laraveli adds one or more user tables, taking admin as an example.
Some file contents may need to be modified according to the actual situation
Recommended: laravel tutorial
Create an Admin model
php artisan make:model Admin -m
Write admins Table fields
Schema::create('admins', function (Blueprint $table) { $table->increments('id'); $table->string('name')->unique(); $table->string('password'); $table->rememberToken(); $table->timestamps(); });
Edit admin model
<?php namespace App; use Illuminate\Notifications\Notifiable; use Illuminate\Foundation\Auth\User as Authenticatable; /** * @property int $id * @property \Carbon\Carbon $created_at * @property \Carbon\Carbon $updated_at */ class Admin extends Authenticatable { use Notifiable; protected $fillable = [ 'name', 'password','remember_token' ]; protected $hidden = [ 'password','remember_token' ]; }
Modify the auth.php configuration file
'guards' => [ ... 'admin' => [ 'driver' => 'session', 'provider' => 'admins' ] ], 'providers' => [ ... 'admins' => [ 'driver' => 'eloquent', 'model' => App\Admin::class, ] ],
Create the directory Admin/Auth under app/Http/Controllers
Create the file HomeController.php in the Admin directory (this file is used to test the jump page after successful login)
<?php namespace App\Http\Controllers\Admin; use App\Http\Controllers\Controller; use Illuminate\Http\Request; class HomeController extends Controller { /** * HomeController constructor. */ public function __construct() { $this->middleware('auth:admin'); } /** * Show the application dashboard. * * @return \Illuminate\Http\Response */ public function index() { return view('admin.home'); } }
Use the command to generate a Request
php artisan make:request AdminLoginRequest
At this time in app/Http/Request This file is generated in the directory, and then edit this file
<?php namespace App\Http\Requests; use Illuminate\Foundation\Http\FormRequest; class AdminLoginRequest extends FormRequest { /** * 确定用户是否有权发出此请求. * * @return bool */ public function authorize() { return true; } /** * 获取适用于请求的验证规则. * * @return array */ public function rules() { return [ 'name' => 'required', 'password' => ['required', 'min:6'] //密码必须,最小长度为6 ]; } }
Create the file LoginController.php
<?php namespace App\Http\Controllers\Admin\Auth; use App\Http\Controllers\Controller; use App\Http\Requests\AdminLoginRequest; use Illuminate\Support\Facades\Auth; class LoginController extends Controller { public function showLoginForm() { return view('admin.auth.login'); } public function postLogin(AdminLoginRequest $loginRequest) { $data = $loginRequest->only('name', 'password'); $result = Auth::guard('admin')->attempt($data, true); if ($result) { return redirect(route('admin.home')); } else { return redirect()->back() ->with('name', $loginRequest->get('name')) ->withErrors(['name' => '用户名或密码错误']); } } public function postLogout() { Auth::guard('admin')->logout(); return redirect(route('admin.login.show')); } }
in the Admin/Auth directory to add routing. Open app/providers/RouteServiceProvider.php
Add a method after the method mapWebRoutes()
protected function mapAdminWebRoutes() { Route::middleware('web') ->prefix('admin') ->namespace($this->namespace) ->group(base_path('routes/admin.php')); }
Call the above added method in the map() method
public function map() { $this->mapApiRoutes(); $this->mapAdminWebRoutes();//调用新增的方法 $this->mapWebRoutes(); }
In Add a routing file admin.php to the routes directory
<?php Route::get('login','Admin\Auth\LoginController@showLoginForm') ->middleware('guest:admin') ->name('admin.login.show'); Route::get('/','Admin\HomeController@index') ->name('admin.home'); Route::post('login','Admin\Auth\LoginController@postLogin') ->middleware('guest:admin') ->name('admin.login.post'); Route::post('logout','Admin\Auth\LoginController@postLogout') ->middleware('auth:admin') ->name('admin.logout');
Copy home.blade.php to resources/views/admin
Copy layouts/app.blade.php to layouts/admin .blade.php, modify the corresponding place
