How to implement the mini program to push template messages?
The following are the development steps
Get the user’s openid
##Get the form_id or prepay_id
Get access_token
Send template message
DEMO download address
Important tipsThis method uses PHP's built-in curl module to send requests. During development, this method is used to access the WeChat server to obtain data, where url is the interface address, params is the carrying parameters, ispost is the request method, and https is the certificate verification
public static function curl($url, $params = false, $ispost = 0, $https = 0) { $httpInfo = array(); $ch = curl_init(); curl_setopt($ch, CURLOPT_HTTP_VERSION, CURL_HTTP_VERSION_1_1); curl_setopt( $ch, CURLOPT_HTTPHEADER, array( 'Content-Type: application/json; charset=utf-8' ) ); curl_setopt($ch, CURLOPT_USERAGENT, 'Mozilla/5.0 (Windows NT 10.0; WOW64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/41.0.2272.118 Safari/537.36'); curl_setopt($ch, CURLOPT_CONNECTTIMEOUT, 30); curl_setopt($ch, CURLOPT_TIMEOUT, 30); curl_setopt($ch, CURLOPT_RETURNTRANSFER, true); if ($https) { curl_setopt($ch, CURLOPT_SSL_VERIFYPEER, FALSE); // 对认证证书来源的检查 curl_setopt($ch, CURLOPT_SSL_VERIFYHOST, FALSE); // 从证书中检查SSL加密算法是否存在 } if ($ispost) { curl_setopt($ch, CURLOPT_POST, true); curl_setopt($ch, CURLOPT_POSTFIELDS, $params); curl_setopt($ch, CURLOPT_URL, $url); } else { if ($params) { if (is_array($params)) { $params = http_build_query($params); } curl_setopt($ch, CURLOPT_URL, $url . '?' . $params); } else { curl_setopt($ch, CURLOPT_URL, $url); } } $response = curl_exec($ch); if ($response === FALSE) { return false; } $httpCode = curl_getinfo($ch, CURLINFO_HTTP_CODE); $httpInfo = array_merge($httpInfo, curl_getinfo($ch)); curl_close($ch); return $response; }Get the user's openidWeChat applet code, it is recommended to save it globally in app.js to facilitate calling
wx.login({ success: function (res) { wx.request({ url: "www.xxx.com", //你的服务器接口地址 data: { code:res.code //通过wx.login获取code发送至服务器 }, header: { 'content-type': 'application/json' }, success: function (res) { that.globalData.OpenId=res.data.openid //存储openid } }) } })The server-side PHP code, I use the laravel framework, Can be reconstructed by yourself
public function getUserInfo(Request $request) { $code = $request->get("code"); $appid=""; //小程序appid $secret=""; //小程序secret $Url = 'https://api.weixin.qq.com/sns/jscode2session?appid=' . $appid . '&secret=' . $secre . '&js_code=' . $code . '&grant_type=authorization_code'; //微信官方给出的接口,利用小程序内获取的code置换openid $UserInfo=HttpUtils::curl($Url, $params = false, $ispost = 0, $https = 1); //上文给出的curl方法 echo $UserInfo; //输出结果,其中包含openid }Get form_id or prepay_idThis article will only give a brief introduction, leaving it to the next blog to explain WeChat payment1.form_id is submitted within the mini program The id generated during the form. When the user submits the form in the mini program and the form is declared to send template messages, and the developer needs to provide services to the user, the developer can be allowed to push limited messages to the user within 7 days. Number of template messages (one submission of the form can be issued once, and the number of entries issued by multiple submissions is independent and does not affect each other) 2.prepay_id is the prepayment generated when the mini program launches WeChat payment. id, when the user completes the payment behavior in the mini program, the developer is allowed to push a limited number of template messages to the user within 7 days (3 messages can be sent for one payment, and the number of messages for multiple payments is independent and mutually exclusive. Impact) Get access_tokenThis method provides parameters for obtaining access_token and subsequently sending template messages. I use the laravel framework and can reconstruct it by myself
public static function access_token(){ $appid=""; //小程序appid $secret=""; //小程序secret $Url="https://api.weixin.qq.com/cgi-bin/token?grant_type=client_credential&appid=". $appid."&secret=".$secret; //微信给出的获取access_token的接口 $access_token=Cache::get("access_token"); //查询缓存中是否已存在access_token if($access_token==""){ $access_token=json_decode(self::curl($Url))->{"access_token"}; //访问接口获取access_token Cache::put("access_token",$access_token,120); //设置缓存,过期时间2小时 } return $access_token; }Send template messages Send template message method
public static function SendMsg($data,$access_token){ $MsgUrl="https://api.weixin.qq.com/cgi-bin/message/wxopen/template/send?access_token=".$access_token; //微信官方接口,需要拼接access_token return json_decode(self::curl($MsgUrl,$params=json_encode($data),$ispost=1,$https=1)); //访问接口,返回参数 }Call example
public function test(Request $request){ $form_id=$request->get("form_id"); $openid=$request->get("openid"); $access_token=WxUtils::access_token(); $data=[ "touser"=>$openid, //接收用户的openid "template_id"=>"k03-Sk5c4eNlQKrS4VqI4cKjEil7JyvcouxtKBFkVcs", //模板id "page"=>"pages/index/index",//点击模板消息跳转至小程序的页面 "form_id"=>$form_id, //可为表单提交时form_id,也可是支付产生的prepay_id "data"=>[ "keyword1"=>[ "value"=> "五公司", //自定义参数 "color"=> '#173177'//自定义文字颜色 ], "keyword2"=>[ "value"=> "保洁服务",//自定义参数 "color"=> '#173177'//自定义文字颜色 ], "keyword3"=>[ "value"=> "2018年10月",//自定义参数 "color"=> '#173177'//自定义文字颜色 ], "keyword4"=>[ "value"=> "已发布",//自定义参数 "color"=> '#173177'//自定义文字颜色 ], "keyword5"=>[ "value"=> "请至小程序订单列表进行查看",//自定义参数 "color"=> '#173177'//自定义文字颜色 ], ] ]; $res=WxUtils::SendMsg($data,$access_token); //返回结果 }
Summary1. Getting openid is quite simple, it is your appid and secret Just don’t make a mistake2. Access_token is the same as above. Don’t make a mistake filling in the parameters. Fill them in strictly according to the official documents3. In the data of the template message, jump to the applet Fill in the route strictly according to the route written by your mini program. When jumping to pages/index/index, do not write.../index/inexRelated recommendations:
The above is the detailed content of How to implement mini program push template message. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
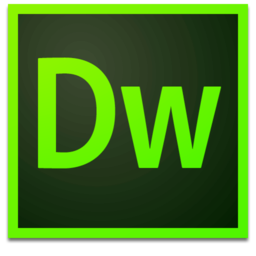
Dreamweaver Mac version
Visual web development tools
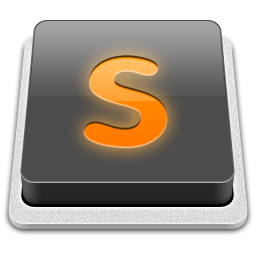
SublimeText3 Mac version
God-level code editing software (SublimeText3)

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
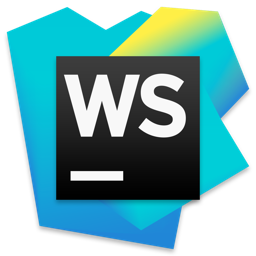
WebStorm Mac version
Useful JavaScript development tools