In the Go language, a function is an organized, reusable code segment (block) used to implement a single specific or related function; its main purpose is to improve the modularity of the application and the efficiency of the code. Reuse, better code management, modular development. Functions usually use parameters and return values to interact with the caller; parameters pass data to the function, return values, and the function passes the processed data to the caller.
The operating environment of this tutorial: Windows 7 system, GO version 1.18, Dell G3 computer.
Function is an organized, reusable code segment used to implement a single or related function, which can improve the modularity of the application and the reuse rate of the code.
Function Overview
Function, function, independent code block used to implement specific functions. The main purpose is code reuse (reuse), better code management, and modular development. Functions usually use parameters and return values to interact with the caller. Parameters pass data to the function, return value, and the function passes the processed data to the caller. Functions in Go language are called first-class citizens. It means that it supports high-order functions, anonymous functions, closures and other features, and can meet advanced function features such as interfaces.
Function definition
Function constitutes the logical structure of code execution. In Go language, the basic composition of function is: keyword func, Function name, parameter list, return value, function body and return statement. Every program contains many functions, and functions are basic code blocks.
Syntax:
Definition:
func 函数名(形参列表)(返回值类型列表) { 函数体,通常会有return语句,返回值 }
Call:
函数名(实参列表)
Function name: The identifier of the function, used to find the function, and internally is an address pointing to the function code. Formal parameter list: composed of variables and types. Return value type list: the type of function return value. Multiple return values need to be specified. Function body: the specific statement that implements the function. return statement: return value statement
The above is a named function and cannot be defined inside other functions.
Function parameters
are used to pass data to the function when calling the function. Actual parameters, actual parameters. Parameters given when calling. Refers to parameters with specific actual data. Formal parameters, formal parameters. Parameters used when defining. It means that the function requires parameters, but the parameters do not have any actual data when they are defined. When calling, the process of assigning values to formal parameter variables using actual parameters occurs, which is called parameter passing. During the execution of the function, the formal parameters have specific data, and the formal parameters are equivalent to the variables declared within the function.
Parameter transfer is divided into two methods: value transfer and address transfer. When the address is passed, the formal parameters need to be defined as pointer types, and the address parameters need to be obtained when calling. Sample code:
func funcTest(p1 int, p2 *int) { p1++ *p2++ fmt.Println(p1, *p2) } func main() { var ( a1 = 42 a2 = 42 ) funcTest(a1, &a2) // 参数赋值过程 fmt.Println(a1, a2) }
The above will output
43 43 42 43
Value passing, the function will get a copy of the actual parameters. When the address is passed, the function will get the actual parameter address, so that the modification of the variable through the address in the function will also affect the actual parameters.
Go supports rest...an indefinite number of parameters. When defining, place the indefinite number of formal parameters at the end of the formal parameter list and use...Type method. Demonstration:
定义: func funcTest(op string, nums ...int) { fmt.Println(nums) // [4, 1, 55, 12], slice切片型数据 } 调用 funcTest("someOp", 4, 1, 55, 12)
Receive The parameter received is slice type.
Function return value
The return statement is used to generate a return value. The return value type needs to be determined when the function is defined, and multi-value returns are supported. Demonstration syntax:
func funcTest() (int, string) { return 42, "Hank" }
You can declare the returned variable when defining. This method is called named return, and the demonstration is:
func funcTest() (num int, title string) { num = 42 title = "Hank" return }
There is no need to return any data, just return it directly!
Function variables
Function can be regarded as a special pointer type, which can be saved in variables like other types. The function can be accessed through function identifiers and variables. The demonstration is as follows:
func funcTest() { fmt.Println("func() type") } func main() { fmt.Printf("%T, (%v)\n", funcTest, funcTest) fn := funcTest fmt.Printf("%T, (%v)\n", fn, fn) funcTest() fn() }
Execution result:
func(), (0x48fe20) func(), (0x48fe20) func() type func() type
It can be seen that the function identifier is a pointer to the function. Can be assigned to other variables.
Function parameters
Functions can also be used as parameters of other functions. The demonstration is as follows:
func funcSuccess() { } func funcAsync(handle func()) { // 调用函数参数 handle() } // 传递函数到其他函数 funcAsync(success)
This kind of callback The usage syntax of functions is very useful when dealing with asynchronous logic.
