In recent years, Golang has become one of the most popular programming languages today due to its many excellent features and perfect performance. However, whether you are using Golang for web development or other fields, error management is still an unavoidable problem. How to handle errors gracefully in Golang? What experiences and suggestions are there?
1. Error classification
In Golang, errors are placed in the error type. The Error type is defined to contain only one Error method, which returns a string. When an error occurs, a new Error type is usually created. In Golang, you can use the built-in error package or custom errors to represent error messages.
Generally speaking, errors in Golang can be divided into the following three categories:
1. Predefined errors
Predefined errors are built-in error types, usually used to represent errors in Golang System level error. For example, errors such as EOF are encountered during file reading. Most of these errors can be handled using the built-in error functions.
2. Custom errors
Custom errors are designed by programmers when writing code and can generate specific types of errors. For example, errors such as JSON parsing failure. For this type of error, the programmer must define the error type himself and return it when the function is called.
3. Runtime errors
Runtime errors are errors encountered by the Golang interpreter when running the program. For example, division by 0 errors, etc. These errors are usually caused by system-level errors and cannot be predicted, so programmers need to pay attention to handling such errors.
2. Go's error handling method
In Golang, the following two methods are usually used to handle errors:
1. Return value processing
In Golang, usually use Return error information in the value to handle errors. Before a function call, it is common to check whether the return value of other function calls is of the wrong type. If so, terminates the current function call and returns the error to the caller.
For example, the following code handles errors when reading the greeting.txt file. The function readGreeting returns the error err. If an error occurs, the function will return a nil value and the err error type.
func greet() error { contents, err := readGreeting("greeting.txt") if err != nil { return err } fmt.Printf("Greetings: %s\n", contents) return nil } func readGreeting(filename string) (string, error) { file, err := os.Open(filename) if err != nil { return "", err } defer file.Close() reader := bufio.NewReader(file) greeting, err := reader.ReadString('\n') if err != nil { return "", err } return greeting, nil }
2, panic and recover
Another way to handle errors in Golang is to use panic and recover. When an error occurs and is not caught, it causes the program to crash. However, if a programmer uses panic within a function, it means that an error has occurred. This panic information will be passed out until it is captured by recover.
For example, the following code example implements a simple example of database connection and access, and how to handle failure situations.
func main() { conn := openConnection("localhost", "mydb") defer closeConnection(conn) if err := insertData(conn, "test"); err != nil { log.Fatal(err) } } func openConnection(ip, dbname string) *DB { conn, err := openDB(ip, dbname) if err != nil { panic(err) } return conn } func insertData(db *DB, data string) error { if db == nil { panic("No database connection!") } _, err := db.Exec("INSERT INTO data VALUES (?)", data) return err } func closeConnection(db *DB) { if db == nil { panic("No database connection!") } db.Close() } func recoverFunction() { if r := recover(); r != nil { fmt.Println("Recovered", r) } }
3. Some suggestions
In Golang, error handling needs to be completed by programmers themselves. However, some experience and suggestions can help programmers better handle errors:
1. For system-level errors, you can consider using the built-in error types or errors package, which is easy to understand and use.
2. For custom errors, you can define the error types yourself in the program and return these errors with functions carrying necessary parameters.
3. Using the defer keyword can ensure that when you forget to clean up resources when writing code, you can still clean up resources correctly. This is a very convenient way.
4. Be careful when using panic and recover in programs, and try to avoid using them in programs.
In short, error handling is still an issue that needs attention in Golang. Correct error handling can help programmers deal with various errors that occur in the program and improve the reliability and robustness of the program.
The above is the detailed content of How to handle errors gracefully in Golang. For more information, please follow other related articles on the PHP Chinese website!
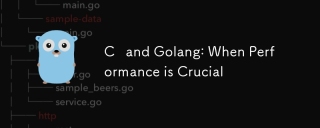
C is more suitable for scenarios where direct control of hardware resources and high performance optimization is required, while Golang is more suitable for scenarios where rapid development and high concurrency processing are required. 1.C's advantage lies in its close to hardware characteristics and high optimization capabilities, which are suitable for high-performance needs such as game development. 2.Golang's advantage lies in its concise syntax and natural concurrency support, which is suitable for high concurrency service development.
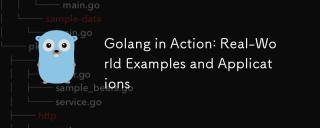
Golang excels in practical applications and is known for its simplicity, efficiency and concurrency. 1) Concurrent programming is implemented through Goroutines and Channels, 2) Flexible code is written using interfaces and polymorphisms, 3) Simplify network programming with net/http packages, 4) Build efficient concurrent crawlers, 5) Debugging and optimizing through tools and best practices.
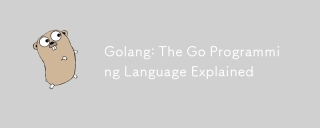
The core features of Go include garbage collection, static linking and concurrency support. 1. The concurrency model of Go language realizes efficient concurrent programming through goroutine and channel. 2. Interfaces and polymorphisms are implemented through interface methods, so that different types can be processed in a unified manner. 3. The basic usage demonstrates the efficiency of function definition and call. 4. In advanced usage, slices provide powerful functions of dynamic resizing. 5. Common errors such as race conditions can be detected and resolved through getest-race. 6. Performance optimization Reuse objects through sync.Pool to reduce garbage collection pressure.
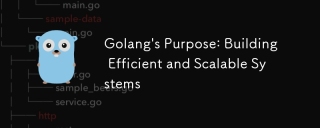
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
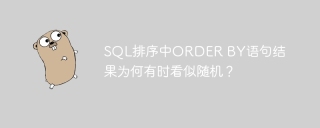
Confused about the sorting of SQL query results. In the process of learning SQL, you often encounter some confusing problems. Recently, the author is reading "MICK-SQL Basics"...
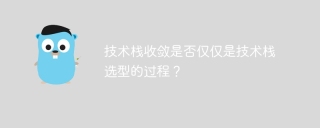
The relationship between technology stack convergence and technology selection In software development, the selection and management of technology stacks are a very critical issue. Recently, some readers have proposed...
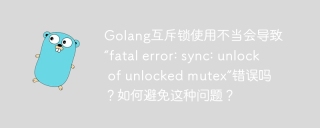
Golang ...
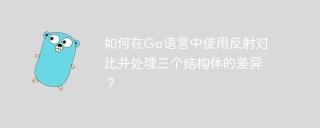
How to compare and handle three structures in Go language. In Go programming, it is sometimes necessary to compare the differences between two structures and apply these differences to the...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
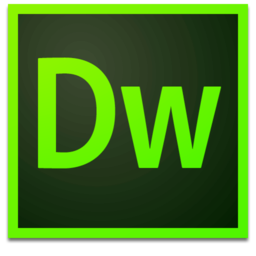
Dreamweaver Mac version
Visual web development tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.