Necessary practical skills for Laravel Eloquent
1. Increment and decrement
To replace the following implementation:
$article = Article::find($article_id); $article->read_count++; $article->save();
You can do this:
$article = Article::find($article_id); $article->increment('read_count');
The following methods can also be implemented:
Article::find($article_id)->increment('read_count'); Article::find($article_id)->increment('read_count', 10); // +10 Product::find($produce_id)->decrement('stock'); // -1
2. Execute the Use, for example "Please execute method X first, if method X fails, execute method Y".
Example 1 -- findOrFail():
To replace the implementation of the following code:
$user = User::find($id); if (!$user) { abort (404); }
You can write like this:
$user = User::findOrFail($id);
Example 2 -- firstOrCreate():
To replace the implementation of the following code:
$user = User::where('email', $email)->first(); if (!$user) { User::create([ 'email' => $email ]); }
Just write it like this:
$user = User::firstOrCreate(['email' => $email]);
3. The model's boot() method
In an Eloquent model, there is a magical place called boot(), where you can override the default behavior:
class User extends Model { public static function boot() { parent::boot(); static::updating(function($model) { // 写点日志啥的 // 覆盖一些属性,类似这样 $model->something = transform($something); }); } }
Set the values of certain fields when creating the model object, probably the most One of the popular examples. Let’s take a look at what to do if you want to generate a UUID field when creating a model object.
public static function boot() { parent::boot(); self::creating(function ($model) { $model->uuid = (string)Uuid::generate(); }); }
4. Association with conditions and sorting
General way to define association:
public function users() { return $this->hasMany('App\User'); }
Do you know? You can also add where or orderBy on the basis of the above,
For example, if you want to associate certain types of users and use the email field to sort at the same time, you can do this:
public function approvedUsers() { return $this->hasMany('App\User')->where('approved', 1)->orderBy('email'); }
5. Model features: time, append, etc.
Some parameters of the Eloquent model are in the form of class attributes. The most commonly used ones are:
class User extends Model { protected $table = 'users'; protected $fillable = ['email', 'password']; // 可以被批量赋值字段,如 User::create() 新增时,可使用字段 protected $dates = ['created_at', 'deleted_at']; // 需要被Carbon维护的字段名 protected $appends = ['field1', 'field2']; // json返回时,附加的字段 }
Not only these, but also:
protected $primaryKey = 'uuid'; // 更换主键 public $incrementing = false; // 设置 不自增长 protected $perPage = 25; // 定义分页每页显示数量(默认15) const CREATED_AT = 'created_at'; const UPDATED_AT = 'updated_at'; //重写 时间字段名 public $timestamps = false; // 设置不需要维护时间字段
There are more. I only listed some interesting features. Please refer to the document abstract Model class for details.
6. Query multiple records by ID
Everyone knows the find() method, right?
$user = User::find(1);
I am very surprised that few people know that this method can accept arrays of multiple IDs as parameters:
$users = User::find([1,2,3]);
7. WhereX
There is an elegant way to This kind of code:
$users = User::where('approved', 1)->get();
Convert to this kind of code:
$users = User::whereApproved(1)->get();
Yes, you read it right, use the field name as a suffix and add it to where after, and it will run through the magic method.
In addition, there are some predefined methods related to time in Eloquent:
User::whereDate('created_at', date('Y-m-d')); User::whereDay('created_at', date('d')); User::whereMonth('created_at', date('m')); User::whereYear('created_at', date('Y'));
8. Sorting by relationship
A more complicated "technique". Do you want to sort forum topics by latest posts? It’s a common requirement to have the most recently updated topics in a forum at the top, right?
First, define a separate relationship for the latest post of the topic:
public function latestPost() { return $this->hasOne(\App\Post::class)->latest(); }
Then, in the controller, we can implement this "magic":
$users = Topic::with('latestPost')->get()->sortByDesc('latestPost.created_at');
9. Eloquent::when() -- No more using if-else
Many people like to use "if-else" to write query conditions, like this:
if (request('filter_by') == 'likes') { $query->where('likes', '>', request('likes_amount', 0)); } if (request('filter_by') == 'date') { $query->orderBy('created_at', request('ordering_rule', 'desc')); }
There is a better way Method--use when()
$query = Author::query(); $query->when(request('filter_by') == 'likes', function ($q) { return $q->where('likes', '>', request('likes_amount', 0)); }); $query->when(request('filter_by') == 'date', function ($q) { return $q->orderBy('created_at', request('ordering_rule', 'desc')); });
