Related learning recommendations: javascript video tutorial
Preface
I have wanted to do this series since last year when I discovered that the replace method of strings has many magical uses, but I never had the time and didn’t think of a good name, so I put it on hold. When I was doing my homework last Friday, I saw a solution in the comments, which is to use Array.from to solve it in one line, and it is quite efficient. So I read through the documents and blogs, and after I figured it out, I thought it was time to start this series. Moreover, arrays are one of the most commonly used data structures in our development. As one of the methods of generating arrays, it makes sense to start with from. Let’s just make do with the name of the series.
- Basic syntax
Definition: The from() method is used to pass an object or iterable with the length property Object to return an array.
Syntax: Array.from(object, mapFunction, thisValue)
Description | |
object | Required, the object to be converted to an array.|
mapFunction | Optional, the function to be called for each element in the array.|
thisValue | Optional, the this object in the mapping function (mapFunction).
- Example usage
1. Convert class array to array
Array.from('hello') //["h", "e", "l", "l", "o"] Array.from(new Set(['name','age'])) //["name", "age"] Array.from({name:'lgc',age:25}) //[] let map=new Map() map.set('name','lgc') map.set('age',25) Array.from(map) //[["name", "lgc"],["age", 25]] function test(){ console.log(Array.from(arguments)) } test(1,2,3) //[1, 2, 3]复制代码This is our most commonly used function. When writing these examples, I was still wondering: why map can be converted into an array but object can only be converted into an empty array. When I looked at the novice tutorial and saw the above definition, I understood. Object has neither a length nor an iterable object. I used to think that object was also an iterable object. After all, you can use for-in. But in fact, the object of es6 is not an iterable object. I won’t go into details here. Interested students can check it out.
2. Array deep copy (one line of code)
function clone(arr){ return Array.isArray(arr) ? Array.from(arr, clone):arr } let arrayA=[[1,2],[3,4]] let arrayB=clone(arrayA) arrayA===arrayB //false arrayA[0]===arrayB[0] //false复制代码The second parameter mapFunction of Array.from is mainly used here. By default, mapFunction passes two parameters, array value and subscript.
3. Array deduplication
function unique(arr){ return Array.from(new Set(arr)) }复制代码This is also the most basic from and one of our most commonly used functions.
4. Other uses of from
Look at the definition of from again: the from() method is used to pass objects or objects with thelength attribute An iterable object to return an array. Just have length? Give it a try
Array.from({length:2},(val,index)=>index) //[0,1]复制代码Okay, but what’s the use of it? First, like the above code, you can easily generate an array within a certain range and with certain rules
Array.from({length:3},(val,index)=>index*10) //[0,10,20]复制代码Second, the initialization of the array. For example, you want to generate an object array of a specified length. What was the first reaction? fill?
let testArr=Array(3).fill({}) testArr[0]===testArr[1] //true复制代码Every object here is actually the same. If you modify one, the others will naturally change accordingly, but many times what we need is not like this.
let testArrb=Array.from({length:3},()=>({})) testArrb[0]===testArrb[1] //false复制代码These two methods can be used according to needs.
5. Advanced usage of from
The above content is actually layer by layer to better understand the following problem-solving ideas. LeetCode Question 867: Given a matrixA, return the transposed matrix of
A.
var transpose = function(A) { let x=A.length let y=A[0].length for(let i=0;i<x;i++){ for(let j=0;j<y;j++){ if(j-i>0){ [A[i][j],A[j][i]]=[A[j][i],A[i][j]] } } } return A };复制代码Execution code: passed, submission: failed. Damn it? Looking at the error message, I found that the situation in Example 2 where the "length and width" are not equal was ignored. Change your thinking and flip the internal and external cycles. Each time the outermost loop is executed, one column is treated as a row. Execute, pass. But this version looks too expensive, and the execution time is too slow. But after all, I have implemented it myself, so you can go to the comment area to find other ideas. The following is implemented by the master in the comment area, but I didn’t understand it the first time.
var transpose = function(A) { return Array.from({length:A[0].length},(_v,i)=>A.map(v=>v[i]))};复制代码{length:A[0].length}, uses the width of the given matrix as the length of the transposed matrix, in order to satisfy the condition of "an object with the
length attribute" . (_v,i)=>A.map(v=>v[i]), takes the columns of the given matrix as the rows of the transposed matrix. The core idea is the same as my second version, but the implementation methods and techniques are too much. And the execution time is also shorter, worship the great god.
If you want to know more about programming learning, please pay attention to thephp training column!
The above is the detailed content of What you need to know about JS arrays: Array.from. For more information, please follow other related articles on the PHP Chinese website!
