summed data:
[ { name: "Group A", value: 30 }, { name: "Group B", value: 40 }, { name: "Group C", value: 30 } ]
Finally, we can output the results or use them in other ways. The complete code is as follows:
const data = [ { name: "Group A", value: 10 }, { name: "Group B", value: 15 }, { name: "Group A", value: 20 }, { name: "Group B", value: 25 }, { name: "Group C", value: 30 }, ]; const groupedData = data.reduce((acc, cur) => { if (!acc[cur.name]) { acc[cur.name] = []; } acc[cur.name].push(cur.value); return acc; }, {}); const summedGroups = Object.keys(groupedData).map((groupName) => { const groupValues = groupedData[groupName]; const sum = groupValues.reduce((acc, cur) => acc + cur); return { name: groupName, value: sum }; }); console.log(summedGroups);
Through the above code, we can easily perform group sum operations on arrays in Javascript, thereby achieving more efficient data processing and calculations.
The above is the detailed content of In-depth analysis of JavaScript's group sum operation. For more information, please follow other related articles on the PHP Chinese website!
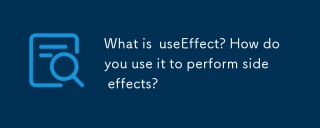
The article discusses useEffect in React, a hook for managing side effects like data fetching and DOM manipulation in functional components. It explains usage, common side effects, and cleanup to prevent issues like memory leaks.
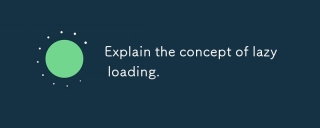
Lazy loading delays loading of content until needed, improving web performance and user experience by reducing initial load times and server load.
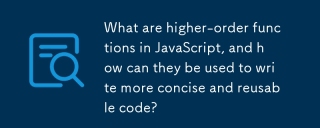
Higher-order functions in JavaScript enhance code conciseness, reusability, modularity, and performance through abstraction, common patterns, and optimization techniques.
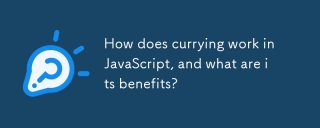
The article discusses currying in JavaScript, a technique transforming multi-argument functions into single-argument function sequences. It explores currying's implementation, benefits like partial application, and practical uses, enhancing code read
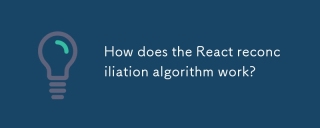
The article explains React's reconciliation algorithm, which efficiently updates the DOM by comparing Virtual DOM trees. It discusses performance benefits, optimization techniques, and impacts on user experience.Character count: 159
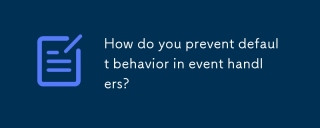
Article discusses preventing default behavior in event handlers using preventDefault() method, its benefits like enhanced user experience, and potential issues like accessibility concerns.
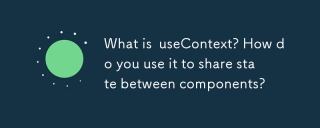
The article explains useContext in React, which simplifies state management by avoiding prop drilling. It discusses benefits like centralized state and performance improvements through reduced re-renders.
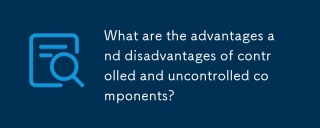
The article discusses the advantages and disadvantages of controlled and uncontrolled components in React, focusing on aspects like predictability, performance, and use cases. It advises on factors to consider when choosing between them.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SublimeText3 Linux new version
SublimeText3 Linux latest version

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
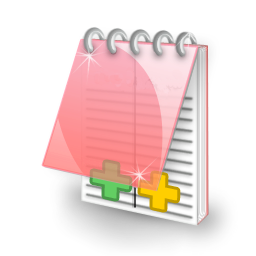
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
