Golang is a popular programming language for writing efficient and high-performance web applications. In recent years, due to its powerful development and operation capabilities, more and more companies and developers choose to use Golang for development. During development, some applications require https forwarding, so how to use Golang to implement https forwarding?
Before introducing the implementation method, let us first understand the concept of https. https is the abbreviation of Hyper Text Transfer Protocol Secure, which is simply the secure version of HTTP. https is more secure than http because https uses random numbers, encryption functions, and digital signatures to encrypt and authenticate data. In https, the communication between the client and the server is encrypted, which ensures the security of data transmission.
Before implementing https forwarding, we need to prepare the following work:
- SSL certificate: Before implementing https forwarding, we need to obtain an SSL certificate from the CA (Certificate Authority) to ensure Security of data transmission.
- Go language environment: We need to install the Go language environment locally.
Next, we will introduce the method of implementing https forwarding through Golang:
- Introducing the net/http and crypto/tls libraries
In Go language, we can implement http requests and responses through the net/http library. When implementing https requests, we need to introduce the crypto/tls library.
import ( "crypto/tls" "net/http" )
- Configure TLS client
When performing https forwarding, we need to set up the TLS client to ensure the security of data transmission. Before configuring TLS, we need to obtain the certificate from the CA (Certificate Authority) and then load the certificate. The code is as follows:
cert, err := tls.LoadX509KeyPair("server.crt", "server.key") if err != nil { log.Fatal(err) } config := &tls.Config{Certificates: []tls.Certificate{cert}, ServerName: "example.com"}
Among them, the LoadX509KeyPair function loads the certificate under the specified path. The Certificates array contains the client certificate and private key. ServerName is the name of the server and must be specified.
- Set up the proxy server
Next, we need to set up the proxy server to achieve https forwarding. In the go language, we can use the ReverseProxy function of the httputil library to implement proxy server settings. The code is as follows:
proxyUrl, _ := url.Parse("https://target-server.com") proxy := httputil.NewSingleHostReverseProxy(proxyUrl) server := &http.Server{ Addr: ":8080", Handler: proxy, TLSConfig: config, TLSNextProto: make(map[string]func(*http.Server, *tls.Conn, http.Handler), 0), } log.Fatal(server.ListenAndServeTLS("", ""))
Among them, replace target-server.com with the actual proxy server address, create a proxy and forward the proxy request to the target server. The ListenAndServeTLS function is used to start the server and can specify the TCP port number and SSL certificate path. So, how does this code work?
The browser sends an https request to the server.
After the server receives the request, the TLS client performs authentication and encryption, and creates a reverse proxy server to forward the request to the target server.
After the target server receives the request, it returns the response to the reverse proxy server.
The reverse proxy server forwards the response to the client to complete https forwarding.
The above is how to implement https forwarding through Golang. The powerful features of Golang greatly simplify the implementation process of https and improve development efficiency. Therefore, if you need to implement https forwarding in your application, Golang is a good choice.
The above is the detailed content of How to implement https forwarding using Golang. For more information, please follow other related articles on the PHP Chinese website!
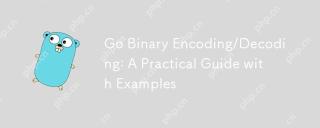
Go's encoding/binary package is a tool for processing binary data. 1) It supports small-endian and large-endian endian byte order and can be used in network protocols and file formats. 2) The encoding and decoding of complex structures can be handled through Read and Write functions. 3) Pay attention to the consistency of byte order and data type when using it, especially when data is transmitted between different systems. This package is suitable for efficient processing of binary data, but requires careful management of byte slices and lengths.
