Laravel is a popular PHP framework that provides many functions, including changing user passwords. In this article, we will introduce how to change user password in Laravel. The following are the specific steps:
Step 1: Create a route for changing passwords
First, we need to create a Laravel route for changing user passwords. You can add the following code in the web.php file:
Route::get('/password', 'UserController@password')->name('password'); Route::post('/password', 'UserController@updatePassword')->name('updatePassword');
Here we define a GET and POST route. The GET route is used to display the form for changing the password, and the POST route is used to submit the form data and perform the password update operation. At the same time, UserController@password and UserController@updatePassword are the two controller methods we need to create.
Step 2: Create a form to change the password
In the resources/views directory, we create a password.blade.php view file, which contains the form for updating the password. The following is a sample code:
There are three input boxes for entering the current password, new password and confirming the new password. The form's submission address is set to the route name we defined in the route.
Step 3: Create controller methods
Now we need to create two methods in the UserController controller: password and updatePassword. The following is the sample code:
public function password() { return view('password'); } public function updatePassword(Request $request) { $this->validate($request, [ 'current-password' => 'required', 'new-password' => 'required|string|min:6|confirmed' ]); $user = Auth::user(); $currentPassword = $user->password; $passwordMatches = Hash::check($request['current-password'], $currentPassword); if ($passwordMatches) { $user->password = Hash::make($request['new-password']); $user->save(); return redirect()->back()->with('success', 'Password updated successfully!'); } else { return redirect()->back()->withErrors(['current-password' => 'Incorrect current password.']); } }
In the updatePassword method, we first verify that the form input complies with the rules. Then, we obtain the current user data through the Auth::user() method, and then verify whether the current password matches the password in the database through the Hash::check() method. If the password matches successfully, we will hash the new password using the Hash::make() method and save it to the database.
Step 4: Test the password change function
Now we have created the route, form and controller method. We can access the password change page by accessing the localhost/password address and test the code we wrote. If all goes well, we can let users change their passwords!
Conclusion
It is very convenient to change user passwords in Laravel. We only need to create a form, route and controller method to achieve this functionality. I hope this article will help you change user passwords in Laravel.
The above is the detailed content of Let's talk about how to change user password in Laravel. For more information, please follow other related articles on the PHP Chinese website!

Laravel is suitable for building web applications quickly, while Python is suitable for a wider range of application scenarios. 1.Laravel provides EloquentORM, Blade template engine and Artisan tools to simplify web development. 2. Python is known for its dynamic types, rich standard library and third-party ecosystem, and is suitable for Web development, data science and other fields.

Laravel and Python each have their own advantages: Laravel is suitable for quickly building feature-rich web applications, and Python performs well in the fields of data science and general programming. 1.Laravel provides EloquentORM and Blade template engines, suitable for building modern web applications. 2. Python has a rich standard library and third-party library, and Django and Flask frameworks meet different development needs.

Laravel is worth choosing because it can make the code structure clear and the development process more artistic. 1) Laravel is based on PHP, follows the MVC architecture, and simplifies web development. 2) Its core functions such as EloquentORM, Artisan tools and Blade templates enhance the elegance and robustness of development. 3) Through routing, controllers, models and views, developers can efficiently build applications. 4) Advanced functions such as queue and event monitoring further improve application performance.

Laravel is not only a back-end framework, but also a complete web development solution. It provides powerful back-end functions, such as routing, database operations, user authentication, etc., and supports front-end development, improving the development efficiency of the entire web application.

Laravel is suitable for web development, Python is suitable for data science and rapid prototyping. 1.Laravel is based on PHP and provides elegant syntax and rich functions, such as EloquentORM. 2. Python is known for its simplicity, widely used in Web development and data science, and has a rich library ecosystem.

Laravelcanbeeffectivelyusedinreal-worldapplicationsforbuildingscalablewebsolutions.1)ItsimplifiesCRUDoperationsinRESTfulAPIsusingEloquentORM.2)Laravel'secosystem,includingtoolslikeNova,enhancesdevelopment.3)Itaddressesperformancewithcachingsystems,en

Laravel's core functions in back-end development include routing system, EloquentORM, migration function, cache system and queue system. 1. The routing system simplifies URL mapping and improves code organization and maintenance. 2.EloquentORM provides object-oriented data operations to improve development efficiency. 3. The migration function manages the database structure through version control to ensure consistency. 4. The cache system reduces database queries and improves response speed. 5. The queue system effectively processes large-scale data, avoid blocking user requests, and improve overall performance.

Laravel performs strongly in back-end development, simplifying database operations through EloquentORM, controllers and service classes handle business logic, and providing queues, events and other functions. 1) EloquentORM maps database tables through the model to simplify query. 2) Business logic is processed in controllers and service classes to improve modularity and maintainability. 3) Other functions such as queue systems help to handle complex needs.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
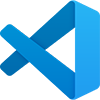
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
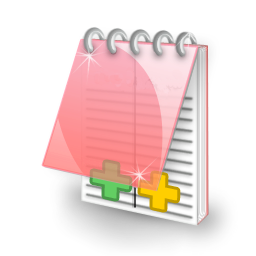
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function