Introduction to the method of determining whether a variable is a number in java
Java method to determine whether it is a number: (Recommended: java video tutorial)
1. Use regular expressions
First, import java.util.regex.Pattern and java.util.regex.Matcher
/** * 利用正则表达式判断字符串是否是数字 * @param str * @return */ public boolean isNumeric(String str){ Pattern pattern = Pattern.compile("[0-9]*"); Matcher isNum = pattern.matcher(str); if( !isNum.matches() ){ return false; } return true; }
2. Use the functions that come with JAVA
public static boolean isNumeric(String str) { for (int i = 0; i < str.length(); i++) { System.out.println(str.charAt(i)); if (!Character.isDigit(str.charAt(i))) { return false; } } return true; }
3. Use org.apache.commons. lang
org.apache.commons.lang.StringUtils; boolean isNunicodeDigits=StringUtils.isNumeric("aaa123456789"); http://jakarta.apache.org/commons/lang/api-release/index.html下面的解释: public static boolean isNumeric(String str)Checks if the String contains only unicode digits. A decimal point is not a unicode digit and returns false. null will return false. An empty String ("") will return true. StringUtils.isNumeric(null) = false StringUtils.isNumeric("") = true StringUtils.isNumeric(" ") = false StringUtils.isNumeric("123") = true StringUtils.isNumeric("12 3") = false StringUtils.isNumeric("ab2c") = false StringUtils.isNumeric("12-3") = false StringUtils.isNumeric("12.3") = false
4. Determine the ASCII code value
public static boolean isNumeric0(String str) { for(int i=str.length();--i>=0;) { int chr=str.charAt(i); if(chr<48 || chr>57) return false; } return true; }
5. Determine whether the characters in str are 0-9 one by one
public static boolean isNumeric3(String str) { final String number = "0123456789"; for(int i = 0;i < number.length; i ++) { if(number.indexOf(str.charAt(i)) == -1) { return false; } } return true; }
6. Capture the NumberFormatException exception
public static boolean isNumeric00(String str) { try{ Integer.parseInt(str); return true; }catch(NumberFormatException e) { System.out.println("异常:\"" + str + "\"不是数字/整数..."); return false; } }
For more java knowledge, please pay attention to the java basic tutorial column.
The above is the detailed content of Introduction to the method of determining whether a variable is a number in java. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
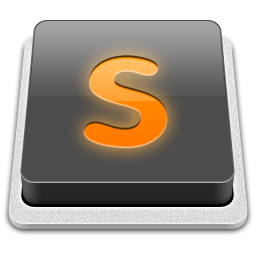
SublimeText3 Mac version
God-level code editing software (SublimeText3)

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

Atom editor mac version download
The most popular open source editor