Brief Tutorial
wallop is a mobile-first pure JavaScript slideshow plugin. This slideshow plug-in is only 4k in size. Its principle is just to add and remove appropriate classes for HTML elements to show and hide them. As for these class styles, you can completely customize them. Features include:
Mobile first
Animation and transition effects are generated using CSS
Lightweight, only 4k size
Highly flexible and scalable
Customizable events and API
No external dependencies
How to use
To use this slideshow plug-in, you first need to introduce the wallop.css and Wallop.min.js files.
<link rel="stylesheet" href="path/to/wallop.css"> <script src="path/to/Wallop.min.js"></script>
HTML structure
The basic HTML structure of this slide is as follows. You can add .Wallop-item--current class to the first slide.
<div class="Wallop"> <div class="Wallop-list"> <div class="Wallop-item">…</div> <div class="Wallop-item">…</div> <div class="Wallop-item">…</div> <div class="Wallop-item">…</div> <div class="Wallop-item">…</div> </div> <button class="Wallop-buttonPrevious">Previous</button> <button class="Wallop-buttonNext">Next</button> </div>
Initialization plug-in
<script> var wallopEl = document.querySelector('.Wallop'); var slider = new Wallop(wallopEl); </script>
Add animation effects
This slideshow plug-in does not have animation transition effects by default. If you need to add animation effects, you need to introduce the corresponding wallop-animation.css file and add Wallop--xxxx class to the element. For example, to add a fade effect:
<head> <link rel="stylesheet" href="path/to/wallop.css"> <link rel="stylesheet" href="path/to/wallop-fade.css"> </head> <div class="Wallop Wallop--fade"> ... </div>
Available animation types
The transition animation types available for this slide are:
Wallop--slide
Wallop--fade
Wallop--scale
Wallop--rotate
Wallop--fold
Wallop--vertical-slide
The above are some built-in transition animation types, and you can also customize your own transition animation.
Configuration parameters
Here are some available configuration parameters:
buttonPreviousClass: 'Wallop-buttonPrevious'
buttonNextClass: 'Wallop-buttonNext'
itemClass: 'Wallop-item'
currentItemClass: 'Wallop-item--current'
showPreviousClass: 'Wallop-item--showPrevious'
showNextClass: 'Wallop-item--showNext'
hidePreviousClass: 'Wallop-item--hidePrevious'
hideNextClass: 'Wallop-item--hideNext'
carousel: true
Method
The Wallop slideshow plugin provides some basic methods for button control:
goTo
Allows jumping to the slide at the specified index.
var slider = document.querySelector('.Wallop'); var Wallop = new Wallop(slider); // 跳转到第二个slide(index从0开始) Wallop.goTo(1);
next
Jump to the next slide.
var slider = document.querySelector('.Wallop'); var Wallop = new Wallop(slider); // 跳转到下一个slide Wallop.next();
previous
Jump to the previous slide.
var slider = document.querySelector('.Wallop'); var Wallop = new Wallop(slider); // 跳转到前一个slide Wallop.previous();
Event
Wallop emits an event every time the slide changes, and it returns a detail object that contains the index of the current slide and the Wallop object.
var slider = document.querySelector('.Wallop'); var Wallop = new Wallop(slider); Wallop.on('change', function(event) { // event.detail.wallopEl // => <div class="Wallop">…</div> // event.detail.currentItemIndex // => number });
The above is the pure JavaScript mobile-first slideshow effect creation process shared with you. I hope it can help you create more beautiful slideshow effects.
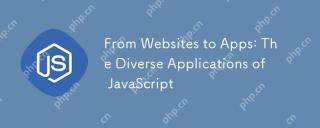
JavaScript is widely used in websites, mobile applications, desktop applications and server-side programming. 1) In website development, JavaScript operates DOM together with HTML and CSS to achieve dynamic effects and supports frameworks such as jQuery and React. 2) Through ReactNative and Ionic, JavaScript is used to develop cross-platform mobile applications. 3) The Electron framework enables JavaScript to build desktop applications. 4) Node.js allows JavaScript to run on the server side and supports high concurrent requests.
