Method to find the maximum value of an array: 1. Math.max() is used with apply() to find the maximum value. The syntax is "Math.max.apply(null,array);"; 2. Math.max() is used together. Use the expansion operator "..." to find, the syntax is "Math.max(...array);"; 3. Use reduce() to find, the syntax is "array.reduce((a,b)=>{return a=a>b?a:b});".
The operating environment of this tutorial: Windows 7 system, ECMAScript version 6, Dell G3 computer.
Several ways to get the maximum value of an array
// 写法一: Math.max.apply(null, [14, 3, 77, 30]); // 写法二: Math.max(...[14, 3, 77, 30]); // 写法三:reduce [14,3,77,30].reduce((accumulator, currentValue)=>{ return accumulator = accumulator > currentValue ? accumulator : currentValue });
There are three ways to write, the first two use Math. The max()
method is implemented. The last one uses the reduce
API. The following will talk about how to use the basic Math.max()
method to achieve the maximum value of the array. , a detailed explanation of the application of the apply method in examples, as well as the introduction and use of reduce
API.
Math.max()
The first two writing methods use the Math.max()
method, which will be introduced here first Consider this method:
Math.max() function returns the maximum value in a set of numbers.
Usage:
Math.max(10, 20); // 20 Math.max(-10, -20); // -10 Math.max(-10, 20); // 20
Note that it is the maximum value in a group of numbers, not the maximum value of an array, from the above Usage We can see that the parameter is not an array, but a set of numbers separated by commas, so we cannot directly use this method to achieve the maximum value of the array, but make some improvements:
Usage of apply method and spread operator
##1. apply Method
Math.max() method to achieve the maximum value of the array. We can use the ES5 apply method to fulfill.
console.log(Math.max(1, 2, 344, 44, 2, 2, 333)); console.log(Math.max.call(null, 1, 2, 344, 44, 2, 2, 333)); console.log(Math.max.apply(null, [1, 2, 344, 44, 2, 2, 333]));
Math.max, and pass null as the first parameter. In this way, the use is the same as the original
Math.max(). Of course, the acquisition of the array has not yet been implemented. The maximum value, but this is the first and key step to understand how
Math.max.apply(null, [14, 3, 77, 30]); is written.
Math.max() uses a parameter list, that is, a set of numbers. Our target is an array. We mentioned the call method earlier, so the apply method can be used here. The difference is: the
apply() method accepts a parameter array to achieve our needs.
Math.max, pass null as the first parameter, and pass an array as the second parameter. This meets our needs.
2. Use of expansion operators
We talked about using the apply method forMath.max() To realize the function, in fact, when we implemented the apply method by hand, we did special processing on the parameter issue. How to deconstruct the incoming parameter array into a parameter list inside apply? Applying ES6, we The spread operator is used.
Math.max()Using the spread operator is to obtain the maximum value of the array Another way to implement values, which also makes it easier to get the maximum value in an array:
var arr = [1, 2, 3]; var max = Math.max(...arr); // 3
reduce
Use the reduce API to obtain the maximum value of the array:
// reduce [14,3,77,30].reduce((accumulator, currentValue)=>{ return accumulator = accumulator > currentValue ? accumulator : currentValue });
Detailed explanation of reduce method parameters