It may not look very elegant, but its powerful function is to pass parameters:
$query = User::query(); $query->when(request('role', false), function ($q, $role) { return $q->where('role_id', $role); }); $authors = $query->get();
10. BelongsTo default model
If There is a Post model attached to the Author model, and the following code can be written in the Blade template:
{{ $post->author->name }}
But what if the author is deleted, or is not set for some reason? You will get an error message like "non-existent object property".
Well, you can avoid it like this:
{{ $post->author->name ?? '' }}
But you can do this effect at the Eloquent relational model level:
public function author() { return $this->belongsTo('App\Author')->withDefault(); }
In this example, if the post has no author Otherwise, the author() relationship method will return an empty App\Author model object.
In addition, we can also assign a default attribute value to the default model.
public function author() { return $this->belongsTo('App\Author')->withDefault([ 'name' => 'Guest Author' ]); }
11. Sorting by assignment function
Imagine you have this code:
function getFullNameAttribute() { return $this->attributes['first_name'] . ' ' . $this->attributes['last_name']; }
Now, you want to sort by "full_name"? Found that there is no The effect:
$clients = Client::orderBy('full_name')->get(); //没有效果
The solution is very simple. We need to sort the results after getting them.
$clients = Client::get()->sortBy('full_name'); // 成功!
Note that the method names are different--it is not orderBy, but sortBy
12. Default sorting under global scope
What if you want User::all() to always sort by the name field? You can assign it a global scope. Let's go back to the boot() method we mentioned above:
protected static function boot() { parent::boot(); // 按照 name 正序排序 static::addGlobalScope('order', function (Builder $builder) { $builder->orderBy('name', 'asc'); }); }
Extended reading Query scope.
13. Native query method
Sometimes, we need to add native queries to Eloquent statements. Fortunately, there is a way.
// whereRaw $orders = DB::table('orders') ->whereRaw('price > IF(state = "TX", ?, 100)', [200]) ->get(); // havingRaw Product::groupBy('category_id')->havingRaw('COUNT(*) > 1')->get(); // orderByRaw User::where('created_at', '>', '2016-01-01') ->orderByRaw('(updated_at - created_at) desc') ->get();
14. Copy: Copy a row
It’s very simple. The explanation is not very in-depth, but here is the best way to copy a database entity (a piece of data):
$task = Tasks::find(1); $newTask = $task->replicate(); $newTask->save();
15. Chunk() method of large chunks of data
is not completely related to Eloquent, it is more More about Collection (collection), but it is still very useful for processing large data collections. You can use chunk() to split this data into small data chunks
Before modification:
$users = User::all(); foreach ($users as $user) { // ...
You can do this:
User::chunk(100, function ($users) { foreach ($users as $user) { // ... } });
16. Add extra when creating the model Operation
We all know this Artisan command:
php artisan make:model Company
But you know that there are three very useful identifiers that can be used to generate model related files
php artisan make:model Company -mcr
-m Create migration files
-c Create controller file
-r Add resource operation method to controller
17. Specify updated_at
when calling the save method你知道 ->save() 方法可以接受参数吗? 我们可以通过传入参数阻止它的默认行为:更新 updated_at 的值为当前时间戳。
$product = Product::find($id); $product->updated_at = '2019-01-01 10:00:00'; $product->save(['timestamps' => false]);
这样,我们成功在 save 时指定了 updated_at 的值。
18. update() 的结果是什么?
你是否想知道这段代码实际上返回什么?
$result = $products->whereNull('category_id')->update(['category_id' => 2]);
我是说,更新操作是在数据库中执行的,但 $result 会包含什么?
答案是受影响的行。 因此如果你想检查多少行受影响, 你不需要额外调用其他任何内容 -- update() 方法会给你返回此数字。
19. 把括号转换成 Eloquent 查询
如果你有个 and 和 or 混合的 SQL 查询,像这样子的:
... WHERE (gender = 'Male' and age >= 18) or (gender = 'Female' and age >= 65)
怎么用 Eloquent 来翻译它呢? 下面是一种错误的方式:
$q->where('gender', 'Male'); $q->orWhere('age', '>=', 18); $q->where('gender', 'Female'); $q->orWhere('age', '>=', 65);
顺序就没对。正确的打开方式稍微复杂点,使用闭包作为子查询:
$q->where(function ($query) { $query->where('gender', 'Male') ->where('age', '>=', 18); })->orWhere(function($query) { $query->where('gender', 'Female') ->where('age', '>=', 65); })
20. 复数参数的 orWhere
终于,你可以传递阵列参数给 orWhere()。平常的方式:
$q->where('a', 1); $q->orWhere('b', 2); $q->orWhere('c', 3);
你可以这样做:
$q->where('a', 1); $q->orWhere(['b' => 2, 'c' => 3]);
更多laravel框架相关技术文章,请访问laravel教程栏目!
The above is the detailed content of Essential practical tips for Laravel Eloquent. For more information, please follow other related articles on the PHP Chinese website!

