1: sys is a built-in module of python.
Use the import statement to enter the sys module.
Related recommendations: "python Video"
When import sys is executed, python is listed in the directory in the sys.path variable Find the sys module file. Then run the statements in the main block of this module to initialize it, and then you can use the module.
2: Common functions of the sys module
You can use the dir() method to view the methods available in the module. The results are as follows. I have not used many of them, so I just keep them simple. Introduce several methods that I have used.
$ python Python 2.7.6 (default, Oct 26 2016, 20:30:19) [GCC 4.8.4] on linux2 Type "help", "copyright", "credits" or "license" for more information. >>> import sys >>> dir(sys) ['__displayhook__', '__doc__', '__excepthook__', '__name__', '__package__', '__stderr__', '__stdin__', '__stdout__', '_clear_type_cache', '_current_frames', '_getframe', '_mercurial', '_multiarch', 'api_version', 'argv', 'builtin_module_names', 'byteorder', 'call_tracing', 'callstats', 'copyright', 'displayhook', 'dont_write_bytecode', 'exc_clear', 'exc_info', 'exc_type', 'excepthook', 'exec_prefix', 'executable', 'exit', 'flags', 'float_info', 'float_repr_style', 'getcheckinterval', 'getdefaultencoding', 'getdlopenflags', 'getfilesystemencoding', 'getprofile', 'getrecursionlimit', 'getrefcount', 'getsizeof', 'gettrace', 'hexversion', 'long_info', 'maxint', 'maxsize', 'maxunicode', 'meta_path', 'modules', 'path', 'path_hooks', 'path_importer_cache', 'platform', 'prefix', 'ps1', 'ps2', 'py3kwarning', 'pydebug', 'setcheckinterval', 'setdlopenflags', 'setprofile', 'setrecursionlimit', 'settrace', 'stderr', 'stdin', 'stdout', 'subversion', 'version', 'version_info', 'warnoptions']
(1) sys.argv implements passing parameters from outside the program to the program
sys.argv variable is a string containing command line parameters List, use the command line to pass parameters to the program. Among them, the name of the script is always the first parameter in the sys.argv list.
(2) sys.path contains a list of directory names of input modules.
Get the string collection of the specified module search path. You can put the written module under a certain path, and you can find it correctly when importing in the program. When importing module_name, module.name is searched based on the path of sys.path. You can also customize the module path.
sys.path.append("Custom module path")
(3) sys.exit([arg]) Exit in the middle of the program, arg=0 means normal exit
Generally, the interpreter automatically exits when the execution reaches the end of the main program. However, if you need to exit the program midway, you can call the sys.exit function with an optional integer parameter returned to the calling program, indicating that you can The call to sys.exit is captured in the main program. (0 means normal exit, others are exceptions) Of course, you can also use string parameters to indicate unsuccessful error messages.
(4) sys.modules
sys.modules is a global dictionary, which is loaded in memory after python is started. Whenever a programmer imports a new module, sys.modules will automatically record the module. When the module is imported for the second time, Python will directly look it up in the dictionary, thus speeding up the program. It has all the methods that the dictionary has.
(5) sys.getdefaultencoding() / sys.setdefaultencoding() / sys.getfilesystemencoding()
sys.getdefaultencoding()
Get the current encoding of the system, which generally defaults to ascii.
sys.setdefaultencoding()
Set the system default encoding. You will not see this method when executing dir (sys). If the execution fails in the interpreter, you can execute reload (sys) first. , after executing setdefaultencoding('utf8'), the system default encoding is set to utf8. (See setting the system default encoding)
sys.getfilesystemencoding()
Get the encoding used by the file system. It returns 'mbcs' under Windows and 'utf-8' under mac
(6) sys.stdin, sys.stdout, sys.stderr
The stdin, stdout, and stderr variables contain stream objects corresponding to standard I/O streams. If you need better control over the output, and print doesn't meet your requirements, they are what you need. You can also replace them, and then you can redirect output and input to other devices (device), or process them in non-standard ways.
(7) sys.platform
Get the current system platform. Such as: win32, Linux, etc.
3: Example
(1) sys.argv sys.path
$ cat sys-test.py #!/usr/bin/python import sys print 'The command line arguments are:' for i in sys.argv: print i print '\n\nThe PYTHONPATH is', sys.path, '\n'
Run result:
$ python sys-test.py my name is ubuntu The command line arguments are: sys-test.py my name is ubuntu The PYTHONPATH is ['/work/python-practice', '/usr/lib/python2.7', '/usr/lib/python2.7/plat-x86_64-linux-gnu', '/usr/lib/python2.7/lib-tk', '/usr/lib/python2.7/lib-old', '/usr/lib/python2.7/lib-dynload', '/usr/local/lib/python2.7/dist-packages', '/usr/lib/python2.7/dist-packages', '/usr/lib/python2.7/dist-packages/PILcompat', '/usr/lib/python2.7/dist-packages/gtk-2.0', '/usr/lib/pymodules/python2.7', '/usr/lib/python2.7/dist-packages/ubuntu-sso-client']
(2) sys.exit
import sys def exitfunc(value): print (value) sys.exit(0) print("hello") try: sys.exit(90) except SystemExit as value: exitfunc(value) print("come?")