This article would like to talk about how to use the reduce APIThe reduce() method executes a reducer function provided by you for each element in the array ( executed in ascending order), summarizing its results into a single return value.It is important to note the parameters of this method:
arr.reduce(callback(accumulator, currentValue[, index[, array]])[, initialValue])To summarize, the reduce method has two parameters, one is callback, which is the reducer function you define, and the other is initialValue That is the initial value. In most cases, we may not use the initialValue parameter and only use callback. However, the initialValue parameter is very relevant to the reduce method, so we must know what the initialValue parameter is for. , and how other parameters are used. Let’s talk about the two parameters callback and initialValue in detail.
callback:
callback 执行数组中每个值 (如果没有提供 initialValue则第一个值除外)的函数,注意这个:如果没有提供 initialValue则第一个值除外,你会发现initialValue在reduce方法中比较关键,如果搞不清楚initialValue参数的意义,几乎很难去运用reduce方法。
callback函数又有四个参数,其中前三个参数也是十分关键的,和initialValue参数一样,需要搞清楚含义,分别是:accumulator,currentValue 和 index。
之所以说清楚reduce方法的几个关键参数是非常关键的,主要就在于,initialValue初始值有和没有这两种情况下,callback的三个参数(accumulator,currentValue 和 index)是不一样的。
initialValue:
initialValue参数 可选,这个参数作为第一次调用 callback函数时的第一个参数的值。 如果没有提供初始值,则将使用数组中的第一个元素。 在没有初始值的空数组上调用 reduce 将报错。
下面讲方法在执行过程中,callback的三个参数(accumulator,currentValue 和 index)是如何不一样的:
回调函数第一次执行时,accumulator 和currentValue的取值有两种情况:如果调用reduce()时提供了initialValue,accumulator取值为initialValue,currentValue取数组中的第一个值;如果没有提供 initialValue,那么accumulator取数组中的第一个值,currentValue取数组中的第二个值。
注意:如果没有提供initialValue,reduce 会从索引1的地方开始执行 callback 方法,跳过第一个索引。如果提供initialValue,从索引0开始。
这也是index参数里描述的:index 可选。是数组中正在处理的当前元素的索引。 如果提供了initialValue,则起始索引号为0,否则从索引1起始。
reduce方法如何运行
1.无初始值的情况
假如运行下段reduce()代码:
[0, 1, 2, 3, 4].reduce(function(accumulator, currentValue, currentIndex, array){ return accumulator + currentValue; });
callback 被调用四次,每次调用的参数和返回值如下表:
由reduce返回的值将是最后一次回调返回值(10)。
你还可以使用箭头函数来代替完整的函数。 下面的代码将产生与上面的代码相同的输出:
[0, 1, 2, 3, 4].reduce((prev, curr) => prev + curr );
2.有初始值的情况
如果你打算提供一个初始值作为reduce()方法的第二个参数,以下是运行过程及结果:
[0, 1, 2, 3, 4].reduce((accumulator, currentValue, currentIndex, array) => { return accumulator + currentValue }, 10)
这种情况下reduce()返回的值是20。
reduce使用场景
reduce使用场景1.将二维数组转化为一维
var flattened = [[0, 1], [2, 3], [4, 5]].reduce( function(a, b) { return a.concat(b); }, [] ); // flattened is [0, 1, 2, 3, 4, 5]
写成箭头函数的形式:
var flattened = [[0, 1], [2, 3], [4, 5]].reduce( ( acc, cur ) => acc.concat(cur), [] );
注意!!!上面这个例子,有初始值,初始值是一个空数组[]
。
concat()方法介绍:
concat() 方法用于合并两个或多个数组。
const array1 = ['a', 'b', 'c']; const array2 = ['d', 'e', 'f']; const array3 = array1.concat(array2); console.log(array3); // expected output: Array ["a", "b", "c", "d", "e", "f"]
连接两个数组
以下代码将两个数组合并为一个新数组:
var alpha = ['a', 'b', 'c']; var numeric = [1, 2, 3]; alpha.concat(numeric); // result in ['a', 'b', 'c', 1, 2, 3]
连接三个数组
以下代码将三个数组合并为一个新数组:
var num1 = [1, 2, 3], num2 = [4, 5, 6], num3 = [7, 8, 9]; var nums = num1.concat(num2, num3); console.log(nums); // results in [1, 2, 3, 4, 5, 6, 7, 8, 9]
reduce使用场景2.数组里所有值的和
var sum = [0, 1, 2, 3].reduce(function (accumulator, currentValue) { return accumulator + currentValue; }, 0); // 和为 6
写成箭头函数的形式:
var total = [ 0, 1, 2, 3 ].reduce( ( acc, cur ) => acc + cur, 0 );
注意,这里设置了初始值,为0,如果不这个初始值会怎么样呢?数组为空的时候,会抛错TypeError,再看一遍下面的描述:
如果数组为空且没有提供initialValue,会抛出TypeError 。如果数组仅有一个元素(无论位置如何)并且没有提供initialValue, 或者有提供initialValue但是数组为空,那么此唯一值将被返回并且callback不会被执行。
所以,在使用reduce时我们可以先判断一下数组是否为空,来避免这个问题。
【相关推荐:javascript视频教程、编程视频】
The above is the detailed content of How to find the maximum value of an array in es6. For more information, please follow other related articles on the PHP Chinese website!