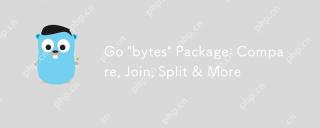
The"bytes"packageinGoisessentialbecauseitoffersefficientoperationsonbyteslices,crucialforbinarydatahandling,textprocessing,andnetworkcommunications.Byteslicesaremutable,allowingforperformance-enhancingin-placemodifications,makingthispackage
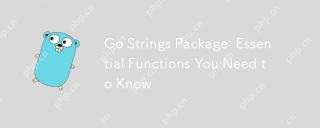
Go'sstringspackageincludesessentialfunctionslikeContains,TrimSpace,Split,andReplaceAll.1)Containsefficientlychecksforsubstrings.2)TrimSpaceremoveswhitespacetoensuredataintegrity.3)SplitparsesstructuredtextlikeCSV.4)ReplaceAlltransformstextaccordingto
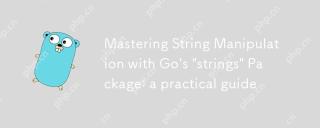
ThestringspackageinGoiscrucialforefficientstringmanipulationduetoitsoptimizedfunctionsandUnicodesupport.1)ItsimplifiesoperationswithfunctionslikeContains,Join,Split,andReplaceAll.2)IthandlesUTF-8encoding,ensuringcorrectmanipulationofUnicodecharacters
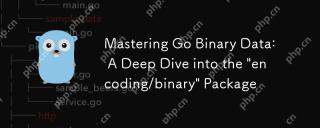
The"encoding/binary"packageinGoiscrucialforefficientbinarydatamanipulation,offeringperformancebenefitsinnetworkprogramming,fileI/O,andsystemoperations.Itsupportsendiannessflexibility,handlesvariousdatatypes,andisessentialforcustomprotocolsa
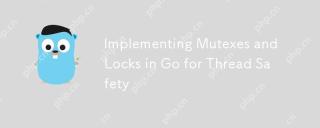
In Go, using mutexes and locks is the key to ensuring thread safety. 1) Use sync.Mutex for mutually exclusive access, 2) Use sync.RWMutex for read and write operations, 3) Use atomic operations for performance optimization. Mastering these tools and their usage skills is essential to writing efficient and reliable concurrent programs.
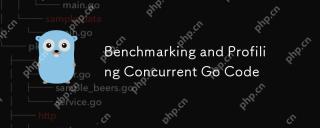
How to optimize the performance of concurrent Go code? Use Go's built-in tools such as getest, gobench, and pprof for benchmarking and performance analysis. 1) Use the testing package to write benchmarks to evaluate the execution speed of concurrent functions. 2) Use the pprof tool to perform performance analysis and identify bottlenecks in the program. 3) Adjust the garbage collection settings to reduce its impact on performance. 4) Optimize channel operation and limit the number of goroutines to improve efficiency. Through continuous benchmarking and performance analysis, the performance of concurrent Go code can be effectively improved.
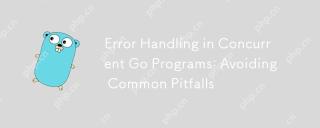
The common pitfalls of error handling in concurrent Go programs include: 1. Ensure error propagation, 2. Processing timeout, 3. Aggregation errors, 4. Use context management, 5. Error wrapping, 6. Logging, 7. Testing. These strategies help to effectively handle errors in concurrent environments.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Zend Studio 13.0.1
Powerful PHP integrated development environment

Notepad++7.3.1
Easy-to-use and free code editor
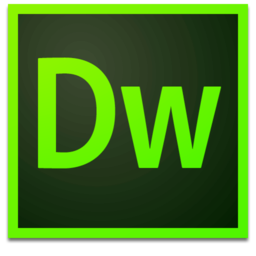
Dreamweaver Mac version
Visual web development tools
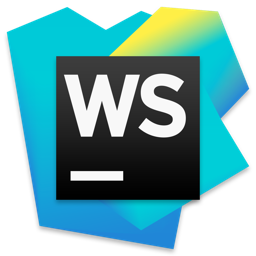
WebStorm Mac version
Useful JavaScript development tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