Running results:
hello 90
The program first prints hello, then executes exit(90), throws an exception and passes 90 to values. Values is executed in the passed function and prints 90. The program exits. The following "come?" will not be printed because it has already exited. If you remove sys.exit(0) in the exitfunc function at this time, the program will continue to execute until "come?" is output.
(3) sys.modules
sys.modules.keys() returns a list of all imported modules
keys is the module name
values is the module
modules return path
import sys print(sys.modules.keys()) print("**************************************************************************") print(sys.modules.values()) print("**************************************************************************") print(sys.modules["os"])
Run result:
['copy_reg', 'sre_compile', '_sre', 'encodings', 'site', '__builtin__', 'sysconfig', '__main__', 'encodings.encodings', 'abc', 'posixpath', '_weakrefset', 'errno', 'encodings.codecs', 'sre_constants', 're', '_abcoll', 'types', '_codecs', 'encodings.__builtin__', '_warnings', 'genericpath', 'stat', 'zipimport', '_sysconfigdata', 'warnings', 'UserDict', 'encodings.ascii', 'sys', 'codecs', '_sysconfigdata_nd', 'os.path', 'sitecustomize', 'signal', 'traceback', 'linecache', 'posix', 'encodings.aliases', 'exceptions', 'sre_parse', 'os', '_weakref'] ******************************************************************************* [<module 'copy_reg' from '/usr/lib/python2.7/copy_reg.pyc'>, <module 'sre_compile' from '/usr/lib/python2.7/sre_compile.pyc'>, <module '_sre' (built-in)>, <module 'encodings' from '/usr/lib/python2.7/encodings/__init__.pyc'>, <module 'site' from '/usr/lib/python2.7/site.pyc'>, <module '__builtin__' (built-in)>, <module 'sysconfig' from '/usr/lib/python2.7/sysconfig.pyc'>, <module '__main__' from 'sys-test1.py'>, None, <module 'abc' from '/usr/lib/python2.7/abc.pyc'>, <module 'posixpath' from '/usr/lib/python2.7/posixpath.pyc'>, <module '_weakrefset' from '/usr/lib/python2.7/_weakrefset.pyc'>, <module 'errno' (built-in)>, None, <module 'sre_constants' from '/usr/lib/python2.7/sre_constants.pyc'>, <module 're' from '/usr/lib/python2.7/re.pyc'>, <module '_abcoll' from '/usr/lib/python2.7/_abcoll.pyc'>, <module 'types' from '/usr/lib/python2.7/types.pyc'>, <module '_codecs' (built-in)>, None, <module '_warnings' (built-in)>, <module 'genericpath' from '/usr/lib/python2.7/genericpath.pyc'>, <module 'stat' from '/usr/lib/python2.7/stat.pyc'>, <module 'zipimport' (built-in)>, <module '_sysconfigdata' from '/usr/lib/python2.7/_sysconfigdata.pyc'>, <module 'warnings' from '/usr/lib/python2.7/warnings.pyc'>, <module 'UserDict' from '/usr/lib/python2.7/UserDict.pyc'>, <module 'encodings.ascii' from '/usr/lib/python2.7/encodings/ascii.pyc'>, <module 'sys' (built-in)>, <module 'codecs' from '/usr/lib/python2.7/codecs.pyc'>, <module '_sysconfigdata_nd' from '/usr/lib/python2.7/plat-x86_64-linux-gnu/_sysconfigdata_nd.pyc'>, <module 'posixpath' from '/usr/lib/python2.7/posixpath.pyc'>, <module 'sitecustomize' from '/usr/lib/python2.7/sitecustomize.pyc'>, <module 'signal' (built-in)>, <module 'traceback' from '/usr/lib/python2.7/traceback.pyc'>, <module 'linecache' from '/usr/lib/python2.7/linecache.pyc'>, <module 'posix' (built-in)>, <module 'encodings.aliases' from '/usr/lib/python2.7/encodings/aliases.pyc'>, <module 'exceptions' (built-in)>, <module 'sre_parse' from '/usr/lib/python2.7/sre_parse.pyc'>, <module 'os' from '/usr/lib/python2.7/os.pyc'>, <module '_weakref' (built-in)>] ******************************************************************************* <module 'os' from '/usr/lib/python2.7/os.pyc'>
(4) sys.stdin/sys.stdout/sys.stderr
stdin, stdout, stderr are in All file attribute objects in Python are automatically related to the standard input, output, and errors in the shell environment when python is started. The I/O redirection of the python program in the shell is provided by the shell and has nothing to do with python itself. Relationship. The python program internally redirects stdin, stdout, and stderr read and write operations to an internal object.
