Javascript operators can be divided into: 1. Arithmetic operators, used to perform common mathematical operations; 2. Assignment operators, used to assign values to variables; 3. String operators; 4. Auto-increment , decrement operator; 5. comparison operator; 6. logical operator; 7. ternary operator; 8. bitwise operator.
The operating environment of this tutorial: windows7 system, javascript version 1.8.5, Dell G3 computer.
Arithmetic operators
Arithmetic operators are used to perform common mathematical operations, such as addition, subtraction, multiplication, division, etc., as shown in the table below The arithmetic operators supported in JavaScript are listed in:
Operator | Description | Example |
---|---|---|
Addition operator | x y means calculating the sum of x plus y | |
- | Subtraction Operator | x - y means to calculate the difference of x minus y |
* | Multiplication operator | x * y means Calculate the product of x times y |
/ | Division operator | x / y means to calculate the quotient of x divided by y |
% | Modulo (remainder) operator | x % y means calculating the remainder of x divided by y |
The sample code is as follows:
var x = 10, y = 4; console.log("x + y =", x + y); // 输出:x + y = 14 console.log("x - y =", x - y); // 输出:x - y = 6 console.log("x * y =", x * y); // 输出:x * y = 40 console.log("x / y =", x / y); // 输出:x / y = 2.5 console.log("x % y =", x % y); // 输出:x % y = 2
var x = 10, y = 4; console.log("x + y =", x + y); // 输出:x + y = 14 console.log("x - y =", x - y); // 输出:x - y = 6 console.log("x * y =", x * y); // 输出:x * y = 40 console.log("x / y =", x / y); // 输出:x / y = 2.5 console.log("x % y =", x % y); // 输出:x % y = 2
In the above code, the content in double quotes is a string , so the operators will be output as they are and will not participate in the operation.
Assignment operator
The assignment operator is used to assign values to variables. The following table lists the assignment operations supported in JavaScript. Symbols:
Description | Example | |
---|---|---|
The simplest assignment operator, assigns the value on the right side of the operator to the variable on the left side of the operator | x = 10 means assigning the variable x to 10 | |
Perform the addition operation first, and then assign the result to the variable on the left side of the operator | x = y is equivalent to x = x y | |
Perform subtraction first, and then assign the result to the variable on the left side of the operator | x -= y is equivalent to x = x - y | |
Perform multiplication first, and then assign the result to the variable on the left side of the operator | x *= y is equivalent to x = x * y | |
Perform the division operation first, and then assign the result to the variable on the left side of the operator | x /= y is equivalent to x = x / y | |
First perform the modulo operation, and then assign the result to the variable on the left side of the operator | x %= y is equivalent to x = x % y |
复制纯文本复制
var x = 10; x += 20; console.log(x); // 输出:30 var x = 12, y = 7; x -= y; console.log(x); // 输出:5 x = 5; x *= 25; console.log(x); // 输出:125 x = 50; x /= 10; console.log(x); // 输出:5 x = 100; x %= 15; console.log(x); // 输出:10
var x = 10; x += 20; console.log(x); // 输出:30 var x = 12, y = 7; x -= y; console.log(x); // 输出:5 x = 5; x *= 25; console.log(x); // 输出:125 x = 50; x /= 10; console.log(x); // 输出:5 x = 100; x %= 15; console.log(x); // 输出:10
String operatorsThe
and =
operators in JavaScript can perform mathematical operations in addition to , can also be used to splice strings, where:
- operator means splicing the strings on the left and right sides of the operator together;
## The
# = operator means to concatenate strings first, and then assign the result to the variable on the left side of the operator. -
The sample code is as follows:
复制纯文本复制
var x = "Hello "; var y = "World!"; var z = x + y; console.log(z); // 输出:Hello World! x += y; console.log(x); // 输出:Hello World!
