


Recently I was working on an e-commerce website and needed to implement the function of clicking to select a product list. I took this opportunity to learn the Vue framework.
In Vue, it is very simple to click on the selected list. You only need to use the v-on instruction and v-bind instruction provided by Vue.
First, define a product list in the template, with a checkbox behind each product.
- <input>
The v-for instruction here is a loop instruction in Vue, which is used to traverse each element in the itemList array. The v-bind directive is an attribute binding directive in Vue, which can bind data in Vue to HTML elements. The v-model directive is a two-way binding directive in Vue, which can achieve two-way synchronization of data.
In data, declare the itemList array and initialize the id, name and checked attributes of each element.
data() { return { itemList: [ { id: 'item1', name: '商品1', checked: false }, { id: 'item2', name: '商品2', checked: false }, { id: 'item3', name: '商品3', checked: false } ] } }
When the user clicks on the checkbox, the click event will be triggered. We only need to define a toggleCheck method in methods to invert the status of the currently selected checkbox.
methods: { toggleCheck(item) { item.checked = !item.checked; } }
Finally, use the v-on directive in the template to bind the click event and call the toggleCheck method.
<input>
In this way, the function of clicking on the selected list can be realized.
To summarize, Vue is very simple to implement a click-to-select list. You only need to use the v-for, v-bind, v-model and v-on instructions provided by Vue to bind the data in HTML and Vue. Defined, two-way synchronization of data and binding of events can be achieved. The Vue framework is not only low-cost to learn, but also very suitable for developing small and medium-sized projects. If you also want to improve your front-end skills, you might as well learn the Vue framework!
The above is the detailed content of How to implement the function of clicking to select a product list in vue. For more information, please follow other related articles on the PHP Chinese website!

HTML and React can be seamlessly integrated through JSX to build an efficient user interface. 1) Embed HTML elements using JSX, 2) Optimize rendering performance using virtual DOM, 3) Manage and render HTML structures through componentization. This integration method is not only intuitive, but also improves application performance.

React efficiently renders data through state and props, and handles user events through the synthesis event system. 1) Use useState to manage state, such as the counter example. 2) Event processing is implemented by adding functions in JSX, such as button clicks. 3) The key attribute is required to render the list, such as the TodoList component. 4) For form processing, useState and e.preventDefault(), such as Form components.

React interacts with the server through HTTP requests to obtain, send, update and delete data. 1) User operation triggers events, 2) Initiate HTTP requests, 3) Process server responses, 4) Update component status and re-render.

React is a JavaScript library for building user interfaces that improves efficiency through component development and virtual DOM. 1. Components and JSX: Use JSX syntax to define components to enhance code intuitiveness and quality. 2. Virtual DOM and Rendering: Optimize rendering performance through virtual DOM and diff algorithms. 3. State management and Hooks: Hooks such as useState and useEffect simplify state management and side effects handling. 4. Example of usage: From basic forms to advanced global state management, use the ContextAPI. 5. Common errors and debugging: Avoid improper state management and component update problems, and use ReactDevTools to debug. 6. Performance optimization and optimality

Reactisafrontendlibrary,focusedonbuildinguserinterfaces.ItmanagesUIstateandupdatesefficientlyusingavirtualDOM,andinteractswithbackendservicesviaAPIsfordatahandling,butdoesnotprocessorstoredataitself.

React can be embedded in HTML to enhance or completely rewrite traditional HTML pages. 1) The basic steps to using React include adding a root div in HTML and rendering the React component via ReactDOM.render(). 2) More advanced applications include using useState to manage state and implement complex UI interactions such as counters and to-do lists. 3) Optimization and best practices include code segmentation, lazy loading and using React.memo and useMemo to improve performance. Through these methods, developers can leverage the power of React to build dynamic and responsive user interfaces.

React is a JavaScript library for building modern front-end applications. 1. It uses componentized and virtual DOM to optimize performance. 2. Components use JSX to define, state and attributes to manage data. 3. Hooks simplify life cycle management. 4. Use ContextAPI to manage global status. 5. Common errors require debugging status updates and life cycles. 6. Optimization techniques include Memoization, code splitting and virtual scrolling.

React's future will focus on the ultimate in component development, performance optimization and deep integration with other technology stacks. 1) React will further simplify the creation and management of components and promote the ultimate in component development. 2) Performance optimization will become the focus, especially in large applications. 3) React will be deeply integrated with technologies such as GraphQL and TypeScript to improve the development experience.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
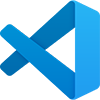
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
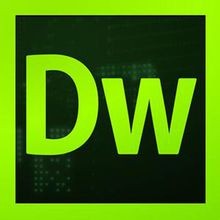
Dreamweaver CS6
Visual web development tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.