ASP.NET graphical verification code generation practice
Why use graphical verification code
In order to prevent attackers from writing programs to repeatedly submit forms and causing trouble for other users and website operations, it is generally necessary to use graphical verification before submitting the form. Code is verified to confirm that the submission operation is a human operation and not a machine batch operation.
Principle Analysis
When generating graphic verification codes, two technologies are mainly used:
1. Generate random Numbers or letters
2. Convert the generated random numbers or random letters into image format and add noise to the image and display it.
Generation method:
1. Front-end control code
<asp:TextBox ID="txtValidateNum" runat="server" Width="98px"></asp:TextBox> <asp:Image ID="Image1" runat="server" Height="22px" Width="58px" ImageUrl="~ValidateNum.aspx"/>请输入图片中验证码!
2. ValidateNum.aspx page processing steps
(1) Randomly generate a random string of length N. The value of N can be set by the developer. The string contains numbers, letters, etc.
(2) Create a randomly generated string into a picture and display it.
(3) Save the verification code
First create a CreateRandomNum(int n) method in the ValidateNum .aspx.cs file to randomly generate a random string of length n. In order to avoid generating repeated random numbers, the random number results will be recorded through variables. If the same value appears in the last random number, the method itself will be called to ensure that different random numbers are generated. The code is as follows:
//生成随机字符串 private string CreateRandomNum (int n) { string allChar = "0,1,2,3,4,5,6,7,8,9,A,B,C,D,E,F,G,H,I,J,K,L,M,N,O,P,Q,R,S,T,U,V,W,X,Y,Z"; string[] allCharArray = allChar.Split(',');//拆分成数组 string randomNum = ""; int temp = 1; Random rand = new Random(); for(int i = 0;i < n;i++) { if(temp != -1) { rand = new Random(i * temp *((int)DateTime.Now.Ticks)); } int t = rand.Next(35); if(temp == t) { return CreateRandomNum(n); } temp = t; randomNum += allCharArray[i]; } return randomNum; }
Then create the CreateImage(string validateNum) method based on the generated random string to further generate a graphic code. In order to further ensure security, add some interference colors to the graphic verification code, such as random background patterns, Word processing, etc. The code is as follows:
//生成图片 private void CreateImage(string validateNum) { if(validateNum == null || validateNum.Trim() == String.Empty) return; //生成Bitmap图像 System.Drawing.Bitmap image = new System.Drawing.Bitmap(validateNum.Length * 12 + 10,22); Graphics g = Graphics.FromImage(image); try { //生成随机生成器 Random random = new Random(); //清空图片背景色 g.Clear(Color.White); //画图片的背景噪音线 for(int i = 0; i < 25; i++) { int x1 = random.Next(image.Width); int x2 = random.Next(image.Width); int y1 = random.Next(image.Height); int y2 = random.Next(image.Height); g.DrawLine(new Pen(Color.Silver),x1,y1,x2,y2); } Font font = new System.Drawing.Font("Arial",12,(System.Drawing.FontStyle.Bold | System.Drawing.FontStyle.Italic)); System.Drawing.Drawing2D.LinearGradientBrush brush = new System.Drawing.Drawing2D.LinearGradientBrush(new Rectangle(0,0,image.Width,image.Height),Color.Blue,Color.DarkRed,1.2f,true); g.DrawString(validateNum,font,brush,2,2); //画图片的前景噪音点 for(int i = 0;i<100;i++) { int x = random.Next(image.Width); int y = random.Next(image.Height); image.SetPixel(x,y,Color.FromArgb(random.Next())); } //画图片的边框线 g.DrawRectangle(new Pen(Color.Silver),0,0,image.Width - 1,image.Height - 1); System.IO.MemoryStream ms = new System.IO.MemoryStream(); //将图像保存到指定的流 image.Save(ms,System.Drawing.Imaging.ImageFormat.Gif); Response.ClearContent(); Response.ContentType = "image/Gif"; Response.BinaryWrite(ms.ToArray()); } finally { g.Dispose(); image.Dispose(); } }
Finally, in the page loading event Page_Load, create and display the image of the verification code string, and save the verification string in the Session.
protected void Page_Load(object sender,EventArgs e) { if(!IsPostBack) { string validateNum = CreateRandomNum(4);//生成4位随机字符串 CreateImage(validateNum);//将随机字符串绘制成图片 Session["ValidateNum"] = validateNum; //将随机字符串保存在Session中 } }
At this point, a simple image verification code generation algorithm has been written. Of course, you can add richer security settings on this basis, such as Session expiration time, etc.