var x = "Hello "; var y = "World!"; var z = x + y; console.log(z); // 输出:Hello World! x += y; console.log(x); // 输出:Hello World!
The auto-increment and auto-decrement operators are used to perform auto-increment (1) and auto-decrement (-1) operations on the value of a variable. They are listed in the following table. Increment and decrement operators supported in JavaScript:
Influence | x | ||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
Increments x by 1 and returns the value of x | x | ||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||
Returns the value of x, and then adds 1 to x | --x | ||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||
Decrease x by 1, and then return the value of x | x-- | ||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||
Return the value of x, Then reduce x by 1 |
运算符 | 名称 | 示例 |
---|---|---|
== | 等于 | x == y 表示如果 x 等于 y,则为真 |
=== | 全等 | x === y 表示如果 x 等于 y,并且 x 和 y 的类型也相同,则为真 |
!= | 不相等 | x != y 表示如果 x 不等于 y,则为真 |
!== | 不全等 | x !== y 表示如果 x 不等于 y,或者 x 和 y 的类型不同,则为真 |
小于 | x | |
> | 大于 | x > y 表示如果 x 大于 y,则为真 |
>= | 大于或等于 | x >= y 表示如果 x 大于或等于 y,则为真 |
小于或等于 | x |
示例代码如下:
var x = 25; var y = 35; var z = "25"; console.log(x == z); // 输出: true console.log(x === z); // 输出: false console.log(x != y); // 输出: true console.log(x !== z); // 输出: true console.log(x < y); // 输出: true console.log(x > y); // 输出: false console.log(x <= y); // 输出: true console.log(x >= y); // 输出: false
var x = 25; var y = 35; var z = "25"; console.log(x == z); // 输出: true console.log(x === z); // 输出: false console.log(x != y); // 输出: true console.log(x !== z); // 输出: true console.log(x < y); // 输出: true console.log(x > y); // 输出: false console.log(x <= y); // 输出: true console.log(x >= y); // 输出: false
逻辑运算符
逻辑运算符通常用来组合多个表达式,逻辑运算符的运算结果是一个布尔值,只能有两种结果,不是 true 就是 false。下表中列举了 JavaScript 中支持的逻辑运算符:
运算符 | 名称 | 示例 |
---|---|---|
&& | 逻辑与 | x && y 表示如果 x 和 y 都为真,则为真 |
|| | 逻辑或 | x || y 表示如果 x 或 y 有一个为真,则为真 |
! | 逻辑非 | !x 表示如果 x 不为真,则为真 |
示例代码如下:
var year = 2021; // 闰年可以被 400 整除,也可以被 4 整除,但不能被 100 整除 if((year % 400 == 0) || ((year % 100 != 0) && (year % 4 == 0))){ console.log(year + " 年是闰年。"); } else{ console.log(year + " 年是平年。"); }
var year = 2021; // 闰年可以被 400 整除,也可以被 4 整除,但不能被 100 整除 if((year % 400 == 0) || ((year % 100 != 0) && (year % 4 == 0))){ console.log(year + " 年是闰年。"); } else{ console.log(year + " 年是平年。"); }
三元运算符
三元运算符(也被称为条件运算符),由一个问号和一个冒号组成,语法格式如下:
条件表达式 ? 表达式1 : 表达式2 ;
如果“条件表达式”的结果为真(true),则执行“表达式1”中的代码,否则就执行“表达式2”中的代码。
示例代码如下:
var x = 11, y = 20; x > y ? console.log("x 大于 y") : console.log("x 小于 y"); // 输出:x 小于 y
var x = 11, y = 20; x > y ? console.log("x 大于 y") : console.log("x 小于 y"); // 输出:x 小于 y
位运算符
位运算符用来对二进制位进行操作,JavaScript 中支持的位运算符如下表所示:
运算符 | 描述 | 示例 |
---|---|---|
& | 按位与:如果对应的二进制位都为 1,则该二进制位为 1 | 5 & 1 等同于 0101 & 0001 结果为 0001,十进制结果为 1 |
| | 按位或:如果对应的二进制位有一个为 1,则该二进制位为 1 | 5 | 1 等同于 0101 | 0001 结果为 0101,十进制结果为 5 |
^ | 按位异或:如果对应的二进制位只有一个为 1,则该二进制位为 1 | 5 ^ 1 等同于 0101 ^ 0001 结果为 0100,十进制结果为 4 |
~ | 按位非:反转所有二进制位,即 1 转换为 0,0 转换为 1 | ~5 等同于 ~0101 结果为 1010,十进制结果为 -6 |
按位左移:将所有二进制位统一向左移动指定的位数,并在最右侧补 0 | 5 | |
>> | 按位右移(有符号右移):将所有二进制位统一向右移动指定的位数,并拷贝最左侧的位来填充左侧 | 5 >> 1 等同于 0101 >> 1 结果为 0010,十进制结果为 2 |
>>> | 按位右移零(无符号右移):将所有二进制位统一向右移动指定的位数,并在最左侧补 0 | 5 >>> 1 等同于 0101 >>> 1 结果为 0010,十进制结果为 2 |
示例代码如下:
var a = 5 & 1, b = 5 | 1, c = 5 ^ 1, d = ~ 5, e = 5 << 1, f = 5 >> 1, g = 5 >>> 1; console.log(a); // 输出:1 console.log(b); // 输出:5 console.log(c); // 输出:4 console.log(d); // 输出:-6 console.log(e); // 输出:10 console.log(f); // 输出:2 console.log(g); // 输出:2
var a = 5 & 1, b = 5 | 1, c = 5 ^ 1, d = ~ 5, e = 5 << 1, f = 5 >> 1, g = 5 >>> 1; console.log(a); // 输出:1 console.log(b); // 输出:5 console.log(c); // 输出:4 console.log(d); // 输出:-6 console.log(e); // 输出:10 console.log(f); // 输出:2 console.log(g); // 输出:2
【推荐学习:javascript高级教程】
The above is the detailed content of What javascript operators can be divided into. For more information, please follow other related articles on the PHP Chinese website!

