


With the popularization of the Internet, a large amount of information is spread on the Internet, which also contains bad information, such as violence, pornography, abuse, etc. This information will not only affect the mental health of netizens, but also cause negative social effects. Therefore, during the development process of the website, sensitive words need to be filtered to protect the legitimate rights and interests of netizens. In development, the PHP programming language is a commonly used programming language. This article will introduce in detail how PHP filters and replaces sensitive words.
1. Overview
Normally, we need to determine whether sensitive words appear when accessing comments or publishing content on the website. If they appear, they need to be filtered or replaced. The traditional method is to match through regular expressions, but for longer and more complex words, matching will take a long time, causing the program to run slowly.
Now, we can use the trie tree algorithm in PHP to quickly identify sensitive words and process them.
2. Implementation of trie tree algorithm
The trie tree algorithm, also known as "dictionary tree", is a tree data structure used for fast retrieval. The biggest advantage of using the trie tree algorithm to search is that according to the given number of words, the search time has nothing to do with the length, only the number of words. That is, no matter how long the search string is, the search time is the same. This provides the possibility for PHP to quickly filter sensitive words.
To use the trie tree algorithm to quickly detect and filter sensitive words, we can first create a trie tree to record all sensitive words. For each string that needs to be detected, we can split the string into individual characters and then match them on the trie tree in order. If a position match fails, false is returned. Otherwise, continue the matching of the next character. If the leaf node is finally reached, the match is considered successful and filtering or replacement is performed.
3. Filtering and Replacement Implementation
After filtering sensitive words, you need to perform a replacement operation to replace the sensitive words with "*" or other characters to achieve the effect of protecting the privacy of netizens.
The method for PHP to filter sensitive words and replace them is as follows:
function filterWords($str, $trie,$replaceStr="*"){ $len = mb_strlen($str); $i = 0; $result = ''; while($i$t; $j++; if($node!=null && $node->end>0){//匹配到最后一个字符 for($k=$i;$knext = array(); $this->end = 0; $this->v = ''; } } function insertTrie(&$trie,$str){ $len=strlen($str); $tmp=$trie; for($i=0;$inext[$t])){ $tmp->next[$t] = new TrieTree(); } $tmp = $tmp->next[$t]; } $tmp->end=1; } $trie = new TrieTree(); $words=array("敏感词1","敏感词2","敏感词3"); foreach ($words as $word) { insertTrie($trie,$word); } $str="这是一个含有敏感词汇的字符串"; echo filterWords($str,$trie);
The above code is a simple example, using the trie tree algorithm implemented in PHP. Among them, the insertTrie() function is used to insert sensitive words into the trie tree, and the filterWords() function is used to filter sensitive words and perform replacement operations.
4. Summary
As there is a large amount of bad information on the Internet, it is very important to protect the legitimate rights and interests of netizens. Filtering and replacing sensitive words is also one of the effective means to prevent the spread of bad information on the Internet. This article introduces in detail the method of quickly filtering sensitive words in PHP and provides relevant code examples. I hope it will be helpful to PHP developers.
The above is the detailed content of Detailed explanation of how to filter and replace sensitive words in PHP. For more information, please follow other related articles on the PHP Chinese website!
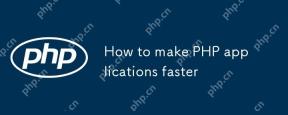
TomakePHPapplicationsfaster,followthesesteps:1)UseOpcodeCachinglikeOPcachetostoreprecompiledscriptbytecode.2)MinimizeDatabaseQueriesbyusingquerycachingandefficientindexing.3)LeveragePHP7 Featuresforbettercodeefficiency.4)ImplementCachingStrategiessuc
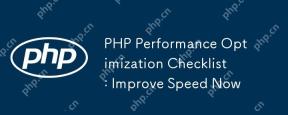
ToimprovePHPapplicationspeed,followthesesteps:1)EnableopcodecachingwithAPCutoreducescriptexecutiontime.2)ImplementdatabasequerycachingusingPDOtominimizedatabasehits.3)UseHTTP/2tomultiplexrequestsandreduceconnectionoverhead.4)Limitsessionusagebyclosin
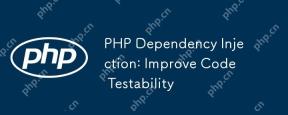
Dependency injection (DI) significantly improves the testability of PHP code by explicitly transitive dependencies. 1) DI decoupling classes and specific implementations make testing and maintenance more flexible. 2) Among the three types, the constructor injects explicit expression dependencies to keep the state consistent. 3) Use DI containers to manage complex dependencies to improve code quality and development efficiency.
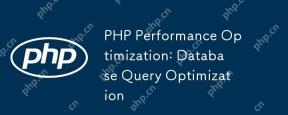
DatabasequeryoptimizationinPHPinvolvesseveralstrategiestoenhanceperformance.1)Selectonlynecessarycolumnstoreducedatatransfer.2)Useindexingtospeedupdataretrieval.3)Implementquerycachingtostoreresultsoffrequentqueries.4)Utilizepreparedstatementsforeffi
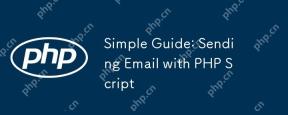
PHPisusedforsendingemailsduetoitsbuilt-inmail()functionandsupportivelibrarieslikePHPMailerandSwiftMailer.1)Usethemail()functionforbasicemails,butithaslimitations.2)EmployPHPMailerforadvancedfeatureslikeHTMLemailsandattachments.3)Improvedeliverability
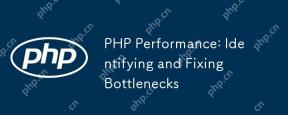
PHP performance bottlenecks can be solved through the following steps: 1) Use Xdebug or Blackfire for performance analysis to find out the problem; 2) Optimize database queries and use caches, such as APCu; 3) Use efficient functions such as array_filter to optimize array operations; 4) Configure OPcache for bytecode cache; 5) Optimize the front-end, such as reducing HTTP requests and optimizing pictures; 6) Continuously monitor and optimize performance. Through these methods, the performance of PHP applications can be significantly improved.
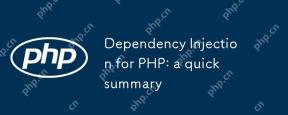
DependencyInjection(DI)inPHPisadesignpatternthatmanagesandreducesclassdependencies,enhancingcodemodularity,testability,andmaintainability.Itallowspassingdependencieslikedatabaseconnectionstoclassesasparameters,facilitatingeasiertestingandscalability.
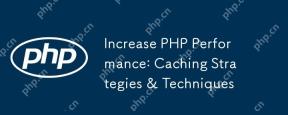
CachingimprovesPHPperformancebystoringresultsofcomputationsorqueriesforquickretrieval,reducingserverloadandenhancingresponsetimes.Effectivestrategiesinclude:1)Opcodecaching,whichstorescompiledPHPscriptsinmemorytoskipcompilation;2)DatacachingusingMemc


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
