Detailed introduction to javaScript reference types (with examples)
This article brings you a detailed introduction to javaScript reference types (with examples). It has certain reference value. Friends in need can refer to it. I hope it will be helpful to you.
Concept: A reference type is a data structure used to organize data and functions together, that is, a class;
Objects are special reference type instances. New objects are created by adding a constructor after new.
Constructor: Function used to create objects.
Example: var person = new Object();
object is a constructor that provides default properties and methods for new objects.
1. Object type
//Common method
var person = new Object(); person.name = "syr"; person.age = 22;
//Object literal
var person = { name : "syr", age : 22, 5 : true //5自动转为"5" } * 数值属性名会自动转为字符串; * 对象字面量推荐只在属性名可读的情况下使用,也是向函数传递大量参数首选方式,必须使用命名参数,对象字面量封装多个可选参数。 * 访问对象一般用点表示法,js中也可以用方括号法,将要访问的属性以字符串的形式放进去。
Example: alert(person["name"]) ; // Equal to person.name
* 方括号法主要优点是可以通过变量来访问属性。 * 点表示法属性名不能包含错误的自负或者保留字和关键字,但方括号法可以。 * 通常,除非必须使用变量来访问属性,否则推荐使用点表示法。
2. Array type: Each item of the array in
* 数组:数据的有序列表。
es can save any type of data, and the array size can also be dynamically adjusted.
方法一 : new可以省略; var colors = new Array(); 方法二 : 数组字面量 var colors = ["red","blue","green"];
Like objects, literals do not call the Array constructor.
var colors = ["red","blue","green"]; colors[0] // 显示第一项 colors[2] = "black"; // 修改第二项 colors[3] = "brown" ; //增加第四项 colors.length = "black" ; 增加一项
The length property returns the length of the array and can add and remove items from the array.
* 检测数组 value instanceof Array // 判断是否为数组 Array.isArray(value) // isArray确定到底是不是数组,不管在哪个环境中创建 * 转换方法
All objects have the toLocalString() method. When tostring() is called in the background, null and undefined return results are represented by empty strings.
* 栈方法 : 后进先出(吃了吐)
push(): accepts any number of parameters and adds them to the end;
pop(): removes an item from the end of the array.
* 队列方法 : 先进先出(吃了拉);
shift(): The front end removes the item and returns the item, and the length is reduced by 1;
unshift(): The front end pushes an item;
* 重排序方法
reverse(): Reverse order;
sort(): order;
compares strings and will be automatically converted to strings.
For example, "10" is in front of "5", so a comparison function should be passed to sort.
function compare(value1,value2){ if(value1 value2){ return 1; }else{ return 0; } } value = [0,1,5,10,15];value.sort(value); //0,1,5,10,15 * 操作方法
concat() splicing: First make a copy of the original array, and then add the parameters to the end. The original array remains unchanged. slice(1,2): Cut: Return the starting position of parameter 1, parameter 2 is the end position, wrap the front and not the back, and the original array remains unchanged. solice(): Mainly inserts items into the array. (The most important method of array operation):
-Delete: You can delete any number of items, specify two parameters: arg1 is the position to be deleted, arg2 is the number of items to be deleted, for example: splice(0,2): Delete the first two items;
-insert: three parameters. Starting position, the number of items to be deleted and the items to be inserted. Multiple items can be inserted later. Example: solice(2,0,"red","green");Insert "red" and "green" from position 2.
-Replacement: Same as above, the number of inserted items does not have to be equal to the number of deleted items.
Note: splice() will change the original array;
* 位置方法
indexof(): search from the beginning
lastIndexof(): never search
arg1 represents the item to be searched, arg2 is the starting point Position (optional parameter), -1 is returned if not found, and congruence is used when comparing.
var number = [1,2,3,4,5,4,3,2,1]; number.indexof(4) ; //返回5 * 迭代方法
every();
filter();
forEach();
map();
* 归并方法
reduce() : from the beginning
reduceRight ; from The tail
will iterate through all items in the array and construct a final return item.
3. Date type
var now = new Data(); date.parse("May 25,2004"); // 新建特定日期 * 继承: 重写了3个方法
toLocalString(): browser setting date;
toString();
valueof();
* 日期格式化方法:
-toDateString( ): Display the week, month, day, and year in a specific format;
-toTimeString(): Hours, minutes, seconds, time zone;
toLocalDateString(): Display the week, month, day, and year by region;
toLocalTimeString() : Hours, minutes and seconds;
toUTCString() : Displays the complete UTC date in a specific format, and the specific display varies depending on the browser.