HTML and React can be seamlessly integrated through JSX to build an efficient user interface. 1) Embed HTML elements using JSX, 2) Optimize rendering performance using virtual DOM, 3) Manage and render HTML structures through componentization. This integration method is not only intuitive, but also improves application performance.

React efficiently renders data through state and props, and handles user events through the synthesis event system. 1) Use useState to manage state, such as the counter example. 2) Event processing is implemented by adding functions in JSX, such as button clicks. 3) The key attribute is required to render the list, such as the TodoList component. 4) For form processing, useState and e.preventDefault(), such as Form components.

React interacts with the server through HTTP requests to obtain, send, update and delete data. 1) User operation triggers events, 2) Initiate HTTP requests, 3) Process server responses, 4) Update component status and re-render.

React is a JavaScript library for building user interfaces that improves efficiency through component development and virtual DOM. 1. Components and JSX: Use JSX syntax to define components to enhance code intuitiveness and quality. 2. Virtual DOM and Rendering: Optimize rendering performance through virtual DOM and diff algorithms. 3. State management and Hooks: Hooks such as useState and useEffect simplify state management and side effects handling. 4. Example of usage: From basic forms to advanced global state management, use the ContextAPI. 5. Common errors and debugging: Avoid improper state management and component update problems, and use ReactDevTools to debug. 6. Performance optimization and optimality

Reactisafrontendlibrary,focusedonbuildinguserinterfaces.ItmanagesUIstateandupdatesefficientlyusingavirtualDOM,andinteractswithbackendservicesviaAPIsfordatahandling,butdoesnotprocessorstoredataitself.

React can be embedded in HTML to enhance or completely rewrite traditional HTML pages. 1) The basic steps to using React include adding a root div in HTML and rendering the React component via ReactDOM.render(). 2) More advanced applications include using useState to manage state and implement complex UI interactions such as counters and to-do lists. 3) Optimization and best practices include code segmentation, lazy loading and using React.memo and useMemo to improve performance. Through these methods, developers can leverage the power of React to build dynamic and responsive user interfaces.

React is a JavaScript library for building modern front-end applications. 1. It uses componentized and virtual DOM to optimize performance. 2. Components use JSX to define, state and attributes to manage data. 3. Hooks simplify life cycle management. 4. Use ContextAPI to manage global status. 5. Common errors require debugging status updates and life cycles. 6. Optimization techniques include Memoization, code splitting and virtual scrolling.

React's future will focus on the ultimate in component development, performance optimization and deep integration with other technology stacks. 1) React will further simplify the creation and management of components and promote the ultimate in component development. 2) Performance optimization will become the focus, especially in large applications. 3) React will be deeply integrated with technologies such as GraphQL and TypeScript to improve the development experience.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
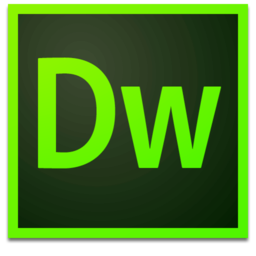
Dreamweaver Mac version
Visual web development tools
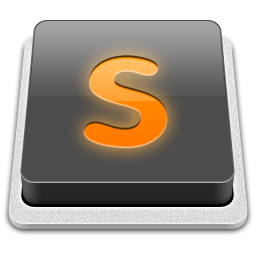
SublimeText3 Mac version
God-level code editing software (SublimeText3)

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
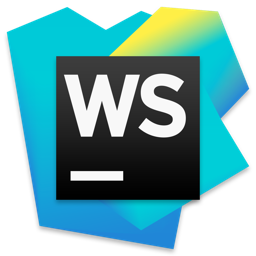
WebStorm Mac version
Useful JavaScript development tools