<ul class="nav navbar-nav navbar-right"> <!-- Authentication Links --> @guest('admin') <li><a href="{{ route('admin.login.show') }}">admin Login</a></li> @else <li class="dropdown"> <a href="#" class="dropdown-toggle" data-toggle="dropdown" role="button" aria-expanded="false" aria-haspopup="true"> {{ Auth::guard('admin')->user()->name }} <span class="caret"></span> </a> <ul class="dropdown-menu"> <li> <a href="{{ route('admin.logout') }}" onclick="event.preventDefault(); document.getElementById('logout-form').submit();"> Logout </a> <form id="logout-form" action="{{ route('admin.logout') }}" method="POST" style="display: none;"> {{ csrf_field() }} </form> </li> </ul> </li> @endguest </ul>
Copy login.blade.php to the admin/Auth directory
@extends('layouts.admin') @section('content') <div class="container"> <div class="row"> <div class="col-md-8 col-md-offset-2"> <div class="panel panel-default"> <div class="panel-heading">Admin Login</div> <div class="panel-body"> <form class="form-horizontal" method="POST" action="{{ route('admin.login.post') }}"> {{ csrf_field() }} <div class="form-group{{ $errors->has('name') ? ' has-error' : '' }}"> <label for="name" class="col-md-4 control-label">E-Mail Address</label> <div class="col-md-6"> <input id="name" type="text" class="form-control" name="name" value="{{ old('name') }}" required autofocus> @if ($errors->has('name')) <span class="help-block"> <strong>{{ $errors->first('name') }}</strong> </span> @endif </div> </div> <div class="form-group{{ $errors->has('password') ? ' has-error' : '' }}"> <label for="password" class="col-md-4 control-label">Password</label> <div class="col-md-6"> <input id="password" type="password" class="form-control" name="password" required> @if ($errors->has('password')) <span class="help-block"> <strong>{{ $errors->first('password') }}</strong> </span> @endif </div> </div> <div class="form-group"> <div class="col-md-8 col-md-offset-4"> <button type="submit" class="btn btn-primary"> Login </button> </div> </div> </form> </div> </div> </div> </div> </div> @endsection
Data filling
php artisan make:seed AdminsTableSeeder
Edit AdminsTableSeeder.php
public function run() { \App\Admin::insert([ 'name'=>'yzha5', 'password'=> bcrypt('123456') ]); } DatabaseSeeder.php $this->call(AdminsTableSeeder::class);
Upload the file to the server, log in to the server, and execute the fill command
php artisan migrate php artisan db:seed
At this time, opening http://xxx/admin directly will not jump to http://xxx/admin/ login, so some exceptions need to be handled. Open app/Exceptions/Handle.php
Rewrite the unauthenticated() method.
use Illuminate\Support\Facades\Route; protected function unauthenticated($request, AuthenticationException $exception) { return starts_with(Route::currentRouteName(), 'admin') ? redirect(route('admin.login.show')) : parent::unauthenticated($request, $exception); }
Improve the above code. When the admin logs in and accesses the URI /admin/login again, it will automatically jump to the URI /home. This is because of the guest middleware. By default, it jumps to /home, which is the file RedirectIfAuthenticated.php in the middleware directory.
The solution is:Create a single file named: RedirectIfAdminAuthenticated
php artisan make:middleware RedirectIfAdminAuthenticated
Edit this file:
<?php namespace App\Http\Middleware; use Closure; use Illuminate\Support\Facades\Auth; class RedirectIfAdminAuthenticated { /** * Handle an incoming request. * * @param $request * @param Closure $next * @param null $guard * @return \Illuminate\Http\RedirectResponse|\Illuminate\Routing\Redirector|mixed */ public function handle($request, Closure $next, $guard = null) { if (Auth::guard($guard)->check()) { return redirect('/admin'); } return $next($request); } } 在Kernel.php中添加一行 protected $routeMiddleware = [ ... 'admin.guest' => \App\Http\Middleware\RedirectIfAdminAuthenticated::class, ... ]; 更改admin路由,将guest:admin改为admin.guest:admin Route::get('login','Admin\Auth\LoginController@showLoginForm') ->middleware('admin.guest:admin') ->name('admin.login.show'); Route::post('login','Admin\Auth\LoginController@postLogin') ->middleware('admin.guest:admin') ->name('admin.login.post');
The above is the detailed content of How to implement multi-user system login in laravel. For more information, please follow other related articles on the PHP Chinese website!

