Preface
In the process of using Vue for development, we will encounter situations where we need to intercept network requests, and we also need to exclude some requests from being intercepted, that is, a whitelist. This article will introduce the method of Vue request interception whitelist implementation.
Text
When developing with Vue, we usually use axios to send network requests. In axios, we can customize the interceptor of network requests. Custom interceptors can help us modify the request configuration before sending a network request, or process the response results after the request response.
Basic usage of Vue request interceptor
In Vue, we first need to install and introduce axios:
npm install axios -S
import axios from 'axios'
Then we can set axios, set the request interceptor and Response interceptor:
axios.interceptors.request.use( config => { // 在请求发送之前做一些事情 return config; }, error => { // 处理请求错误时做一些事情 return error; }); axios.interceptors.response.use( response => { // 处理响应数据做一些事情 return response; }, error => { // 处理响应错误做一些事情 return error; });
The config object in the request interceptor contains information related to the current request. We can modify this object in the interceptor, such as adding some request header information, etc.
The response object in the response interceptor contains the return information of the network request. We can also modify this object, such as judging error codes, etc.
Vue request interception whitelist implementation
In some specific scenarios, we do not want to intercept certain requests. At this time, we need to modify the request interceptor to implement the whitelist function. .
In the request interceptor, we can decide whether to intercept the current request by judging whether the URL of the current request is in the whitelist. Here we need to define a whitelist list and make a judgment in the request interceptor:
const whitelist = ['/login', '/register']; // 定义白名单 axios.interceptors.request.use( config => { // 在请求发送之前做一些事情 if (whitelist.indexOf(config.url) === -1) { // 当前请求不在白名单之中,进行拦截 const token = localStorage.getItem('token'); if (token) { config.headers.Authorization = token; } else { window.location.href = '/login'; } } return config; }, error => { // 处理请求错误时做一些事情 return error; });
In the above code, we first define a whitelist list, and then use the request interceptor to check the URL of the current request. Make a judgment and intercept if the current request is not in the whitelist. In this interceptor, we also added some logic code, such as obtaining the local token, adding the Authorization field to the request header, etc.
In the above code, if the requested URL is not in the whitelist, it will be intercepted, and the following operations will be performed during the interception:
- Get the local token.
- Determine whether the token exists locally, and if not, jump to the login page.
- If there is a token, add the Authorization field in headers to pass it to the backend.
After completing the above operations, return the processed config object and the request will continue to be sent.
Summary
The application of Vue request interceptor is very flexible. Through custom interceptors, we can modify and intercept network requests at any time, and at the same time realize the whitelist function, that is Exclude certain requests from interception. In actual project development, request interception whitelisting is an essential function, so we need to learn how to customize the request interception interceptor to implement request whitelisting.
The above is the detailed content of How to implement request interception whitelist in vue. For more information, please follow other related articles on the PHP Chinese website!
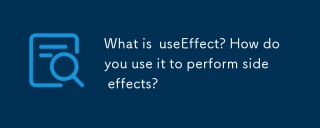
The article discusses useEffect in React, a hook for managing side effects like data fetching and DOM manipulation in functional components. It explains usage, common side effects, and cleanup to prevent issues like memory leaks.
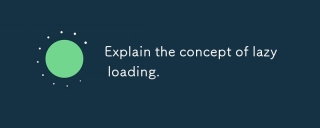
Lazy loading delays loading of content until needed, improving web performance and user experience by reducing initial load times and server load.
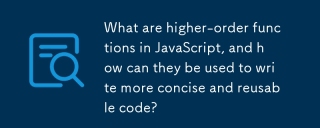
Higher-order functions in JavaScript enhance code conciseness, reusability, modularity, and performance through abstraction, common patterns, and optimization techniques.
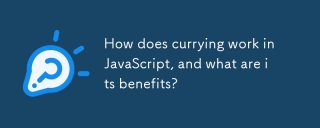
The article discusses currying in JavaScript, a technique transforming multi-argument functions into single-argument function sequences. It explores currying's implementation, benefits like partial application, and practical uses, enhancing code read
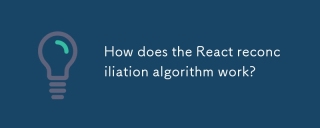
The article explains React's reconciliation algorithm, which efficiently updates the DOM by comparing Virtual DOM trees. It discusses performance benefits, optimization techniques, and impacts on user experience.Character count: 159
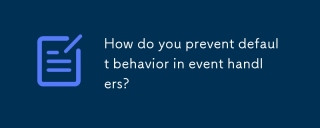
Article discusses preventing default behavior in event handlers using preventDefault() method, its benefits like enhanced user experience, and potential issues like accessibility concerns.
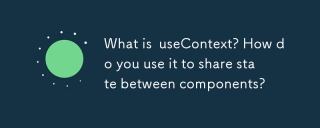
The article explains useContext in React, which simplifies state management by avoiding prop drilling. It discusses benefits like centralized state and performance improvements through reduced re-renders.
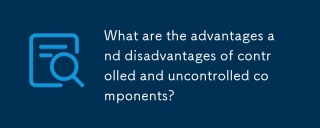
The article discusses the advantages and disadvantages of controlled and uncontrolled components in React, focusing on aspects like predictability, performance, and use cases. It advises on factors to consider when choosing between them.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
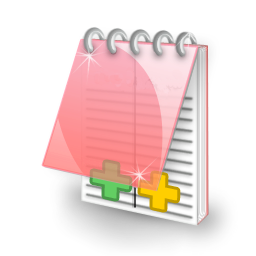
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
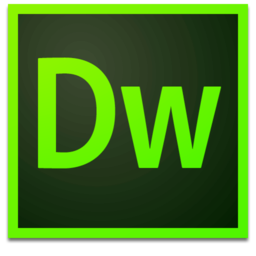
Dreamweaver Mac version
Visual web development tools

Notepad++7.3.1
Easy-to-use and free code editor
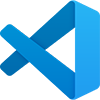
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
