1. Character conversion
var s1 = "01"; var s2 = "1.1"; var s3 = "z";//字母'z'无法转换为数字,所以或返回NaN var b = false; var f = 1.1; var o = { valueOf: function() { return -1; } }; s1 = -s1; //value becomes numeric -1 s2 = -s2; //value becomes numeric -1.1 s3 = -s3; //value becomes NaN b = -b; //value becomes numeric 0 f = -f; //change to -1.1 o = -o; // 1 先执行对象的valueOf()方法返回-1,--1为1 o = +o;//-1 o的valueOf()值为-1,+-1还是-1
2. Special character operations
var result1 = 5 - true; //4 because true is converted to 1 var result2 = NaN - 1; //NaN NaN不是一个数字,和任何数字做任何运算都是NaN var result3 = 5 - 3; //2 var result4 = 5 - ""; //5 because "" is converted to 0 var result5 = 5 - "2"; //3 because "2" is converted to 2 var result6 = 5 - null; //5 because null is converted to 0
3. Variable to string operation
var value1 = 10; var value2 = true; var value3 = null; var value4;//value4 没有赋值就是underfined 转换为字符串就是'underfined' alert(String(value1)); //"10" alert(String(value2)); //"true" alert(String(value3)); //"null" alert(String(value4)); //"undefined"
4. Number conversion
var num = 10; alert(num.toString()); //"10"默认十进制 alert(num.toString(2)); //"1010"二进制 alert(num.toString(8)); //"12"八进制 alert(num.toString(10)); //"10"十进制 alert(num.toString(16)); //"a"十六进制
5. String comparison operation
var result1 = 5 > 3; //true var result2 = 5 < 3; //false var result3 = "Brick" < "alphabet"; //true 字符串比较按照字母表顺序进行比较 小写字母在大写字母后面 var result4 = "Brick".toLowerCase() < "alphabet".toLowerCase(); //false字母表顺序比较 var result5 = "23" < "3"; //true 字符2小于字符3 var result6 = "23" < 3; //false 此时'23'转换成23了 var result7 = "a" < 3; //false because "a" becomes NaN 字符'a'无法转换成数字 var result8 = NaN < 3; //false NaN 和任何数字比较,都无法转换成数字,所以一直是false var result9 = NaN >= 3; //false
6. Character conversion
var num1 = parseInt("AF", 16); //175 按照16进制输出十进制数据 10*16+15*1 var num2 = parseInt("AF"); //NaN 没有指定进制,默认按照10进制转换,由于AF不在十进制范围,返回NaN alert(num1); alert(num2);
7. Use of parseInt
var num1 = parseInt("1234blue"); //1234 var num2 = parseInt(""); //NaN 字符''无法转换成数字 var num3 = parseInt("0xA"); //10 - hexadecimal 16进制的A var num4 = parseInt(22.5); //22 var num5 = parseInt("70"); //70 - decimal var num6 = parseInt("0xf"); //15 16进制为15
8. Use of Number objects
var num1 = Number("Hello world!"); //NaN var num2 = Number(""); //0 空字符串可以转换成0 这个parseInt()不一样 var num3 = Number("000011"); //11 var num4 = Number(true); //1
9. NaN usage
alert(NaN == NaN); //false alert(isNaN(NaN)); //true alert(isNaN(10)); //false ?10 is a number alert(isNaN("10")); //false ?can be converted to number 10 alert(isNaN("blue")); //true ?cannot be converted to a number alert(isNaN(true)); //false ?can be converted to number 1
10. System maximum number
var result = Number.MAX_VALUE + 1; alert(isFinite(result)); // false
11. Infinity
alert(5 * 6); //30 alert(5 * NaN); //NaN alert(Infinity * 0); //NaN alert(Infinity * 2); //Infinity alert("5" * 5); //25 alert(true * 10); //10 alert(false * 10); //0 alert(26 % 5); //1 alert(Infinity % 3); //NaN alert(3 % 0); //NaN alert(5 % Infinity); //5 alert(0 % 10); //0 alert(true % 25); //1 alert(3 % false); //NaN
12. for in loop
for (var propName in window) { document.write(propName); document.write("<br />"); }
13. Comparison of special characters
alert(null == undefined); //true alert(null === undefined); //false alert("NaN" == NaN); //false alert("NaN" === NaN); //false alert(NaN == NaN); //false alert(NaN === NaN); //false alert(NaN != NaN); //true alert(NaN !== NaN); //true alert(false == 0); //true alert(false === 0); //false alert(true == 1); //true alert(true === 1); //false alert(null == 0); //false alert(undefined == 0); //false alert(5 == "5"); //true alert(5 === "5"); //false
14. Boolean object
var message = "Hello world!"; var messageAsBoolean = Boolean(message); alert(messageAsBoolean); //true
15. Use for loop with tags
Both the break statement and the continue statement can be used in conjunction with labeled statements to return to a specific location in the code.
Usually this is done when there is a loop inside a loop, for example:
var num = 0; //返回到特定的位置 outermost: for (var i=0; i < 10; i++) { for (var j=0; j < 10; j++) { if (i == 5 && j == 5) { break outermost; } num++; } } alert(num); //55
In the above example, the label outermost represents the first for statement. Normally, each for statement executes the code block 10 times, which means that num will be executed 100 times under normal circumstances, and when the execution is completed, num should equal 100. The break statement here has one parameter, which is the label of the statement to jump to after stopping the loop. In this way, the break statement can not only jump out of the inner for statement (that is, the statement that uses variable j), but also jump out of the outer for statement (that is, the statement that uses variable i). Therefore, the final value of num is 55, because the loop will terminate when the values of i and j are both equal to 5.