TonavigateReact'scomplexecosystemeffectively,understandthetoolsandlibraries,recognizetheirstrengthsandweaknesses,andintegratethemtoenhancedevelopment.StartwithcoreReactconceptsanduseState,thengraduallyintroducemorecomplexsolutionslikeReduxorMobXasnee

Reactuseskeystoefficientlyidentifylistitemsbyprovidingastableidentitytoeachelement.1)KeysallowReacttotrackchangesinlistswithoutre-renderingtheentirelist.2)Chooseuniqueandstablekeys,avoidingarrayindices.3)Correctkeyusagesignificantlyimprovesperformanc

KeysinReactarecrucialforoptimizingtherenderingprocessandmanagingdynamiclistseffectively.Tospotandfixkey-relatedissues:1)Adduniquekeystolistitemstoavoidwarningsandperformanceissues,2)Useuniqueidentifiersfromdatainsteadofindicesforstablekeys,3)Ensureke

React's one-way data binding ensures that data flows from the parent component to the child component. 1) The data flows to a single, and the changes in the state of the parent component can be passed to the child component, but the child component cannot directly affect the state of the parent component. 2) This method improves the predictability of data flows and simplifies debugging and testing. 3) By using controlled components and context, user interaction and inter-component communication can be handled while maintaining a one-way data stream.

KeysinReactarecrucialforefficientDOMupdatesandreconciliation.1)Choosestable,unique,andmeaningfulkeys,likeitemIDs.2)Fornestedlists,useuniquekeysateachlevel.3)Avoidusingarrayindicesorgeneratingkeysdynamicallytopreventperformanceissues.

useState()iscrucialforoptimizingReactappperformanceduetoitsimpactonre-rendersandupdates.Tooptimize:1)UseuseCallbacktomemoizefunctionsandpreventunnecessaryre-renders.2)EmployuseMemoforcachingexpensivecomputations.3)Breakstateintosmallervariablesformor

Use Context and useState to share states because they simplify state management in large React applications. 1) Reduce propdrilling, 2) The code is clearer, 3) It is easier to manage global state. However, pay attention to performance overhead and debugging complexity. The rational use of Context and optimization technology can improve the efficiency and maintainability of the application.

Using incorrect keys can cause performance issues and unexpected behavior in React applications. 1) The key is a unique identifier of the list item, helping React update the virtual DOM efficiently. 2) Using the same or non-unique key will cause list items to be reordered and component states to be lost. 3) Using stable and unique identifiers as keys can optimize performance and avoid full re-rendering. 4) Use tools such as ESLint to verify the correctness of the key. Proper use of keys ensures efficient and reliable React applications.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
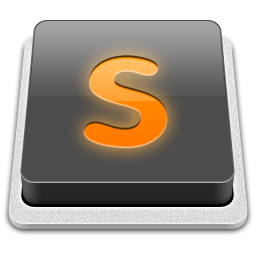
SublimeText3 Mac version
God-level code editing software (SublimeText3)

Zend Studio 13.0.1
Powerful PHP integrated development environment

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

SublimeText3 English version
Recommended: Win version, supports code prompts!