4. RegExp type: regular expression;
var expression = /pattern/flags; 标志flags包括 : g : 全局; i : 不区分大小写; m : 多行模式; 例子 : var pattern = /at/g 全局找at var pattern = /[bc]at/i ; 匹配第一个bat或cat,不区分大小写。 无意符须转义; * RegExp实例方法:
The main method of RegExp is
-exec(): capture group, the parameter is a string, return an array, if there is no match, return null , returns two additional properties: index and input.
index: Position input: Captured string
-test(): Returns true if matched, commonly used to verify user input.
5. Function type
* 概念
A function is actually an object. Like other reference types, it has properties and methods. The function name is just a pointer to the object.
* 没有重载
When declaring the same function, the latter one will overwrite the previous one.
* 函数声明和函数表达式 function sum(){ } // 函数声明 var sum = function(){ } // 函数表达式函数声明可以变量提升,任何时候任何位置都可以调用。 * 作为值的函数
Pass the function as a parameter to another function. To access the function without executing the function, you must remove the pair of parentheses after the function name
// Returning a function from one function to another
// Sort according to an object array
function compare(pro){
return function(obj1,obj2){ var val1 = obj1[pro]; var val2 = obj2[pro]; if(val1 > val2){ return 1; }else if(val1 <p> contains two special objects: this and argument<br>-argument: contains all the parameters passed in, and contains The callee attribute is a pointer and only wants to own the function of this object. <br>// Recursive algorithm to calculate factorial <br>function fac(num){</p><pre class="brush:php;toolbar:false">if(num <p>Function is also an object, so it has properties and methods; </p><pre class="brush:php;toolbar:false">* -属性 : length 和 prototype
-length indicates that you want to receive named parameters number.
-Prototype is the real place where all forces and methods are saved, such as tostring(), valueOf, etc., which can also be used for inheritance, which is extremely important.
The prototype attribute is not enumerable, so for-in cannot be used.
* -方法 : 非继承而来的方法有两个: apply() 和 call().
The function is to call the function in a specific scope. It actually sets the value of this object in the function body. The two methods have the same function, but the difference is the way they receive parameters. call(): List the parameters one by one
apply(): The parameter is an array. The function of both is not only to pass parameters, but also to expand the scope of the function. Pass in inaccessible places.
bind(): method, creates a function instance, binds to this value, global scope.
6、基本包装类型 (也是对象)
为方便操作基本类型值,提供3个特殊引用类型:Boolean,Number和String.
每读取值后台都会创建对应的基本包装类型的对象,才可以用方法操作数据。
引用类型与基本包装类型主要区别为对象生存期,用new创建引用累心实例一直保存在内存中,自动创建基本包装类型存在执行瞬间,然后立即销毁,所以不能给基本类型添加属性和方法。
* Boolean 类型
typeof 基本类型 // ‘boolean’typeof 引用类型 // 'object'建议永不要使用Boolean对象
* Number 类型
toFixed()方法 : 按照指定方法返回数值的字符串表示。
var num = 10;num.tpFixed(2) // '10.00'
toExpoential() : 幂
* String 类型
属性:length : 表示含多少个字符方法:
1)字符方法:charAt()和charCodeAt():查找某个字符在字符串中的位置。 charCodeAt():查找字符编码
2)字符串操作方法:拼接用+号
-三个给予字符串创建新串:
slice():切片 // 第一个参数为起始位置,第二个参数为结束位置substr():子函数 // 第一个参数为起始位置,第二个参数为截取的个数substring():子串 // 第一个参数为起始位置,第二个参数为结束位置
以上方法都不会影响原始字符串
3)字符串位置方法:
indexof:从字符串中查找字符串,返回位置,查不到则返回-1.
indexOf:从头查找lastIndexOf:从尾查找 返回第一次出现的位置
4)trim:创建字符串副本,删除前后所有空格,原始字符串不变。
5)字符串大小写转变:
toLocalUpperCase():转大写,针对特定地区
toLocalLowerCase():转小写,针对特定地区
toUpperCase():转大写
toLowerCase():转小写
6)字符串模式匹配方法:
match():与RegExp的exec方法相同,参数为正则表达式活RegExp对象。
search():从尾开始查找,参数与match相同,找不到返回-1
replace():替换
split():切片,基于指定分隔符字符串为多个子字符串。第二个参数可选,返回几个数组。
7、单体内置对象
已自行实例化,可直接使用,Global和Math
* -Global对象,全局对象:所有全局作用域中属性和方法都是它的。
--url编码
--eval():解析字符串代码并执行
* -Math对象
--Math.ceil():向上取整。
--Math.floor():向下取整。
--Math.round():四舍五入。
* random()方法:随机数,返回0~1之间随机数。
本篇文章到这里就已经全部结束了,更多其他精彩内容可以关注PHP中文网的JavaScript视频教程栏目!