The continue statement can be used in the same way:
var iNum = 0; outermost: for (var i=0; i<10; i++) { for (var j=0; j<10; j++) { if (i == 5 && j == 5) { continue outermost; } iNum++; } } alert(iNum); //输出 "95"
In the above example, the continue statement will force the loop to continue, not only the inner loop, but also the outer loop. This occurs when j equals 5, meaning that the inner loop will be reduced by 5 iterations, resulting in iNum having a value of 95.
Tip: As you can see, labeled statements used in conjunction with break and continue are very powerful, but overusing them can cause trouble when debugging your code. Make sure the labels you use are descriptive and don't nest too many levels of loops.
The above is today’s summary of JavaScript learning, and it will continue to be updated every day. I hope you will continue to pay attention.
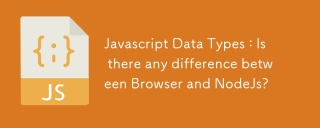
JavaScript core data types are consistent in browsers and Node.js, but are handled differently from the extra types. 1) The global object is window in the browser and global in Node.js. 2) Node.js' unique Buffer object, used to process binary data. 3) There are also differences in performance and time processing, and the code needs to be adjusted according to the environment.
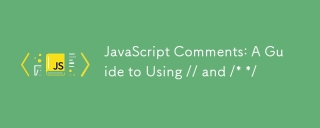
JavaScriptusestwotypesofcomments:single-line(//)andmulti-line(//).1)Use//forquicknotesorsingle-lineexplanations.2)Use//forlongerexplanationsorcommentingoutblocksofcode.Commentsshouldexplainthe'why',notthe'what',andbeplacedabovetherelevantcodeforclari
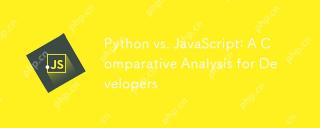
The main difference between Python and JavaScript is the type system and application scenarios. 1. Python uses dynamic types, suitable for scientific computing and data analysis. 2. JavaScript adopts weak types and is widely used in front-end and full-stack development. The two have their own advantages in asynchronous programming and performance optimization, and should be decided according to project requirements when choosing.
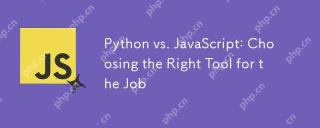
Whether to choose Python or JavaScript depends on the project type: 1) Choose Python for data science and automation tasks; 2) Choose JavaScript for front-end and full-stack development. Python is favored for its powerful library in data processing and automation, while JavaScript is indispensable for its advantages in web interaction and full-stack development.
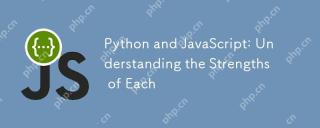
Python and JavaScript each have their own advantages, and the choice depends on project needs and personal preferences. 1. Python is easy to learn, with concise syntax, suitable for data science and back-end development, but has a slow execution speed. 2. JavaScript is everywhere in front-end development and has strong asynchronous programming capabilities. Node.js makes it suitable for full-stack development, but the syntax may be complex and error-prone.
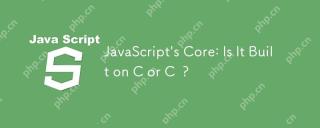
JavaScriptisnotbuiltonCorC ;it'saninterpretedlanguagethatrunsonenginesoftenwritteninC .1)JavaScriptwasdesignedasalightweight,interpretedlanguageforwebbrowsers.2)EnginesevolvedfromsimpleinterpreterstoJITcompilers,typicallyinC ,improvingperformance.
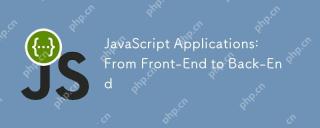
JavaScript can be used for front-end and back-end development. The front-end enhances the user experience through DOM operations, and the back-end handles server tasks through Node.js. 1. Front-end example: Change the content of the web page text. 2. Backend example: Create a Node.js server.
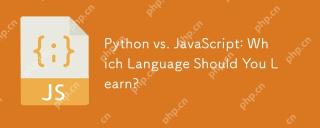
Choosing Python or JavaScript should be based on career development, learning curve and ecosystem: 1) Career development: Python is suitable for data science and back-end development, while JavaScript is suitable for front-end and full-stack development. 2) Learning curve: Python syntax is concise and suitable for beginners; JavaScript syntax is flexible. 3) Ecosystem: Python has rich scientific computing libraries, and JavaScript has a powerful front-end framework.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
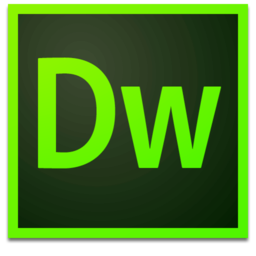
Dreamweaver Mac version
Visual web development tools

Zend Studio 13.0.1
Powerful PHP integrated development environment
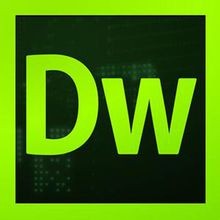
Dreamweaver CS6
Visual web development tools
