


Introduction to Error handling and problem location under PHP5 (code example)
This article brings you an introduction to Error handling and problem location under PHP5 (code examples). It has certain reference value. Friends in need can refer to it. I hope it will be helpful to you. .
Let’s talk about the problem locating method when PHP encounters an E_ERROR level fatal runtime error. For example, Fatal error: Allowed size memory of
memory overflow. When this kind of error occurs, the program will exit directly. An error log will be recorded in PHP's error log indicating the specific file and number of lines of code where the error was reported, and no other information will be lost. If it is PHP7, you can catch errors like exceptions, but not with PHP5.
The generally thought of method is to look at the specific code of the error report. If the error file is CommonReturn.class.php, it will look like the following.
<?php /** * 公共返回封装 * Class CommonReturn */ class CommonReturn { /** * 打包函数 * @param $params * @param int $status * * @return mixed */ static public function packData($params, $status = 0) { $res['status'] = $status; $res['data'] = json_encode($params); return $res; } }
The json_encode line reported an error, and then you checked the packData method. There are many project classes that call it. How to locate the problem?
Scene Reproduction
Okay, first let’s reproduce the scene. If the actually called program bug.php is as follows
<?php require_once './CommonReturn.class.php'; $res = ini_set('memory_limit', '1m'); $res = []; $char = str_repeat('x', 999); for ($i = 0; $i < 900 ; $i++) { $res[] = $char; } $get_pack = CommonReturn::packData($res); // something else
When running bug.php, the PHP error log will record
[08-Jan-2019 11:22:52 Asia/Shanghai] PHP Fatal error: Allowed memory size of 1048576 bytes exhausted (tried to allocate 525177 bytes) in /CommonReturn.class.php on line 20
that the reproduction was successful. The error log only explains the file and line of code where the error was reported. , it is impossible to know the context stack information of the program, and does not know which piece of business logic is called, so it is impossible to locate and fix the error. How to troubleshoot if it occurs occasionally and there is no feedback from the front-end business.
Solution ideas
1. Some people thought of modifying memory_limit to increase memory allocation, but this method treats the symptoms but not the root cause. When doing development, you must find the root cause of the problem.
2. Turn on core dump. If the code file is generated, it can be debugged. However, it is found that the code will only be generated when the process exits abnormally. Errors at the E_ERROR level may not necessarily generate code files. The possibility of memory overflow is handled by PHP internally.
3. Use register_shutdown_function to register a callback function when PHP terminates, and then call error_get_last. If the last error that occurred is obtained, use debug_print_backtrace to obtain the stack information of the program. Let's try it.
Modify the CommonReturn.class.php file as follows
<?php /** * 公共返回封装 * Class CommonReturn */ class CommonReturn { /** * 打包函数 * @param $params * @param int $status * * @return mixed */ static public function packData($params, $status = 0) { register_shutdown_function(['CommonReturn', 'handleFatal']); $res['status'] = $status; $res['data'] = json_encode($params); return $res; } /** * 错误处理 */ static protected function handleFatal() { $err = error_get_last(); if ($err['type']) { ob_start(); debug_print_backtrace(DEBUG_BACKTRACE_IGNORE_ARGS, 5); $trace = ob_get_clean(); $log_cont = 'time=%s' . PHP_EOL . 'error_get_last:%s' . PHP_EOL . 'trace:%s' . PHP_EOL; @file_put_contents('/tmp/debug_' . __FUNCTION__ . '.log', sprintf($log_cont, date('Y-m-d H:i:s'), var_export($err, 1), $trace), FILE_APPEND); } } }
Run bug.php again, the log is as follows.
error_get_last:array ( 'type' => 1, 'message' => 'Allowed memory size of 1048576 bytes exhausted (tried to allocate 525177 bytes)', 'file' => '/CommonReturn.class.php', 'line' => 23, ) trace:#0 CommonReturn::handleFatal()
The traceback information has no source, which is embarrassing. I guess because the backtrace information is stored in memory and will be cleared when a fatal error occurs. There is no other way, try passing the backtrace in from the outside. Modify CommonReturn.class.php again.
<?php /** * 公共返回封装 * Class CommonReturn */ class CommonReturn { /** * 打包函数 * @param $params * @param int $status * * @return mixed */ static public function packData($params, $status = 0) { ob_start(); debug_print_backtrace(DEBUG_BACKTRACE_IGNORE_ARGS, 5); $trace = ob_get_clean(); register_shutdown_function(['CommonReturn', 'handleFatal'], $trace); $res['status'] = $status; $res['data'] = json_encode($params); return $res; } /** * 错误处理 * @param $trace */ static protected function handleFatal($trace) { $err = error_get_last(); if ($err['type']) { $log_cont = 'time=%s' . PHP_EOL . 'error_get_last:%s' . PHP_EOL . 'trace:%s' . PHP_EOL; @file_put_contents('/tmp/debug_' . __FUNCTION__ . '.log', sprintf($log_cont, date('Y-m-d H:i:s'), var_export($err, 1), $trace), FILE_APPEND); } } }
Run bug.php
again, the log is as follows.
error_get_last:array ( 'type' => 1, 'message' => 'Allowed memory size of 1048576 bytes exhausted (tried to allocate 525177 bytes)', 'file' => '/CommonReturn.class.php', 'line' => 26, ) trace:#0 CommonReturn::packData() called at [/bug.php:13]
Successfully located the source of the call, which is on line 13 of bug.php. Publish the final CommonReturn.class.php to the production environment, and just look at the log when an error occurs again. But in this case, all programs that call packData will execute the trace function, which will definitely affect performance.
Summary
You need to pay attention to the register_shutdown_function function used. You can register multiple different callbacks, but if a certain callback function If you exit, any callback functions registered later will not be executed.
debug_print_backtrace This function to obtain backtrace information first contains request parameters, and the second is the number of backtrace record levels. We do not return request parameters here, which can save some memory, and if If the request parameters are huge, calling this function may cause memory overflow.
The best way is to upgrade PHP7, which can catch errors like exceptions.
The above is the detailed content of Introduction to Error handling and problem location under PHP5 (code example). For more information, please follow other related articles on the PHP Chinese website!

