1. Calculate the sum of 1 2 3 ... 99
Idea: The remainder 2 is equal to 0 is an even number, remove it to get an odd number
public class Test1{ public static void main(String [] args){ //声明1到100奇数和的变量sum int sum = 0; for(int i=1;i<=100;i++){ if(i%2 != 0){ sum = sum+i; } } System.out.println("1到100奇数和是" + sum); } }
Free teaching video sharing: java learning
2. Read in an integer n less than 10 and output its factorial
Idea: Multiplication is the same as addition, j = i
public class Test2{ public static void main(String [] args){ Scanner input = new Scanner(System.in); System.out.println("输入小于10的整数n" ); //用户输入小于10的整数n int n = input.nextInt(); //声明循环的变量i int i ; //声明阶乘的的结果为变量j int j = 1; if(n>0){ for(i=1;i<=n;i++){ j = j*i; } System.out.println("这个数的阶乘是" + j); }else{ System.out.println("请输入小于10的整数,不能是0或负数" ); } } }
3. Find the sum of all numbers within 100 that are divisible by 3 but not divisible by 5
Idea: combination of looping and selection judgment
public class Test3{ public static void main(String [] args){ //声明和的变量sum int sum = 0; for(int i = 1;i<100;i++){ if(i%3 == 0 && i%5 != 0){ sum = sum+i; } } System.out.println("100以内能被3整除不能被5整除的数和是" + sum) ; } }
4 ."A hundred coins buys a hundred chickens" is a famous math problem in ancient my country
3 cents can buy a rooster, 2 cents can buy a hen, and 1 cent can buy 3 chicks. If you use 100 Wen to buy 100 chickens, how many roosters, hens, and chicks can you buy?
Idea: First understand the meaning of the question, nested loops, when buying (1-100/3) roosters, how many hens can be bought, and the remaining ones are chicks; don’t forget to make the final judgment There are three conditions: the number of chickens must be a multiple of 3, there must be 100 chickens, and 100 money must be spent.
public class Test4{ public static void main(String [] args){ //声明公鸡数量为变量a;母鸡数量为变量b;小鸡数量为变量c; int a; int b; int c; for(a=0;a<=100/3;a++){ for(b=0;b<=100/2;b++){ c= 100-(a+b); //小鸡数量必须是3的倍数,必须100只鸡,必须花100钱 if(c%3 == 0 && a+b+c == 100 && a*3+b*2+c/3 == 100){ System.out.println("公鸡,母鸡,小鸡的数量分别是" + a + "," +b + "," + c); } } } } } /*公鸡,母鸡,小鸡的数量分别是0,40,60 公鸡,母鸡,小鸡的数量分别是5,32,63 公鸡,母鸡,小鸡的数量分别是10,24,66 公鸡,母鸡,小鸡的数量分别是15,16,69 公鸡,母鸡,小鸡的数量分别是20,8,72 公鸡,母鸡,小鸡的数量分别是25,0,75 */
5. Output the multiplication table.
Idea: Nested loops can be done directly. Note here that the value range of the second number must be less than or equal to the first number
public class Test6{ public static void main(String [] args){ for(int i=1;i<10;i++){ for(int j=1;j<=i;j++){ System.out.print(j +"*"+ i + "=" + j*i + " "); } System.out.println(); } } }
6. Find the number of daffodils.
The so-called daffodil number refers to a three-digit number abc. If it satisfies a cubed b cubed c cubed = abc, then abc is the narcissus number.
Idea: It’s not difficult, as long as you are familiar with how to find each digit in the number
public class Test7{ public static void main(String [] args){ for(int n=100;n<1000;n++){ if((n/100)*(n/100)*(n/100)+(n/10%10)*(n/10%10)*(n/10%10)+(n%10)*(n%10)*(n%10) == n){ System.out.println(n); } } } } /* 153 370 371 407 */
7. What are the prime numbers within 100?
Prime number: A number that is not divisible by other numbers except 1 and itself. For example, 2, 3, 5, 7, 11
Idea: The outer loop goes through 2-100, the inner loop is an uncertain number of times, use while, if the remainder is not equal to zero, then continue to the next number until the remainder By itself, this number is a prime number
public class Test8 { public static void main(String[] args) { int j; for (int i = 2; i <= 100; i++) { j = 2; while (i % j != 0) { j++; } if (j == i) { System.out.println("100以内的质数有" + i); } } } }
Recommended related articles and tutorials:Introduction to java development
The above is the detailed content of Sharing some questions about loop structures in java. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
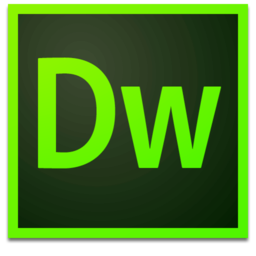
Dreamweaver Mac version
Visual web development tools
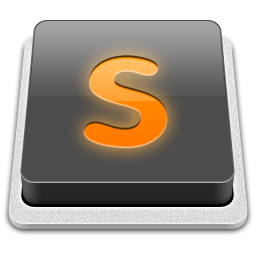
SublimeText3 Mac version
God-level code editing software (SublimeText3)

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
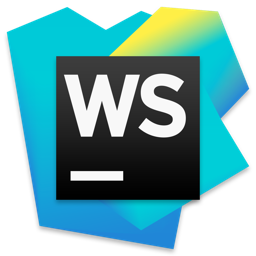
WebStorm Mac version
Useful JavaScript development tools