Principle of quick sort
Quick sort is an improvement on bubble sort. Bubble sort is to compare small values one by one. One end, and the larger value is placed on the other end to achieve the purpose of sorting.
Quick sorting is to first select a critical value, put the values smaller than the critical value on one end, and put the values larger than the critical value on the other end. . Repeat the method in the previous paragraph, and you can divide the two sides that have passed the critical value and divide them twice... After sorting the data, the entire quick sort is completed.
Quick Sort Algorithm
Core Algorithm:
//QuickSort while(i < j) { while(num[j] > tmp && j > i) --j; while(num[i] <= tmp && i < j) { ++i; } if(i < j) { t = num[i]; num[i] = num[j]; num[j] = t; } } num[left] = num[i]; num[i] = tmp;
The following is the complete QuickSort program:
//QuickSort.java public class QuickSort { public static void main(String[] args) { int[] num = {10, 9, 8, 7, 6, 5, 4, 3, 2, 1}; System.out.print("Qriginal array is:"); for (int i = 0; i < num.length; i++) { System.out.print(num[i] + " "); } System.out.println(); //QuickSort quicksort(num, 0, 9); System.out.print("Sorted array is:"); for (int i = 0; i < num.length; i++) { System.out.print(num[i] + " "); } System.out.println(); } public static void quicksort(int[] num, int left, int right) { if(left > right) return; int tmp, i, j, t; tmp = num[left]; i = left; j = right; while(i < j) { while(num[j] > tmp && j > i) --j; while(num[i] <= tmp && i < j) { ++i; } if(i < j) { t = num[i]; num[i] = num[j]; num[j] = t; } } num[left] = num[i]; num[i] = tmp; quicksort(num, left, i - 1); quicksort(num, i + 1, right); } }
The program output is shown in the figure below:
Qriginal array is:10 9 8 7 6 5 4 3 2 1 Sorted array is:1 2 3 4 5 6 7 8 9 10
Quick sorting is more efficient than other sorting methods, so quick sorting is the best general sorting method now. The time complexity of QuickSort is O(nlogn).
The above is the detailed content of Quick sort in Java. For more information, please follow other related articles on the PHP Chinese website!
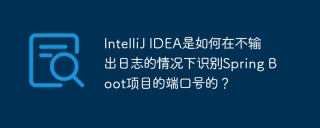
Start Spring using IntelliJIDEAUltimate version...
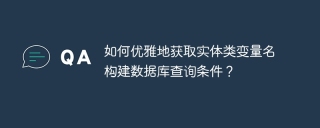
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
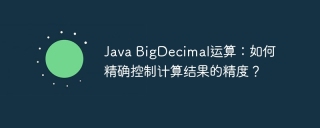
Java...
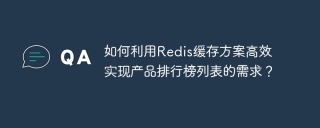
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
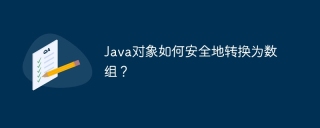
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
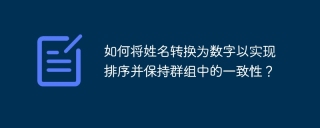
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
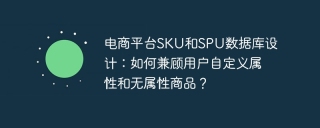
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
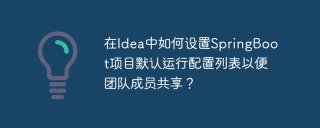
How to set the SpringBoot project default run configuration list in Idea using IntelliJ...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
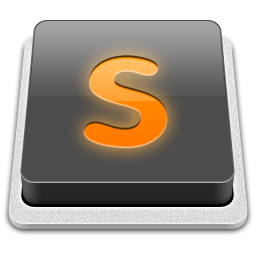
SublimeText3 Mac version
God-level code editing software (SublimeText3)

Atom editor mac version download
The most popular open source editor

Notepad++7.3.1
Easy-to-use and free code editor

Zend Studio 13.0.1
Powerful PHP integrated development environment