The equal sign arrow "=>" in es6 refers to the arrow function, which is an abbreviation of a function. The syntax is (parameter)=>{function body};". The syntax of arrow function expression More concise than function expressions, there is no binding of this, super, arguments and new.target, it cannot be called through the new keyword, and the binding of this cannot be changed.
The operating environment of this tutorial: Windows 7 system, ECMAScript version 6, Dell G3 computer.
=>
is an arrow function, which is a new function added in the ES6 standard .As the name suggests, arrow functions are a new syntax that uses arrows (=>) to define functions. The syntax of arrow function expressions is more concise than function expressions, but it is slightly different from traditional JavaScript functions, mainly focusing on the following aspects. :
There is no binding of this, super, arguments and new.target, and its value is determined by the nearest non-arrow function in the periphery
Cannot Called through the new keyword
No prototype
The binding of this cannot be changed
The arguments object is not supported
Duplicate named parameters are not supported
The point of this in the function body always points to the object in which it is defined, and It will not point to the object that calls it. We know who executes the function in es5 and who it points to.
Execution Environment
You can learn and try it yourself, you can simply copy the sample program code to your browser console. Now, it is recommended to use Firefox(22) developer tools, Firefox(22) development The browser tool now supports arrow functions, and you can also use Google Chrome. If you use Google Chrome, you have to do the following two things:
In the address bar in Google Chrome Enter: about:flags, find the "Use experiential JavaScript" option and enable it.
Add use strict at the beginning of the function, and then test the arrow in your Google browser Function bar (tip: please use Google Chrome v38, I was fooled by the browser version at the time):
(function(){ "use strict"; // use arrow functions here }());
Fortunately, more and more browsers will support it in the future. ES6 features. Now that you have completed all the preparations, let’s continue to dive into it!
A new topic
People are discussing recently about A topic in ES6: About arrow functions, like this:
=>
New syntax
With the discussion, a new syntax was born:
param => expression
The new syntax is used on variables. Multiple variables can be declared in expressions. The following is the usage pattern of arrow functions:
// 一个参数对应一个表达式 param => expression;// 例如 x => x+2; // 多个参数对应一个表达式 (param [, param]) => expression; //例如 (x,y) => (x + y); // 一个参数对应多个表示式 param => {statements;} //例如 x = > { x++; return x;}; // 多个参数对应多个表达式 ([param] [, param]) => {statements} // 例如 (x,y) => { x++;y++;return x*y;}; //表达式里没有参数 () => expression; //例如var flag = (() => 2)(); flag等于2 () => {statements;} //例如 var flag = (() => {return 1;})(); flag就等于1 //传入一个表达式,返回一个对象 ([param]) => ({ key: value }); //例如 var fuc = (x) => ({key:x}) var object = fuc(1); alert(object);//{key:1}
Arrow function How is it implemented
We can convert an ordinary function into an arrow function to implement it:
// 当前函数 var func = function (param) { return param.split(" "); } // 利用箭头函数实现 var func = param => param.split(" ");
From the above example we can It can be seen that the syntax of the arrow function actually returns a new function, which has a function body and parameters.
Therefore, we can call the function we just created like this:
func("Felipe Moura"); // returns ["Felipe", "Moura"]
Immediate execution function (IIFE)
You Arrow functions can be used in immediate execution functions, for example:
( x => x * 2 )( 3 ); // 6
This line of code generates a temporary function. This function has a formal parameter x, and the return value of the function is x*2, and the system will execute it immediately. This temporary function assigns 3 to the formal parameter x.
The following example describes the situation where there are multiple lines of code in the temporary function body:
( (x, y) => { x = x * 2; return x + y; })( 3, "A" ); // "6A"
Related thoughts
Consider the following function:
var func = x => { return x++; };
We have listed some common problems:
The arrow function creates a temporary function whose arguments will not be Settings:
console.log(arguments); // not defined
typeof
and instanceof function
can also check temporary functions normally:
func instanceof Function; // true typeof func; // function func.constructor == Function; // true
Placing arrow functions within brackets is invalid :
// 有效的常规语法 (function (x, y){ x= x * 2; return x + y; } (3, "B") ); // 无效的箭头函数语法 ( (x, y) => { x= x * 2; return x + y; } ( 3, "A" ) ); // 但是可以这样写就是有效的了: ( (x,y) => { x= x * 2;return x + y; } )( 3,"A" );//立即执行函数
Although the arrow function will generate a temporary function, this temporary function is not a constructor:
var instance= new func(); // TypeError: func is not a constructor
There is also no prototype object:
func.prototype; // undefined
Scope
The scope of this arrow function is somewhat different from other functions. If it is not in strict mode, the this keyword points to window, and in strict mode it is undefined, in the constructor This points to the current object instance. If this is within a function of an object, this points to the object. This may point to a DOM element. For example, when we add an event listening function, this may point to something other than It's very straightforward. In fact, the pointing of this (not just this variable) variables is judged according to a rule: scope flow. Below I will demonstrate how this appears in the event listening function and in the object function:
In the event listening function:
document.body.addEventListener('click', function(evt){ console.log(this); // the HTMLBodyElement itself });
In the constructor:
function Person () { let fullName = null; this.getName = function () { return fullName; }; this.setName = function (name) { fullName = name; return this; }; } let jon = new Person(); jon.setName("Jon Doe"); console.log(jon.getName()); // "Jon Doe" //注:this关键字这里就不解释了,大家自己google,baidu吧。
In In this example, if we let the Person.setName function return the Person object itself, we can use it like this:
jon.setName("Jon Doe") .getName(); // "Jon Doe"
In an object:
let obj = { foo: "bar", getIt: function () { return this.foo; } }; console.log( obj.getIt() ); // "bar"
但是当执行流(比如使用了setTimeout)和作用域变了的时候,this也会变。
function Student(data){ this.name = data.name || "Jon Doe"; this.age = data.age>=0 ? data.age : -1; this.getInfo = function () { return this.name + ", " + this.age; }; this.sayHi = function () { window.setTimeout( function () { console.log( this ); }, 100 ); } } let mary = new Student({ name: "Mary Lou", age: 13 }); console.log( mary.getInfo() ); // "Mary Lou, 13" mary.sayHi(); // window
当setTimeout函数改变了执行流的情况时,this的指向会变成全局对象,或者是在严格模式下就是undefine,这样在setTimeout函数里面我们使用其他的变量去指向this对象,比如self,that,当然不管你用什么变量,你首先应该在setTimeout访问之前,给self,that赋值,或者使用bind方法不然这些变量就是undefined。
这是后就是箭头函数登场的时候了,它可以保持作用域,this的指向就不会变了。
让我们看下上文起先的例子,在这里我们使用箭头函数:
function Student(data){ this.name = data.name || "Jon Doe"; this.age = data.age>=0 ? data.age : -1; this.getInfo = function () { return this.name + ", " + this.age; }; this.sayHi = function () { window.setTimeout( ()=>{ // the only difference is here console.log( this ); }, 100 ); } } let mary = new Student({ name: "Mary Lou", age: 13 }); console.log( mary.getInfo() ); // "Mary Lou, 13" mary.sayHi(); // Object { name: "Mary Lou", age: 13, ... }
分析:在sayHi函数中,我们使用了箭头函数,当前作用域是在student对象的一个方法中,箭头函数生成的临时函数的作用域也就是student对象的sayHi函数的作用域。所以即使我们在setTimeout调用了箭头函数生成的临时函数,这个临时函数中的this也是正确的指向。
有趣和有用的使用
创建一个函数很容易,我们可以利用它可以保持作用域的特征:
例如我们可以这么使用:Array.forEach()
var arr = ['a', 'e', 'i', 'o', 'u']; arr.forEach(vowel => { console.log(vowel); });
分析:在forEach里箭头函数会创建并返回一个临时函数 tempFun,这个tempFun你可以想象成这样的:function(vowel){ console.log(vowel);}但是Array.forEach函数会怎么去处理传入的tempFunc呢?在forEach函数里会这样调用它:tempFunc.call(this,value);所有我们看到函数的正确执行效果。
//在Array.map里使用箭头函数,这里我就不分析函数执行过程了。。。。 var arr = ['a', 'e', 'i', 'o', 'u']; arr.map(vowel => { return vowel.toUpperCase(); }); // [ "A", "E", "I", "O", "U" ]
费布拉奇数列
var factorial = (n) => { if(n==0) { return 1; } return (n * factorial (n-1) ); } factorial(6); // 720
我们也可以用在Array.sort方法里:
let arr = ['a', 'e', 'i', 'o', 'u']; arr.sort( (a, b)=> a < b? 1: -1 );
也可以在事件监听函数里使用:
// EventObject, BodyElement document.body.addEventListener('click', event=>console.log(event, this));
总结
尽管大家可能会认为使用箭头函数会降低你代码的可读性,但是由于它对作用域的特殊处理,它能让我们能很好的处理this的指向问题。箭头函数加上let关键字的使用,将会让我们JavaScript代码上一个层次!尽量多使用箭头函数,你可以再你的浏览器测试你写的箭头函数代码,大家可以再评论区留下你对箭头函数的想法和使用方案!我希望大家能享受这篇文章,就像你会不就的将来享受箭头函数带给你的快乐.
更多编程相关知识,请访问:编程入门!!
The above is the detailed content of What does the es6 equal sign arrow mean?. For more information, please follow other related articles on the PHP Chinese website!

