jquery determines the implementation code of the current browser_jquery
Written a method to determine the current browser type and version. It has only been tested on IE 8/11, Google, and 360 browser (incomplete). It requires the use of jquery
Core code:
;(function($, window, document,undefined){ if(!window.browser){ var userAgent = navigator.userAgent.toLowerCase(),uaMatch; window.browser = {} /** * 判断是否为ie */ function isIE(){ return ("ActiveXObject" in window); } /** * 判断是否为谷歌浏览器 */ if(!uaMatch){ uaMatch = userAgent.match(/chrome\/([\d.]+)/); if(uaMatch!=null){ window.browser['name'] = 'chrome'; window.browser['version'] = uaMatch[1]; } } /** * 判断是否为火狐浏览器 */ if(!uaMatch){ uaMatch = userAgent.match(/firefox\/([\d.]+)/); if(uaMatch!=null){ window.browser['name'] = 'firefox'; window.browser['version'] = uaMatch[1]; } } /** * 判断是否为opera浏览器 */ if(!uaMatch){ uaMatch = userAgent.match(/opera.([\d.]+)/); if(uaMatch!=null){ window.browser['name'] = 'opera'; window.browser['version'] = uaMatch[1]; } } /** * 判断是否为Safari浏览器 */ if(!uaMatch){ uaMatch = userAgent.match(/safari\/([\d.]+)/); if(uaMatch!=null){ window.browser['name'] = 'safari'; window.browser['version'] = uaMatch[1]; } } /** * 最后判断是否为IE */ if(!uaMatch){ if(userAgent.match(/msie ([\d.]+)/)!=null){ uaMatch = userAgent.match(/msie ([\d.]+)/); window.browser['name'] = 'ie'; window.browser['version'] = uaMatch[1]; }else{ /** * IE10 */ if(isIE() && !!document.attachEvent && (function(){"use strict";return !this;}())){ window.browser['name'] = 'ie'; window.browser['version'] = '10'; } /** * IE11 */ if(isIE() && !document.attachEvent){ window.browser['name'] = 'ie'; window.browser['version'] = '11'; } } } /** * 注册判断方法 */ if(!$.isIE){ $.extend({ isIE:function(){ return (window.browser.name == 'ie'); } }); } if(!$.isChrome){ $.extend({ isChrome:function(){ return (window.browser.name == 'chrome'); } }); } if(!$.isFirefox){ $.extend({ isFirefox:function(){ return (window.browser.name == 'firefox'); } }); } if(!$.isOpera){ $.extend({ isOpera:function(){ return (window.browser.name == 'opera'); } }); } if(!$.isSafari){ $.extend({ isSafari:function(){ return (window.browser.name == 'safari'); } }); } } })(jQuery, window, document);
How to use:
//使用方式 console.log(window.browser); console.log($.isIE()); console.log($.isChrome());
The complete test code specially provided by the editor of Script House:
<html> <head> <title>jquery 浏览器判断</title> </head> <body> <script src="http://demo.jb51.net/jslib/jquery/jquery-1.8.3.min.js"></script> <script type="text/javascript"> (function($, window, document,undefined){ if(!window.browser){ var userAgent = navigator.userAgent.toLowerCase(),uaMatch; window.browser = {} /** * 判断是否为ie */ function isIE(){ return ("ActiveXObject" in window); } /** * 判断是否为谷歌浏览器 */ if(!uaMatch){ uaMatch = userAgent.match(/chrome\/([\d.]+)/); if(uaMatch!=null){ window.browser['name'] = 'chrome'; window.browser['version'] = uaMatch[1]; } } /** * 判断是否为火狐浏览器 */ if(!uaMatch){ uaMatch = userAgent.match(/firefox\/([\d.]+)/); if(uaMatch!=null){ window.browser['name'] = 'firefox'; window.browser['version'] = uaMatch[1]; } } /** * 判断是否为opera浏览器 */ if(!uaMatch){ uaMatch = userAgent.match(/opera.([\d.]+)/); if(uaMatch!=null){ window.browser['name'] = 'opera'; window.browser['version'] = uaMatch[1]; } } /** * 判断是否为Safari浏览器 */ if(!uaMatch){ uaMatch = userAgent.match(/safari\/([\d.]+)/); if(uaMatch!=null){ window.browser['name'] = 'safari'; window.browser['version'] = uaMatch[1]; } } /** * 最后判断是否为IE */ if(!uaMatch){ if(userAgent.match(/msie ([\d.]+)/)!=null){ uaMatch = userAgent.match(/msie ([\d.]+)/); window.browser['name'] = 'ie'; window.browser['version'] = uaMatch[1]; }else{ /** * IE10 */ if(isIE() && !!document.attachEvent && (function(){"use strict";return !this;}())){ window.browser['name'] = 'ie'; window.browser['version'] = '10'; } /** * IE11 */ if(isIE() && !document.attachEvent){ window.browser['name'] = 'ie'; window.browser['version'] = '11'; } } } /** * 注册判断方法 */ if(!$.isIE){ $.extend({ isIE:function(){ return (window.browser.name == 'ie'); } }); } if(!$.isChrome){ $.extend({ isChrome:function(){ return (window.browser.name == 'chrome'); } }); } if(!$.isFirefox){ $.extend({ isFirefox:function(){ return (window.browser.name == 'firefox'); } }); } if(!$.isOpera){ $.extend({ isOpera:function(){ return (window.browser.name == 'opera'); } }); } if(!$.isSafari){ $.extend({ isSafari:function(){ return (window.browser.name == 'safari'); } }); } } })(jQuery, window, document); //使用方式 alert(window.browser.name); //下面是ie F2中测试可以看到效果 console.log(window.browser); console.log($.isIE()); console.log($.isChrome()); </script> </body> </html>
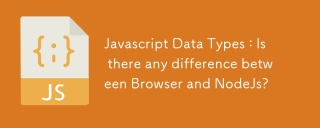
JavaScript core data types are consistent in browsers and Node.js, but are handled differently from the extra types. 1) The global object is window in the browser and global in Node.js. 2) Node.js' unique Buffer object, used to process binary data. 3) There are also differences in performance and time processing, and the code needs to be adjusted according to the environment.
