Home >Web Front-end >Front-end Q&A >What are parent-child components in react
In the mutual calling of react components, the caller is called the parent component and the callee is called the child component. Values can be passed between parent and child components: 1. When a parent component passes a value to a child component, the value to be passed is first passed to the child component, and then in the child component, props are used to receive the value passed by the parent component; 2. Child component When passing values to the parent component, you need to pass them to the parent component through the trigger method.
#The operating environment of this tutorial: Windows7 system, react18 version, Dell G3 computer.
The react component is a self-defined non-html tag. It is stipulated that the first letter of the react component is capitalized
:
class App extends Component{ } <app></app>
In the mutual calling of components, the caller
is called the parent component, and the callee
Called a subcomponent:
import React from 'react'; import Children from './Children'; class Up extends React.Component { constructor(props){ super(props); this.state = { } } render(){ console.log("render"); return( <div> up <children></children> </div> ) } } export default Up;
import React from 'react'; class Children extends React.Component{ constructor(props){ super(props); this.state = { } } render(){ return ( <div> Children </div> ) } } export default Children;
Parent component passes value to subcomponent using props. When a parent component passes a value to a child component, the value to be passed is first passed to the child component, and then in the child component, props are used to receive the value passed by the parent component.
The parent component defines a property when calling the child component:
<children></children>
This value msg
will be bound to the props
property of the child component , subcomponents can be used directly:
this.props.msg
The parent component can pass values and methods to the component, and can even pass itself to the subcomponent
import React from 'react'; import Children from './Children'; class Up extends React.Component { constructor(props){ super(props); this.state = { } } render(){ console.log("render"); return( <div> up <Children msg="父组件传值给子组件" /> </div> ) } } export default Up;
import React from 'react'; class Children extends React.Component{ constructor(props){ super(props); this.state = { } } render(){ return ( <div> Children <br> {this.props.msg} </div> ) } } export default Children;
import React from 'react'; import Children from './Children'; class Up extends React.Component { constructor(props){ super(props); this.state = { } } run = () => { console.log("父组件run方法"); } render(){ console.log("render"); return(up <children></children>) } } export default Up;
import React from 'react'; class Children extends React.Component{ constructor(props){ super(props); this.state = { } } run = () => { this.props.run(); } render(){ return ( <div> Children <br> <button>Run</button> </div> ) } } export default Children;
import React from 'react'; import Children from './Children'; class Up extends React.Component { constructor(props){ super(props); this.state = { } } run = () => { console.log("父组件run方法"); } render(){ console.log("render"); return( <div> up <Children msg={this}/> </div> ) } } export default Up;
import React from 'react'; class Children extends React.Component{ constructor(props){ super(props); this.state = { } } run = () => { console.log(this.props.msg); } render(){ return ( <div> Children <br> <button>Run</button> </div> ) } } export default Children;
import React from 'react'; import Children from './Children'; class Up extends React.Component { constructor(props){ super(props); this.state = { } } getChildrenData = (data) => { console.log(data); } render(){ console.log("render"); return(up <children></children>) } } export default Up;
import React from 'react'; class Children extends React.Component{ constructor(props){ super(props); this.state = { } } render(){ return ( <div> Children <br> <button> {this.props.upFun("子组件数据")}}>Run</button> </div> ) } } export default Children;
import React from 'react'; import Children from './Children'; class Up extends React.Component { constructor(props){ super(props); this.state = { } } clickButton = () => { console.log(this.refs.children); } render(){ console.log("render"); return(up <children></children>) } } export default Up; ``` ```js import React from 'react'; class Children extends React.Component{ constructor(props){ super(props); this.state = { title: "子组件" } } runChildren = () => { } render(){ return (Children) } } export default Children; ``` 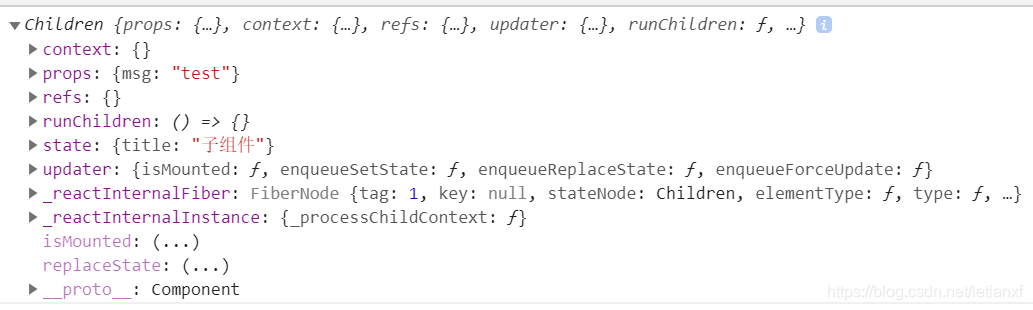
Redis video tutorial】
The above is the detailed content of What are parent-child components in react. For more information, please follow other related articles on the PHP Chinese website!