Standard input
import sys #print('Hi, %s!' %input('Please enter your name: ')) python3.*版本用input print('Hi, %s!' %raw_input('Please enter your name: ')) #python2.*版本用raw_input 运行结果: Please enter your name: er Hi, er! 等同于: #!/usr/bin/python import sys print('Please enter your name:') name=sys.stdin.readline()[:-1] print('Hi, %s!' %name) 标准输出 print('Hello World!\n') 等同于: #!/usr/bin/python import sys sys.stdout.write('output resule is good!\n') 其他实验 #!/usr/bin/python import sys for i in (sys.stdin, sys.stdout, sys.stderr): print(i)
Execution result:
python sys-test1.py <open file '<stdin>', mode 'r' at 0x7fa4e630f0c0> <open file '<stdout>', mode 'w' at 0x7fa4e630f150> <open file '<stderr>', mode 'w' at 0x7fa4e630f1e0>
The above is the detailed content of Basic introduction to python sys module. For more information, please follow other related articles on the PHP Chinese website!
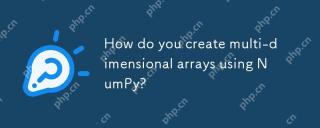
Create multi-dimensional arrays with NumPy can be achieved through the following steps: 1) Use the numpy.array() function to create an array, such as np.array([[1,2,3],[4,5,6]]) to create a 2D array; 2) Use np.zeros(), np.ones(), np.random.random() and other functions to create an array filled with specific values; 3) Understand the shape and size properties of the array to ensure that the length of the sub-array is consistent and avoid errors; 4) Use the np.reshape() function to change the shape of the array; 5) Pay attention to memory usage to ensure that the code is clear and efficient.
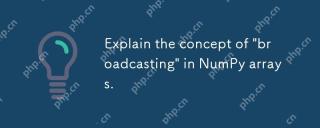
BroadcastinginNumPyisamethodtoperformoperationsonarraysofdifferentshapesbyautomaticallyaligningthem.Itsimplifiescode,enhancesreadability,andboostsperformance.Here'showitworks:1)Smallerarraysarepaddedwithonestomatchdimensions.2)Compatibledimensionsare
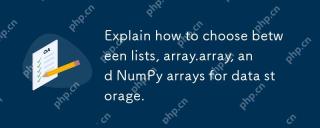
ForPythondatastorage,chooselistsforflexibilitywithmixeddatatypes,array.arrayformemory-efficienthomogeneousnumericaldata,andNumPyarraysforadvancednumericalcomputing.Listsareversatilebutlessefficientforlargenumericaldatasets;array.arrayoffersamiddlegro
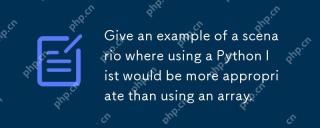
Pythonlistsarebetterthanarraysformanagingdiversedatatypes.1)Listscanholdelementsofdifferenttypes,2)theyaredynamic,allowingeasyadditionsandremovals,3)theyofferintuitiveoperationslikeslicing,but4)theyarelessmemory-efficientandslowerforlargedatasets.
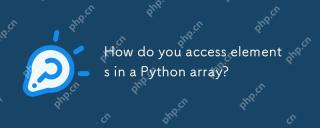
ToaccesselementsinaPythonarray,useindexing:my_array[2]accessesthethirdelement,returning3.Pythonuseszero-basedindexing.1)Usepositiveandnegativeindexing:my_list[0]forthefirstelement,my_list[-1]forthelast.2)Useslicingforarange:my_list[1:5]extractselemen
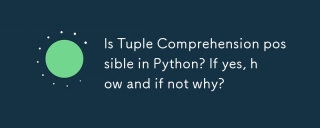
Article discusses impossibility of tuple comprehension in Python due to syntax ambiguity. Alternatives like using tuple() with generator expressions are suggested for creating tuples efficiently.(159 characters)
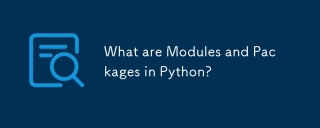
The article explains modules and packages in Python, their differences, and usage. Modules are single files, while packages are directories with an __init__.py file, organizing related modules hierarchically.
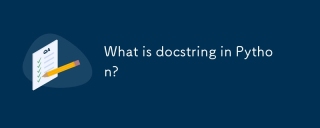
Article discusses docstrings in Python, their usage, and benefits. Main issue: importance of docstrings for code documentation and accessibility.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Zend Studio 13.0.1
Powerful PHP integrated development environment
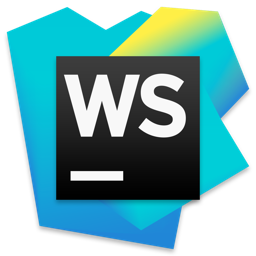
WebStorm Mac version
Useful JavaScript development tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