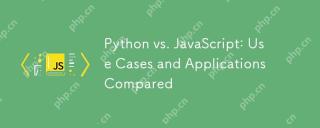
Python is more suitable for data science and automation, while JavaScript is more suitable for front-end and full-stack development. 1. Python performs well in data science and machine learning, using libraries such as NumPy and Pandas for data processing and modeling. 2. Python is concise and efficient in automation and scripting. 3. JavaScript is indispensable in front-end development and is used to build dynamic web pages and single-page applications. 4. JavaScript plays a role in back-end development through Node.js and supports full-stack development.
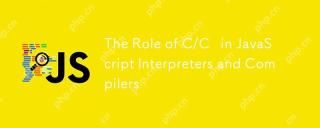
C and C play a vital role in the JavaScript engine, mainly used to implement interpreters and JIT compilers. 1) C is used to parse JavaScript source code and generate an abstract syntax tree. 2) C is responsible for generating and executing bytecode. 3) C implements the JIT compiler, optimizes and compiles hot-spot code at runtime, and significantly improves the execution efficiency of JavaScript.
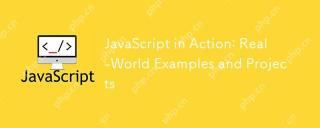
JavaScript's application in the real world includes front-end and back-end development. 1) Display front-end applications by building a TODO list application, involving DOM operations and event processing. 2) Build RESTfulAPI through Node.js and Express to demonstrate back-end applications.
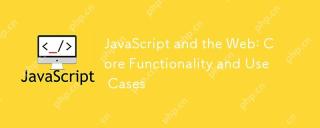
The main uses of JavaScript in web development include client interaction, form verification and asynchronous communication. 1) Dynamic content update and user interaction through DOM operations; 2) Client verification is carried out before the user submits data to improve the user experience; 3) Refreshless communication with the server is achieved through AJAX technology.
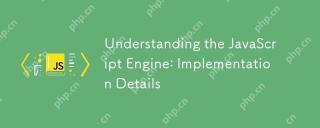
Understanding how JavaScript engine works internally is important to developers because it helps write more efficient code and understand performance bottlenecks and optimization strategies. 1) The engine's workflow includes three stages: parsing, compiling and execution; 2) During the execution process, the engine will perform dynamic optimization, such as inline cache and hidden classes; 3) Best practices include avoiding global variables, optimizing loops, using const and lets, and avoiding excessive use of closures.

Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
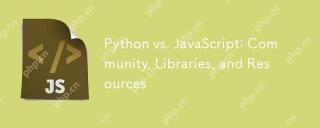
Python and JavaScript have their own advantages and disadvantages in terms of community, libraries and resources. 1) The Python community is friendly and suitable for beginners, but the front-end development resources are not as rich as JavaScript. 2) Python is powerful in data science and machine learning libraries, while JavaScript is better in front-end development libraries and frameworks. 3) Both have rich learning resources, but Python is suitable for starting with official documents, while JavaScript is better with MDNWebDocs. The choice should be based on project needs and personal interests.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
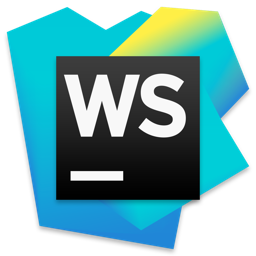
WebStorm Mac version
Useful JavaScript development tools

Atom editor mac version download
The most popular open source editor
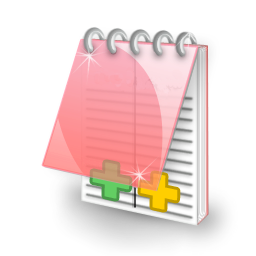
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software