Anonymous functions
Anonymous functions can be defined. Anonymous functions can be saved to variables, passed as arguments, or called immediately. If a function is used temporarily, an anonymous function is a good choice. Example syntax:
赋值给变量 fn := func() { } fn() // 作为参数 someFunc(func() { }) // 立即调用 func() { }()
Closure
由于匿名函数可以定义在其他函数内,同时变量的作用域为层叠的,也就是匿名函数可以会访问其所在的外层函数内的局部变量。当外层函数运行结束后,匿名函数会与其使用的外部函数的局部变量形成闭包。示例代码:
var fn func() func outer() { v := 42 fn = func() { v ++ fmt.Print(v) } } outer() fn() // 43
此例中,fn 对应的匿名函数与 outer() 的局部变量 v,就形成了闭包。
函数调用示意图
var v = "global" func funcTest(v) { v = "funcTest" fmt.Println(v) } func main() { v := "main" funcTest(v) }
代码编译期间,会将函数代码存放在内存代码区。 函数被调用时,在运行期间会在函数运行栈区开辟函数栈,内部由局部变量标识符列表(就是局部变量),上层标识符列表引用等信息。直到运行结束,此空间才会被出栈,释放。 函数内部调用了新函数,新函数的执行空间入栈,要等到新函数执行空间出栈,调用他的函数才会被出栈。 以上代码的运行逻辑图如下:
递归调用
函数内部调用函数本身。称之为递归调用。示例代码:
func funcTest() { fmt.Println("run") funcTest() }
定义实现递归调用函数时,通常需要定义一个出口。用来确定何时不再进行递归调用了。一旦满足条件,则调用停止。例如:
func funcTest(v) { fmt.Println(v, "run") v ++ if v <= 10 { funcTest() } }
典型的应用有,树状菜单的处理,遍历目录,快速排序等。 递归调用的优势是编码简单,与描述的业务逻辑保持一致。
【相关推荐:Go视频教程】
The above is the detailed content of What does function mean in go language?. For more information, please follow other related articles on the PHP Chinese website!
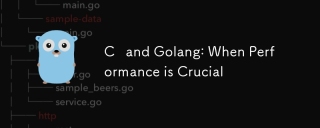
C is more suitable for scenarios where direct control of hardware resources and high performance optimization is required, while Golang is more suitable for scenarios where rapid development and high concurrency processing are required. 1.C's advantage lies in its close to hardware characteristics and high optimization capabilities, which are suitable for high-performance needs such as game development. 2.Golang's advantage lies in its concise syntax and natural concurrency support, which is suitable for high concurrency service development.
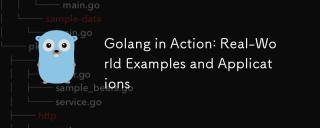
Golang excels in practical applications and is known for its simplicity, efficiency and concurrency. 1) Concurrent programming is implemented through Goroutines and Channels, 2) Flexible code is written using interfaces and polymorphisms, 3) Simplify network programming with net/http packages, 4) Build efficient concurrent crawlers, 5) Debugging and optimizing through tools and best practices.
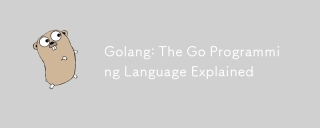
The core features of Go include garbage collection, static linking and concurrency support. 1. The concurrency model of Go language realizes efficient concurrent programming through goroutine and channel. 2. Interfaces and polymorphisms are implemented through interface methods, so that different types can be processed in a unified manner. 3. The basic usage demonstrates the efficiency of function definition and call. 4. In advanced usage, slices provide powerful functions of dynamic resizing. 5. Common errors such as race conditions can be detected and resolved through getest-race. 6. Performance optimization Reuse objects through sync.Pool to reduce garbage collection pressure.
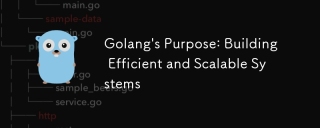
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
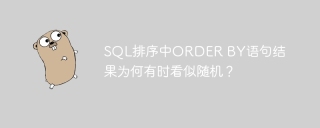
Confused about the sorting of SQL query results. In the process of learning SQL, you often encounter some confusing problems. Recently, the author is reading "MICK-SQL Basics"...
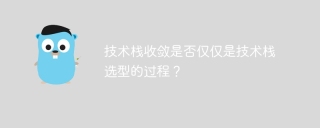
The relationship between technology stack convergence and technology selection In software development, the selection and management of technology stacks are a very critical issue. Recently, some readers have proposed...
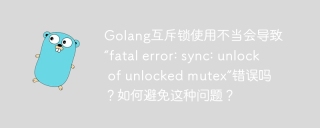
Golang ...
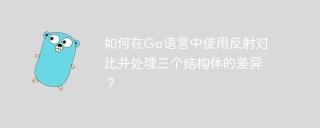
How to compare and handle three structures in Go language. In Go programming, it is sometimes necessary to compare the differences between two structures and apply these differences to the...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
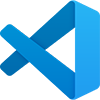
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
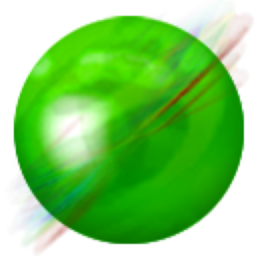
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
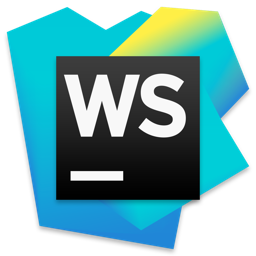
WebStorm Mac version
Useful JavaScript development tools