React'slimitationsinclude:1)asteeplearningcurveduetoitsvastecosystem,2)SEOchallengeswithclient-siderendering,3)potentialperformanceissuesinlargeapplications,4)complexstatemanagementasappsgrow,and5)theneedtokeepupwithitsrapidevolution.Thesefactorsshou

Reactischallengingforbeginnersduetoitssteeplearningcurveandparadigmshifttocomponent-basedarchitecture.1)Startwithofficialdocumentationforasolidfoundation.2)UnderstandJSXandhowtoembedJavaScriptwithinit.3)Learntousefunctionalcomponentswithhooksforstate

ThecorechallengeingeneratingstableanduniquekeysfordynamiclistsinReactisensuringconsistentidentifiersacrossre-rendersforefficientDOMupdates.1)Usenaturalkeyswhenpossible,astheyarereliableifuniqueandstable.2)Generatesynthetickeysbasedonmultipleattribute

JavaScriptfatigueinReactismanageablewithstrategieslikejust-in-timelearningandcuratedinformationsources.1)Learnwhatyouneedwhenyouneedit,focusingonprojectrelevance.2)FollowkeyblogsliketheofficialReactblogandengagewithcommunitieslikeReactifluxonDiscordt

TotestReactcomponentsusingtheuseStatehook,useJestandReactTestingLibrarytosimulateinteractionsandverifystatechangesintheUI.1)Renderthecomponentandcheckinitialstate.2)Simulateuserinteractionslikeclicksorformsubmissions.3)Verifytheupdatedstatereflectsin

KeysinReactarecrucialforoptimizingperformancebyaidinginefficientlistupdates.1)Usekeystoidentifyandtracklistelements.2)Avoidusingarrayindicesaskeystopreventperformanceissues.3)Choosestableidentifierslikeitem.idtomaintaincomponentstateandimproveperform

Reactkeysareuniqueidentifiersusedwhenrenderingliststoimprovereconciliationefficiency.1)TheyhelpReacttrackchangesinlistitems,2)usingstableanduniqueidentifierslikeitemIDsisrecommended,3)avoidusingarrayindicesaskeystopreventissueswithreordering,and4)ens

UniquekeysarecrucialinReactforoptimizingrenderingandmaintainingcomponentstateintegrity.1)Useanaturaluniqueidentifierfromyourdataifavailable.2)Ifnonaturalidentifierexists,generateauniquekeyusingalibrarylikeuuid.3)Avoidusingarrayindicesaskeys,especiall


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

SublimeText3 English version
Recommended: Win version, supports code prompts!

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
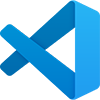
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