The above is the detailed content of ASP.NET graphical verification code generation practice. For more information, please follow other related articles on the PHP Chinese website!
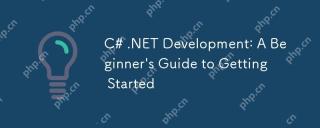
To start C#.NET development, you need to: 1. Understand the basic knowledge of C# and the core concepts of the .NET framework; 2. Master the basic concepts of variables, data types, control structures, functions and classes; 3. Learn advanced features of C#, such as LINQ and asynchronous programming; 4. Be familiar with debugging techniques and performance optimization methods for common errors. With these steps, you can gradually penetrate the world of C#.NET and write efficient applications.
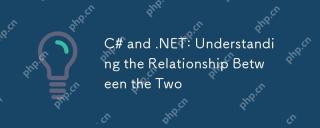
The relationship between C# and .NET is inseparable, but they are not the same thing. C# is a programming language, while .NET is a development platform. C# is used to write code, compile into .NET's intermediate language (IL), and executed by the .NET runtime (CLR).
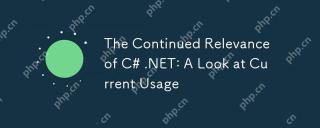
C#.NET is still important because it provides powerful tools and libraries that support multiple application development. 1) C# combines .NET framework to make development efficient and convenient. 2) C#'s type safety and garbage collection mechanism enhance its advantages. 3) .NET provides a cross-platform running environment and rich APIs, improving development flexibility.
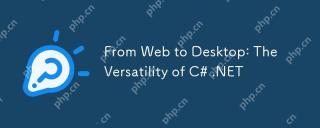
C#.NETisversatileforbothwebanddesktopdevelopment.1)Forweb,useASP.NETfordynamicapplications.2)Fordesktop,employWindowsFormsorWPFforrichinterfaces.3)UseXamarinforcross-platformdevelopment,enablingcodesharingacrossWindows,macOS,Linux,andmobiledevices.
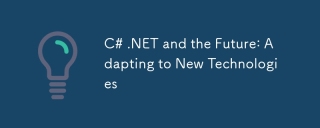
C# and .NET adapt to the needs of emerging technologies through continuous updates and optimizations. 1) C# 9.0 and .NET5 introduce record type and performance optimization. 2) .NETCore enhances cloud native and containerized support. 3) ASP.NETCore integrates with modern web technologies. 4) ML.NET supports machine learning and artificial intelligence. 5) Asynchronous programming and best practices improve performance.
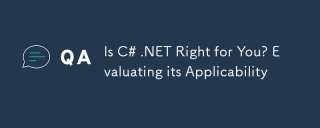
C#.NETissuitableforenterprise-levelapplicationswithintheMicrosoftecosystemduetoitsstrongtyping,richlibraries,androbustperformance.However,itmaynotbeidealforcross-platformdevelopmentorwhenrawspeediscritical,wherelanguageslikeRustorGomightbepreferable.
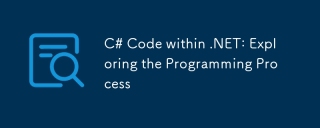
The programming process of C# in .NET includes the following steps: 1) writing C# code, 2) compiling into an intermediate language (IL), and 3) executing by the .NET runtime (CLR). The advantages of C# in .NET are its modern syntax, powerful type system and tight integration with the .NET framework, suitable for various development scenarios from desktop applications to web services.
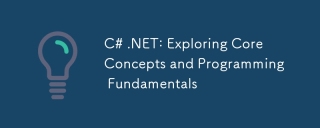
C# is a modern, object-oriented programming language developed by Microsoft and as part of the .NET framework. 1.C# supports object-oriented programming (OOP), including encapsulation, inheritance and polymorphism. 2. Asynchronous programming in C# is implemented through async and await keywords to improve application responsiveness. 3. Use LINQ to process data collections concisely. 4. Common errors include null reference exceptions and index out-of-range exceptions. Debugging skills include using a debugger and exception handling. 5. Performance optimization includes using StringBuilder and avoiding unnecessary packing and unboxing.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor
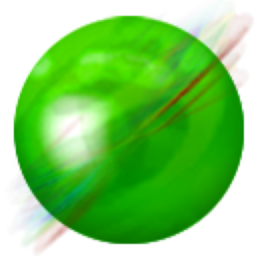
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
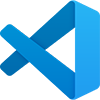
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.