The above is the detailed content of Detailed introduction to javaScript reference types (with examples). For more information, please follow other related articles on the PHP Chinese website!
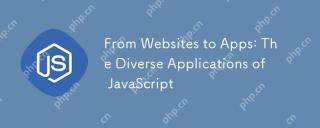
JavaScript is widely used in websites, mobile applications, desktop applications and server-side programming. 1) In website development, JavaScript operates DOM together with HTML and CSS to achieve dynamic effects and supports frameworks such as jQuery and React. 2) Through ReactNative and Ionic, JavaScript is used to develop cross-platform mobile applications. 3) The Electron framework enables JavaScript to build desktop applications. 4) Node.js allows JavaScript to run on the server side and supports high concurrent requests.
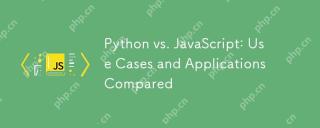
Python is more suitable for data science and automation, while JavaScript is more suitable for front-end and full-stack development. 1. Python performs well in data science and machine learning, using libraries such as NumPy and Pandas for data processing and modeling. 2. Python is concise and efficient in automation and scripting. 3. JavaScript is indispensable in front-end development and is used to build dynamic web pages and single-page applications. 4. JavaScript plays a role in back-end development through Node.js and supports full-stack development.
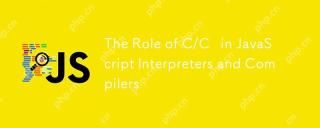
C and C play a vital role in the JavaScript engine, mainly used to implement interpreters and JIT compilers. 1) C is used to parse JavaScript source code and generate an abstract syntax tree. 2) C is responsible for generating and executing bytecode. 3) C implements the JIT compiler, optimizes and compiles hot-spot code at runtime, and significantly improves the execution efficiency of JavaScript.
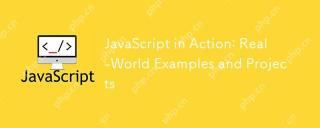
JavaScript's application in the real world includes front-end and back-end development. 1) Display front-end applications by building a TODO list application, involving DOM operations and event processing. 2) Build RESTfulAPI through Node.js and Express to demonstrate back-end applications.
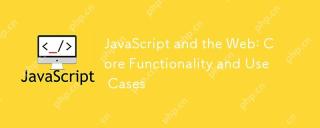
The main uses of JavaScript in web development include client interaction, form verification and asynchronous communication. 1) Dynamic content update and user interaction through DOM operations; 2) Client verification is carried out before the user submits data to improve the user experience; 3) Refreshless communication with the server is achieved through AJAX technology.
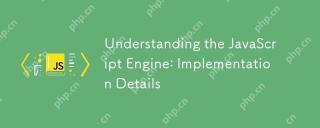
Understanding how JavaScript engine works internally is important to developers because it helps write more efficient code and understand performance bottlenecks and optimization strategies. 1) The engine's workflow includes three stages: parsing, compiling and execution; 2) During the execution process, the engine will perform dynamic optimization, such as inline cache and hidden classes; 3) Best practices include avoiding global variables, optimizing loops, using const and lets, and avoiding excessive use of closures.

Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
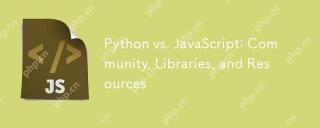
Python and JavaScript have their own advantages and disadvantages in terms of community, libraries and resources. 1) The Python community is friendly and suitable for beginners, but the front-end development resources are not as rich as JavaScript. 2) Python is powerful in data science and machine learning libraries, while JavaScript is better in front-end development libraries and frameworks. 3) Both have rich learning resources, but Python is suitable for starting with official documents, while JavaScript is better with MDNWebDocs. The choice should be based on project needs and personal interests.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
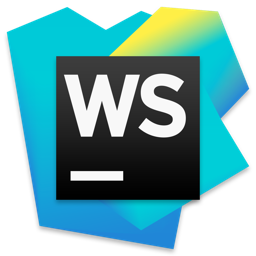
WebStorm Mac version
Useful JavaScript development tools

Atom editor mac version download
The most popular open source editor
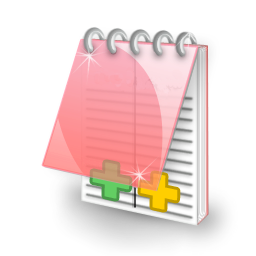
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software