


How to execute PHP query statement by clicking a button to complete data query
In the process of web development, it is often necessary to obtain data from the database. So how to query data from the database through PHP? This article will focus on how to execute PHP query statements by clicking buttons to complete data queries.
1. Create a database and data table
First, we need to create a database and data table in the MySQL database to store data. The test database and test_table data table can be created through the following SQL statements:
CREATE DATABASE test; USE test; CREATE TABLE test_table ( id INT(6) UNSIGNED AUTO_INCREMENT PRIMARY KEY, name VARCHAR(30) NOT NULL, age INT(6) NOT NULL, address VARCHAR(50) )
2. Connect to the database
In PHP, we can connect to the MySQL database through the mysqli extension. The following is a sample code for connecting to the database:
<?php $servername = "localhost"; $username = "username"; $password = "password"; $dbname = "test"; // 创建连接 $conn = mysqli_connect($servername, $username, $password, $dbname); // 检查连接 if (!$conn) { die("Connection failed: " . mysqli_connect_error()); } echo "Connected successfully"; ?>
3. Write query statements
Next, we need to write query statements to obtain data. The following is a sample code to obtain all the data in the test_table table:
<?php $sql = "SELECT * FROM test_table"; $result = mysqli_query($conn, $sql); if (mysqli_num_rows($result) > 0) { // 输出数据 while($row = mysqli_fetch_assoc($result)) { echo "id: " . $row["id"]. " - Name: " . $row["name"]. " - Age: " . $row["age"]. " - Address: " . $row["address"]. "<br>"; } } else { echo "0 结果"; } mysqli_close($conn); ?>
4. Click the button to execute the query statement
Through the above code, we can obtain data from the database, but only through The browser automatically executes the query statement when accessing the page. So how to execute the query statement by clicking the button? This can be achieved through the following steps:
- Add a button in the HTML page
<button>查询</button>
- Use JavaScript to listen to the click event of the button and send it to the server through Ajax Request
<script> $(document).ready(function(){ $("#query_button").click(function(){ $.ajax({ url:"query.php", //请求的PHP文件 success:function(result){ $("#result").html(result); //将结果输出到页面的result标签中 } }); }); }); </script>
- Write the code to execute the query statement in the query.php file and return the result to the client
<?php $sql = "SELECT * FROM test_table"; $result = mysqli_query($conn, $sql); if (mysqli_num_rows($result) > 0) { // 输出数据 while($row = mysqli_fetch_assoc($result)) { echo "id: " . $row["id"]. " - Name: " . $row["name"]. " - Age: " . $row["age"]. " - Address: " . $row["address"]. "<br>"; } } else { echo "0 结果"; } mysqli_close($conn); ?>
Go through the above steps. Implement the function of clicking a button to execute a PHP query statement.
5. Summary
This article introduces how to query data from the database through PHP and execute the query statement by clicking a button. By studying this article, readers can learn how to use the mysqli extension to connect to the MySQL database, how to write query statements, and how to use Ajax to update page content without refreshing the page. Hope this article can be helpful to readers.
The above is the detailed content of How to execute PHP query statement by clicking a button to complete data query. For more information, please follow other related articles on the PHP Chinese website!
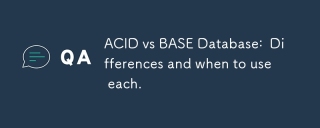
The article compares ACID and BASE database models, detailing their characteristics and appropriate use cases. ACID prioritizes data integrity and consistency, suitable for financial and e-commerce applications, while BASE focuses on availability and
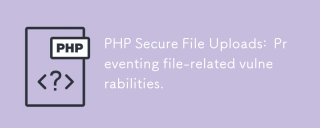
The article discusses securing PHP file uploads to prevent vulnerabilities like code injection. It focuses on file type validation, secure storage, and error handling to enhance application security.
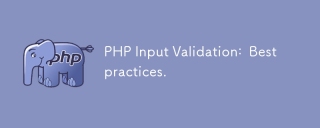
Article discusses best practices for PHP input validation to enhance security, focusing on techniques like using built-in functions, whitelist approach, and server-side validation.
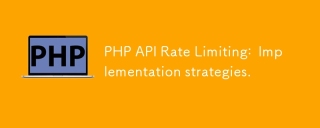
The article discusses strategies for implementing API rate limiting in PHP, including algorithms like Token Bucket and Leaky Bucket, and using libraries like symfony/rate-limiter. It also covers monitoring, dynamically adjusting rate limits, and hand
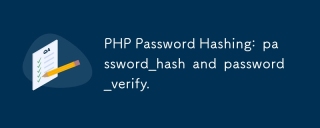
The article discusses the benefits of using password_hash and password_verify in PHP for securing passwords. The main argument is that these functions enhance password protection through automatic salt generation, strong hashing algorithms, and secur
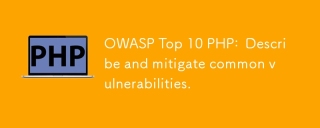
The article discusses OWASP Top 10 vulnerabilities in PHP and mitigation strategies. Key issues include injection, broken authentication, and XSS, with recommended tools for monitoring and securing PHP applications.
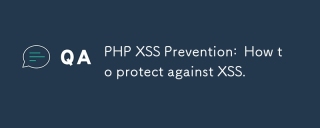
The article discusses strategies to prevent XSS attacks in PHP, focusing on input sanitization, output encoding, and using security-enhancing libraries and frameworks.

The article discusses the use of interfaces and abstract classes in PHP, focusing on when to use each. Interfaces define a contract without implementation, suitable for unrelated classes and multiple inheritance. Abstract classes provide common funct


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
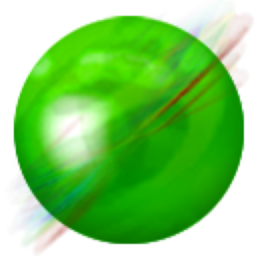
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
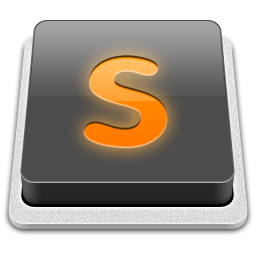
SublimeText3 Mac version
God-level code editing software (SublimeText3)

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
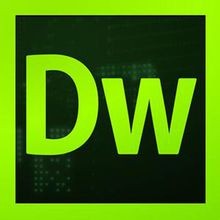
Dreamweaver CS6
Visual web development tools