Laravel is suitable for building web applications quickly, while Python is suitable for a wider range of application scenarios. 1.Laravel provides EloquentORM, Blade template engine and Artisan tools to simplify web development. 2. Python is known for its dynamic types, rich standard library and third-party ecosystem, and is suitable for Web development, data science and other fields.

Laravel and Python each have their own advantages: Laravel is suitable for quickly building feature-rich web applications, and Python performs well in the fields of data science and general programming. 1.Laravel provides EloquentORM and Blade template engines, suitable for building modern web applications. 2. Python has a rich standard library and third-party library, and Django and Flask frameworks meet different development needs.

Laravel is worth choosing because it can make the code structure clear and the development process more artistic. 1) Laravel is based on PHP, follows the MVC architecture, and simplifies web development. 2) Its core functions such as EloquentORM, Artisan tools and Blade templates enhance the elegance and robustness of development. 3) Through routing, controllers, models and views, developers can efficiently build applications. 4) Advanced functions such as queue and event monitoring further improve application performance.

Laravel is not only a back-end framework, but also a complete web development solution. It provides powerful back-end functions, such as routing, database operations, user authentication, etc., and supports front-end development, improving the development efficiency of the entire web application.

Laravel is suitable for web development, Python is suitable for data science and rapid prototyping. 1.Laravel is based on PHP and provides elegant syntax and rich functions, such as EloquentORM. 2. Python is known for its simplicity, widely used in Web development and data science, and has a rich library ecosystem.

Laravelcanbeeffectivelyusedinreal-worldapplicationsforbuildingscalablewebsolutions.1)ItsimplifiesCRUDoperationsinRESTfulAPIsusingEloquentORM.2)Laravel'secosystem,includingtoolslikeNova,enhancesdevelopment.3)Itaddressesperformancewithcachingsystems,en

Laravel's core functions in back-end development include routing system, EloquentORM, migration function, cache system and queue system. 1. The routing system simplifies URL mapping and improves code organization and maintenance. 2.EloquentORM provides object-oriented data operations to improve development efficiency. 3. The migration function manages the database structure through version control to ensure consistency. 4. The cache system reduces database queries and improves response speed. 5. The queue system effectively processes large-scale data, avoid blocking user requests, and improve overall performance.

Laravel performs strongly in back-end development, simplifying database operations through EloquentORM, controllers and service classes handle business logic, and providing queues, events and other functions. 1) EloquentORM maps database tables through the model to simplify query. 2) Business logic is processed in controllers and service classes to improve modularity and maintainability. 3) Other functions such as queue systems help to handle complex needs.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

Atom editor mac version download
The most popular open source editor
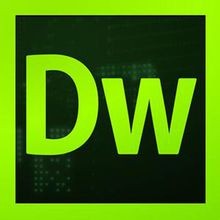
Dreamweaver CS6
Visual web development tools
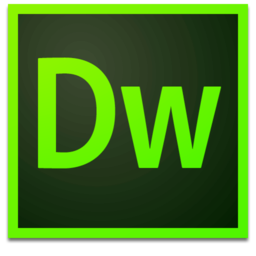
Dreamweaver Mac version
Visual web development tools