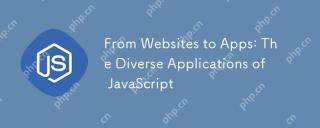
JavaScript is widely used in websites, mobile applications, desktop applications and server-side programming. 1) In website development, JavaScript operates DOM together with HTML and CSS to achieve dynamic effects and supports frameworks such as jQuery and React. 2) Through ReactNative and Ionic, JavaScript is used to develop cross-platform mobile applications. 3) The Electron framework enables JavaScript to build desktop applications. 4) Node.js allows JavaScript to run on the server side and supports high concurrent requests.
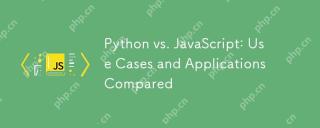
Python is more suitable for data science and automation, while JavaScript is more suitable for front-end and full-stack development. 1. Python performs well in data science and machine learning, using libraries such as NumPy and Pandas for data processing and modeling. 2. Python is concise and efficient in automation and scripting. 3. JavaScript is indispensable in front-end development and is used to build dynamic web pages and single-page applications. 4. JavaScript plays a role in back-end development through Node.js and supports full-stack development.
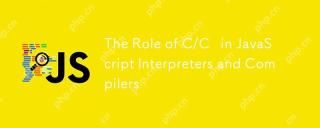
C and C play a vital role in the JavaScript engine, mainly used to implement interpreters and JIT compilers. 1) C is used to parse JavaScript source code and generate an abstract syntax tree. 2) C is responsible for generating and executing bytecode. 3) C implements the JIT compiler, optimizes and compiles hot-spot code at runtime, and significantly improves the execution efficiency of JavaScript.
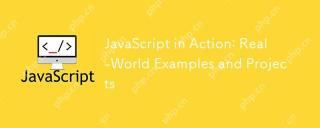
JavaScript's application in the real world includes front-end and back-end development. 1) Display front-end applications by building a TODO list application, involving DOM operations and event processing. 2) Build RESTfulAPI through Node.js and Express to demonstrate back-end applications.
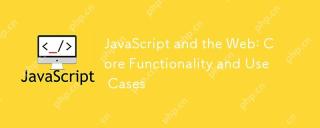
The main uses of JavaScript in web development include client interaction, form verification and asynchronous communication. 1) Dynamic content update and user interaction through DOM operations; 2) Client verification is carried out before the user submits data to improve the user experience; 3) Refreshless communication with the server is achieved through AJAX technology.
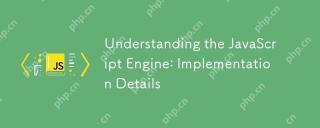
Understanding how JavaScript engine works internally is important to developers because it helps write more efficient code and understand performance bottlenecks and optimization strategies. 1) The engine's workflow includes three stages: parsing, compiling and execution; 2) During the execution process, the engine will perform dynamic optimization, such as inline cache and hidden classes; 3) Best practices include avoiding global variables, optimizing loops, using const and lets, and avoiding excessive use of closures.

Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
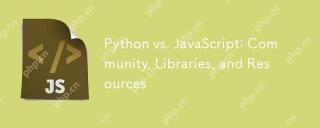
Python and JavaScript have their own advantages and disadvantages in terms of community, libraries and resources. 1) The Python community is friendly and suitable for beginners, but the front-end development resources are not as rich as JavaScript. 2) Python is powerful in data science and machine learning libraries, while JavaScript is better in front-end development libraries and frameworks. 3) Both have rich learning resources, but Python is suitable for starting with official documents, while JavaScript is better with MDNWebDocs. The choice should be based on project needs and personal interests.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
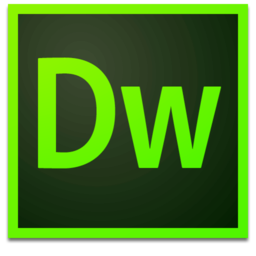
Dreamweaver Mac version
Visual web development tools
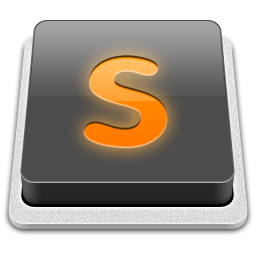
SublimeText3 Mac version
God-level code editing software (SublimeText3)

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
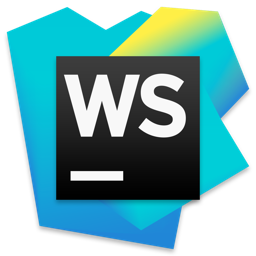
WebStorm Mac version
Useful JavaScript development tools