With the development of the Internet, website and application development are becoming more and more common, and more and more functions need to be implemented. The check-in function is a very basic but very popular function. For social networking websites and applications, the check-in function is a very important part. Let's take the PHP language as an example to explain how to implement the check-in function.
1. Preparation work
Before starting, we need to prepare the following work:
- Install an interpreter with PHP version 7.0 or above, such as XAMPP.
- Create a database and import the sign-in table.
We are using the mysql database here. After opening the database, execute the following SQL statement:
CREATE DATABASE sign; USE sign; CREATE TABLE checkin( id MEDIUMINT NOT NULL AUTO_INCREMENT, user_id VARCHAR(16) NOT NULL, create_time DATETIME NOT NULL, PRIMARY KEY (id) )ENGINE=InnoDB DEFAULT CHARSET=utf8;
Here we have created a database named sign, which contains a checkin table , used to store sign-in information. The checkin table contains three fields: id represents the unique identifier of the check-in record, user_id represents the ID of the check-in user, and create_time represents the check-in time.
2. Code Implementation
Next, we start writing the code for the check-in function. First we need to create an index.php file, the code is as follows:
nbsp;html> <meta> <title>签到</title> <?php if(isset($_POST['submit'])) { // 判断是否提交了表单 $userId = $_POST['user_id']; // 获取用户 ID $conn = mysqli_connect('localhost', 'root', '', 'sign'); // 连接数据库 if(!$conn) { die('连接数据库失败: ' . mysqli_error($conn)); // 判断连接是否成功 } $query = "INSERT INTO checkin (user_id, create_time) VALUES ('$userId', NOW())"; // SQL 插入语句 if(mysqli_query($conn, $query)) { // 判断插入是否成功 echo "<h2>签到成功!"; } else { echo "<h2 id="签到失败">签到失败!</h2>"; } mysqli_close($conn); // 关闭connection } ?>
Code idea:
- When the user opens the check-in page, a form is displayed on the page, and there is an input box in the form And a submit button to enter the user ID and submit the check-in information.
- When the user enters the ID and clicks the submit button, the form will send a request to the server from the page to determine whether the user has signed in and save the sign-in information in the database.
- The last page displays the check-in results.
3. Complete code
The following is the complete check-in function code (index.php file).
nbsp;html> <meta> <title>签到</title> <?php if(isset($_POST['submit'])) { // 判断是否提交了表单 $userId = $_POST['user_id']; // 获取用户 ID $conn = mysqli_connect('localhost', 'root', '', 'sign'); // 连接数据库 if(!$conn) { die('连接数据库失败: ' . mysqli_error($conn)); // 判断连接是否成功 } $query = "INSERT INTO checkin (user_id, create_time) VALUES ('$userId', NOW())"; // SQL 插入语句 if(mysqli_query($conn, $query)) { // 判断插入是否成功 echo "<h2>签到成功!"; } else { echo "<h2 id="签到失败">签到失败!</h2>"; } mysqli_close($conn); // 关闭connection } ?>
Code analysis:
- ##if(isset($_POST['submit']))
means that if the form submits data, it will be executed after the form is submitted. logic (sign-in operation).
- $userId = $_POST['user_id'];
Get the user ID.
- $conn = mysqli_connect('localhost', 'root', '', 'sign');
Connect to the database.
- if(!$conn)
If the connection fails, the program execution will exit and a failure message will be prompted.
- $query = "INSERT INTO checkin (user_id, create_time) VALUES ('$userId', NOW())";
Add a new check-in record.
- if(mysqli_query($conn, $query))
If the addition is successful, the user will be prompted to sign in successfully and a sign-in record will be added.
- mysqli_close($conn)
Close the database connection.
The above is the detailed content of Steps to implement the PHP check-in function (with code). For more information, please follow other related articles on the PHP Chinese website!
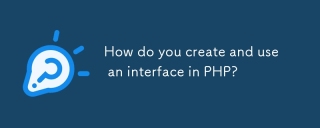
The article explains how to create, implement, and use interfaces in PHP, focusing on their benefits for code organization and maintainability.
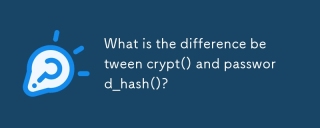
The article discusses the differences between crypt() and password_hash() in PHP for password hashing, focusing on their implementation, security, and suitability for modern web applications.
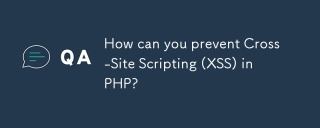
Article discusses preventing Cross-Site Scripting (XSS) in PHP through input validation, output encoding, and using tools like OWASP ESAPI and HTML Purifier.
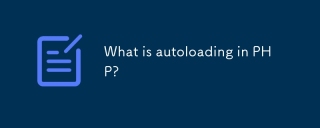
Autoloading in PHP automatically loads class files when needed, improving performance by reducing memory use and enhancing code organization. Best practices include using PSR-4 and organizing code effectively.
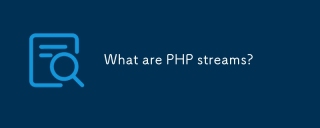
PHP streams unify handling of resources like files, network sockets, and compression formats via a consistent API, abstracting complexity and enhancing code flexibility and efficiency.
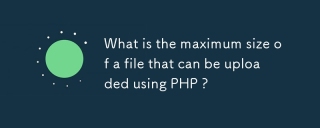
The article discusses managing file upload sizes in PHP, focusing on the default limit of 2MB and how to increase it by modifying php.ini settings.
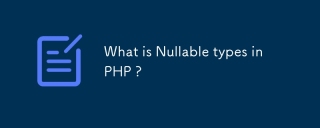
The article discusses nullable types in PHP, introduced in PHP 7.1, allowing variables or parameters to be either a specified type or null. It highlights benefits like improved readability, type safety, and explicit intent, and explains how to declar
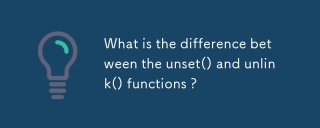
The article discusses the differences between unset() and unlink() functions in programming, focusing on their purposes and use cases. Unset() removes variables from memory, while unlink() deletes files from the filesystem. Both are crucial for effec


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
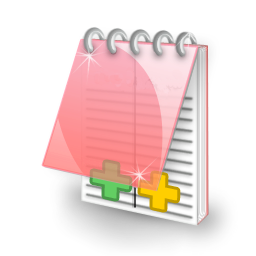
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

SublimeText3 English version
Recommended: Win version, supports code prompts!

SublimeText3 Linux new version
SublimeText3 Linux latest version

Notepad++7.3.1
Easy-to-use and free code editor