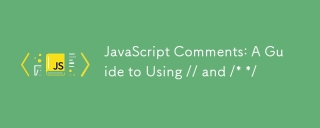
JavaScriptusestwotypesofcomments:single-line(//)andmulti-line(//).1)Use//forquicknotesorsingle-lineexplanations.2)Use//forlongerexplanationsorcommentingoutblocksofcode.Commentsshouldexplainthe'why',notthe'what',andbeplacedabovetherelevantcodeforclari
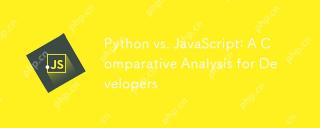
The main difference between Python and JavaScript is the type system and application scenarios. 1. Python uses dynamic types, suitable for scientific computing and data analysis. 2. JavaScript adopts weak types and is widely used in front-end and full-stack development. The two have their own advantages in asynchronous programming and performance optimization, and should be decided according to project requirements when choosing.
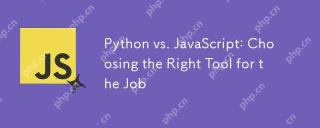
Whether to choose Python or JavaScript depends on the project type: 1) Choose Python for data science and automation tasks; 2) Choose JavaScript for front-end and full-stack development. Python is favored for its powerful library in data processing and automation, while JavaScript is indispensable for its advantages in web interaction and full-stack development.
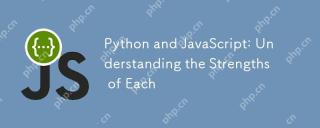
Python and JavaScript each have their own advantages, and the choice depends on project needs and personal preferences. 1. Python is easy to learn, with concise syntax, suitable for data science and back-end development, but has a slow execution speed. 2. JavaScript is everywhere in front-end development and has strong asynchronous programming capabilities. Node.js makes it suitable for full-stack development, but the syntax may be complex and error-prone.
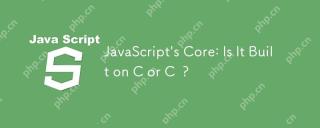
JavaScriptisnotbuiltonCorC ;it'saninterpretedlanguagethatrunsonenginesoftenwritteninC .1)JavaScriptwasdesignedasalightweight,interpretedlanguageforwebbrowsers.2)EnginesevolvedfromsimpleinterpreterstoJITcompilers,typicallyinC ,improvingperformance.
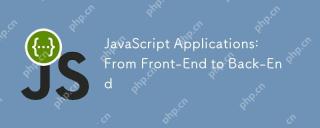
JavaScript can be used for front-end and back-end development. The front-end enhances the user experience through DOM operations, and the back-end handles server tasks through Node.js. 1. Front-end example: Change the content of the web page text. 2. Backend example: Create a Node.js server.
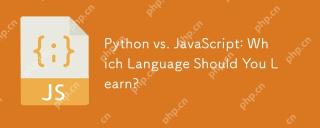
Choosing Python or JavaScript should be based on career development, learning curve and ecosystem: 1) Career development: Python is suitable for data science and back-end development, while JavaScript is suitable for front-end and full-stack development. 2) Learning curve: Python syntax is concise and suitable for beginners; JavaScript syntax is flexible. 3) Ecosystem: Python has rich scientific computing libraries, and JavaScript has a powerful front-end framework.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use
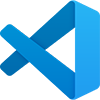
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

Notepad++7.3.1
Easy-to-use and free code editor

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