Collaborative document editing is an effective tool for distributed teams to optimize their workflows. It improves communication and project progress through real-time collaboration and feedback loops, and common tools include Google Docs, Microsoft Teams, and Notion. Pay attention to challenges such as version control and learning curve when using it.

ThepreviousversionofLaravelissupportedwithbugfixesforsixmonthsandsecurityfixesforoneyearafteranewmajorversion'srelease.Understandingthissupporttimelineiscrucialforplanningupgrades,ensuringprojectstability,andleveragingnewfeaturesandsecurityenhancemen

Laravelcanbeeffectivelyusedforbothfrontendandbackenddevelopment.1)Backend:UtilizeLaravel'sEloquentORMforsimplifieddatabaseinteractions.2)Frontend:LeverageBladetemplatesforcleanHTMLandintegrateVue.jsfordynamicSPAs,ensuringseamlessfrontend-backendinteg

Laravelcanbeusedforfullstackdevelopment.1)BackendmasterywithLaravel'sexpressivesyntaxandfeatureslikeEloquentORMfordatabasemanagement.2)FrontendintegrationusingBladefordynamicHTMLtemplates.3)EnhancingfrontendwithLaravelMixforassetcompilation.4)Fullsta

Answer: The best tools for upgrading Laravel include Laravel's UpgradeGuide, LaravelShift, Rector, Composer, and LaravelPint. 1. Use Laravel's UpgradeGuide as the upgrade roadmap. 2. Use LaravelShift to automate most of the upgrade work, but it requires manual review. 3. Automatically refactor the code through Rector, and you need to understand and possibly customize its rules. 4. Use Composer to manage dependencies and pay attention to possible dependency conflicts. 5. Run LaravelPint to maintain code style consistency, but it does not solve the functional problems.

ToenhanceengagementandcohesionamongdistributedteamsbeyondZoom,implementthesestrategies:1)Organizevirtualcoffeebreaksforinformalchats,2)UseasynchronoustoolslikeSlackfornon-workdiscussions,3)Introducegamificationwithteamgamesorchallenges,and4)Encourage

Laravel10introducesseveralbreakingchanges:1)ItrequiresPHP8.1orhigher,2)TheRouteServiceProvidernowusesabootmethodforloadingroutes,3)ThewithTimestamps()methodonEloquentrelationshipsisdeprecated,and4)TheRequestclassnowpreferstherules()methodforvalidatio

Tomaintainfocusandmotivationinremotework,createastructuredenvironment,managedigitaldistractions,fostermotivationthroughsocialinteractionsandgoalsetting,maintainwork-lifebalance,anduseappropriatetechnology.1)Setupadedicatedworkspaceandsticktoaroutine.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
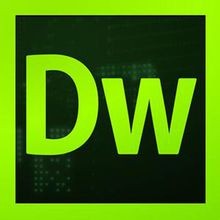
Dreamweaver CS6
Visual web development tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
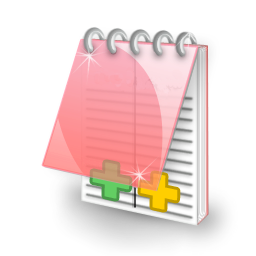
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