ThesecrettokeepingaPHP-poweredwebsiterunningsmoothlyunderheavyloadinvolvesseveralkeystrategies:1)ImplementopcodecachingwithOPcachetoreducescriptexecutiontime,2)UsedatabasequerycachingwithRedistolessendatabaseload,3)LeverageCDNslikeCloudflareforservin
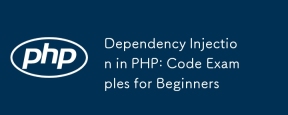
You should care about DependencyInjection(DI) because it makes your code clearer and easier to maintain. 1) DI makes it more modular by decoupling classes, 2) improves the convenience of testing and code flexibility, 3) Use DI containers to manage complex dependencies, but pay attention to performance impact and circular dependencies, 4) The best practice is to rely on abstract interfaces to achieve loose coupling.
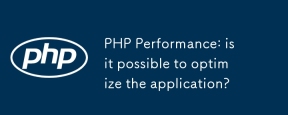
Yes,optimizingaPHPapplicationispossibleandessential.1)ImplementcachingusingAPCutoreducedatabaseload.2)Optimizedatabaseswithindexing,efficientqueries,andconnectionpooling.3)Enhancecodewithbuilt-infunctions,avoidingglobalvariables,andusingopcodecaching
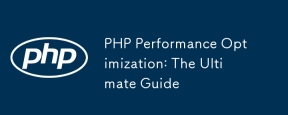
ThekeystrategiestosignificantlyboostPHPapplicationperformanceare:1)UseopcodecachinglikeOPcachetoreduceexecutiontime,2)Optimizedatabaseinteractionswithpreparedstatementsandproperindexing,3)ConfigurewebserverslikeNginxwithPHP-FPMforbetterperformance,4)
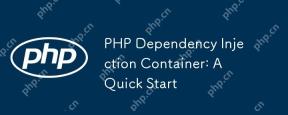
APHPDependencyInjectionContainerisatoolthatmanagesclassdependencies,enhancingcodemodularity,testability,andmaintainability.Itactsasacentralhubforcreatingandinjectingdependencies,thusreducingtightcouplingandeasingunittesting.
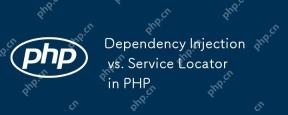
Select DependencyInjection (DI) for large applications, ServiceLocator is suitable for small projects or prototypes. 1) DI improves the testability and modularity of the code through constructor injection. 2) ServiceLocator obtains services through center registration, which is convenient but may lead to an increase in code coupling.
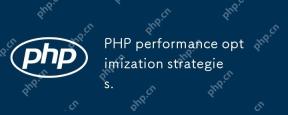
PHPapplicationscanbeoptimizedforspeedandefficiencyby:1)enablingopcacheinphp.ini,2)usingpreparedstatementswithPDOfordatabasequeries,3)replacingloopswitharray_filterandarray_mapfordataprocessing,4)configuringNginxasareverseproxy,5)implementingcachingwi
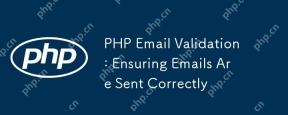
PHPemailvalidationinvolvesthreesteps:1)Formatvalidationusingregularexpressionstochecktheemailformat;2)DNSvalidationtoensurethedomainhasavalidMXrecord;3)SMTPvalidation,themostthoroughmethod,whichchecksifthemailboxexistsbyconnectingtotheSMTPserver.Impl


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
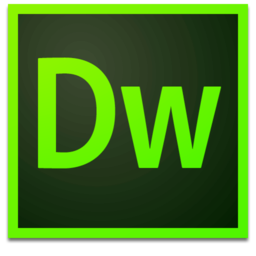
Dreamweaver Mac version
Visual web development tools

Zend Studio 13.0.1
Powerful PHP integrated development environment
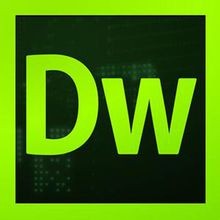
Dreamweaver CS6
Visual web development tools
