Python factorial sum method
Title description:
Get the integer input by the user n, output the value of 1! 2! … n!.
If the input value is 0, negative, non-numeric or non-integer, a prompt message will be output: Incorrect input, please enter a positive integer.
(Recommended learning: Python video tutorial)
Method one:
#factTest1 def main(): a = input() sum = 0 if a.isdigit(): n = eval(a) if n > 0: fact = 1 for i in range(1, n+1): fact *= i sum += fact print(sum) else: print("输入有误,请输入正整数") else: print("输入有误,请输入正整数") main()
Method two: Recursive thinking
#factTest2 import sys sys.setrecursionlimit(5000) def getSum(i): sum = 0 if i==0: return 0 else: for x in range(1,i+1): sum += fact(x) return sum def fact(m): if m==0: return 1 else: return m*fact(m-1) def main(): n = input() if n.isdigit(): a = eval(n) if a>0: result = getSum(a) print(result) else: print("输入有误,请输入正整数") else: print("输入有误,请输入正整数") main()
Summary of the problem:
When using the recursive method to find the factorial of 1024, an exception occurred: RecursionError: maximum recursion depth exceeded in comparison, exceeded The maximum depth of recursion. Some netizens mentioned that the default maximum recursion depth in Python is 1000, but in actual testing, my computer experienced an exception when it reached 997. I don’t know what determines this. Therefore, in order to be able to calculate the factorial of 1024, a larger value needs to be given to the maximum recursion depth. The following methods can be used here:
import sys sys.setrecursionlimit(5000) #修改为5000
In addition, you can also view the maximum recursion depth:
import sys sys.getrecursionlimit() # output:1000
The above is the detailed content of Python factorial sum method. For more information, please follow other related articles on the PHP Chinese website!
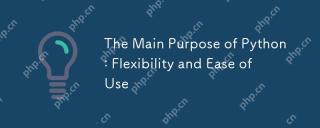
Python's flexibility is reflected in multi-paradigm support and dynamic type systems, while ease of use comes from a simple syntax and rich standard library. 1. Flexibility: Supports object-oriented, functional and procedural programming, and dynamic type systems improve development efficiency. 2. Ease of use: The grammar is close to natural language, the standard library covers a wide range of functions, and simplifies the development process.
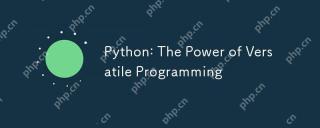
Python is highly favored for its simplicity and power, suitable for all needs from beginners to advanced developers. Its versatility is reflected in: 1) Easy to learn and use, simple syntax; 2) Rich libraries and frameworks, such as NumPy, Pandas, etc.; 3) Cross-platform support, which can be run on a variety of operating systems; 4) Suitable for scripting and automation tasks to improve work efficiency.
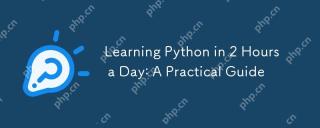
Yes, learn Python in two hours a day. 1. Develop a reasonable study plan, 2. Select the right learning resources, 3. Consolidate the knowledge learned through practice. These steps can help you master Python in a short time.
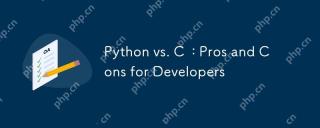
Python is suitable for rapid development and data processing, while C is suitable for high performance and underlying control. 1) Python is easy to use, with concise syntax, and is suitable for data science and web development. 2) C has high performance and accurate control, and is often used in gaming and system programming.
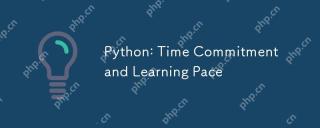
The time required to learn Python varies from person to person, mainly influenced by previous programming experience, learning motivation, learning resources and methods, and learning rhythm. Set realistic learning goals and learn best through practical projects.
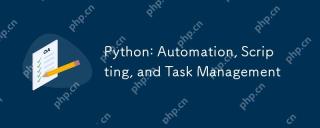
Python excels in automation, scripting, and task management. 1) Automation: File backup is realized through standard libraries such as os and shutil. 2) Script writing: Use the psutil library to monitor system resources. 3) Task management: Use the schedule library to schedule tasks. Python's ease of use and rich library support makes it the preferred tool in these areas.
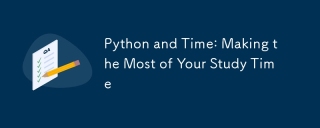
To maximize the efficiency of learning Python in a limited time, you can use Python's datetime, time, and schedule modules. 1. The datetime module is used to record and plan learning time. 2. The time module helps to set study and rest time. 3. The schedule module automatically arranges weekly learning tasks.
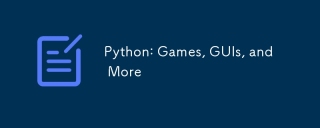
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
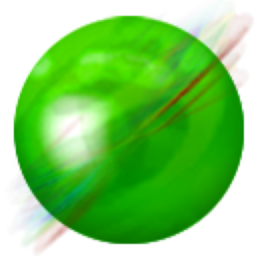
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

SublimeText3 English version
Recommended: Win version, supports code prompts!
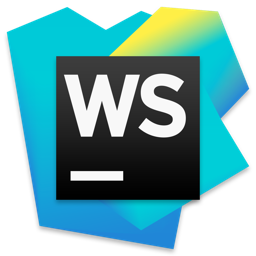
WebStorm Mac version
Useful JavaScript development tools

SublimeText3 Linux new version
SublimeText3 Linux latest version