When developing PHP, you often encounter situations where you need to convert objects into strings or arrays. At this time, we need to use some functions and techniques in PHP. This article will explain how to convert an object to a string or array and give some sample code.
1. Convert an object to a string
In PHP, we can convert an object to a string by passing it to the echo function. However, this approach only works for simple objects. If you want to convert a complex object to a string, you need to use the __toString() method in PHP.
__toString() method is one of the magic methods in PHP, used to convert objects into strings. With this method, we can easily convert the object into a string.
The sample code is as follows:
class Person { public $name; public $age; public function __toString() { return $this->name . ", " . $this->age; } } $person = new Person(); $person->name = "Tom"; $person->age = 30; echo $person; //输出Tom, 30
2. Convert objects to arrays
In PHP, you can use the function get_object_vars() to convert simple objects into arrays. The get_object_vars() function returns an array containing all the properties of the object and their values.
The sample code is as follows:
class Person { public $name; public $age; public function __construct($name, $age) { $this->name = $name; $this->age = $age; } } $person = new Person("Tom", 30); $array = get_object_vars($person); print_r($array); //输出Array([name] => Tom [age] => 30)
3. Convert object array to string array
We can also convert object array to string array. The array_map() function in PHP can help us accomplish this task. The array_map() function passes each element of one or more arrays to the specified function and returns a new array whose elements are the return values of each function.
The sample code is as follows:
class Person { public $name; public $age; public function __construct($name, $age) { $this->name = $name; $this->age = $age; } public function toString() { return $this->name . ", " . $this->age; } } $person1 = new Person("Tom", 30); $person2 = new Person("Jerry", 35); $array = array($person1, $person2); $stringArray = array_map(function($person) { return $person->toString(); }, $array); print_r($stringArray); //输出Array([0] => Tom, 30 [1] => Jerry, 35)
The above is how PHP converts objects into strings, arrays, and object arrays. Through these techniques, we can easily complete the conversion between objects, strings, and arrays, and play their role in development.
The above is the detailed content of How to convert object to string or array in php. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

SublimeText3 English version
Recommended: Win version, supports code prompts!

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
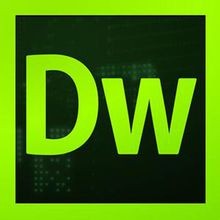
Dreamweaver CS6
Visual web development tools

Atom editor mac version download
The most popular open source editor
